IDEA
For my midterm project, I decided to create a game out of the options from which we could choose any to create a project. A game that would use all the concepts learned in class so far. I got my inspiration from a game on the apple app store called “Hit the Island”. I always wondered how the game was created and how the developers got it to be interactive with the dynamic island on the iPhone 14 pro series. After taking this class, I have gained enough knowledge to know that the game doesn’t actually interact with the dynamic island but has a bar-shaped object behind the island with which the balls in the game interact.
ORIGINAL GAME
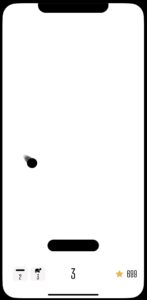
The original game below was developed by Funn Media, LLLC.
UI DESIGN
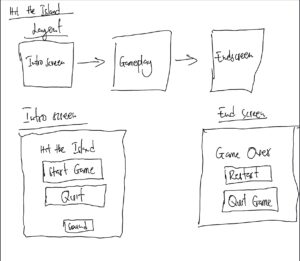
This is the basic form which i want my game to take. On a surface level, the game basically consists of a paddle, a ball and another paddle on top of the screen which i call the island. In the game, the paddle is controlled using the left and right arrow keys. Every time the ball hits the island, the user gains a point. The game ends when the balls fall off the screen. The game has three different pages. The intro page, the game page and the end page. In the intro page, the user has the option to start the game or quit the game. In the end page or game-over page, which shows after the user has lost the game, the user has the option to restart the game or quit as well.
PROGRESS SO FAR
So far, I have the basic game page set up with the balls and the user-paddle. I also have placeholders( which are basic color backgrounds ) set in place for the intro page and the end page which i am yet to design in either photoshop or illustrator.
CHALLENGES SO FAR AND INTENDED UPDATE
I haven’t encountered any major challenges so far. This is my progress as at now and in the final version of my game, i intend to to have two paddles, the moveable one which i have now and a static one (the island) at the top of the screen just as the original game. Every time the ball hits the paddles, there will be a sound feedback to improve the overall experience of the game. There will also be a background sound and an option for the user to mute the sound in the game or to keep it playing.