Concept:
For my final project, I decided to make a running experience game with a timer. I had this idea so that people can exercise from home. A person puts the accelerometer on his/her leg clicks the button to start the game and presses it again to end it. I wanted to go with a simple design and a specific purpose for this project.
Highlight and Process:
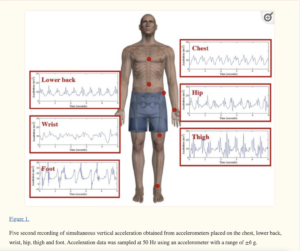
This project did not go as smoothly as I expected it to be. I had to try multiple sensors to see which one fits my concept best. I tried a potentiometer, an XYZ-axis accelerometer, and a Gyroscope Accelerometer. I had to research a lot about them and see what can be done.
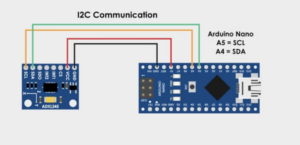
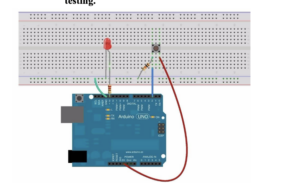
I decided to go on and use the ADXL335 Accelerometer. I read the documentation I found online for it. I have to say that figuring out the circuit was not the easiest thing either. I made the circuit maybe 5 times to get it right. I also decided to use the Arcade button with LED. I burned myself while soldering but I learned to be careful.
After figuring out the circuit and testing it with build-in examples, I moved on to designing the box and putting the accelerometer in a small container I made of two bottle caps. Cutting the box using the laser cutter was not smooth either as the laser cutter stopped working in the middle of cutting my box, and I had to redo it the next day. I made sure everything was in place and worked fine, then I moved to the code.
I started with the code, and I found an example of how to convert the data we get from the accelerometer to acceleration. This was my starting point. I refined the code a little bit to serve the purpose of my project. Then I added different elements like the button and the serial communication. However, when I did that the code stopped working. It took me two days to figure out the problem as highlighted in the pdf file attached.
// variables for the sensor x, y, z axis const int xInput = A0; const int yInput = A1; const int zInput = A2; // initialize minimum and maximum Raw Ranges for each axis int RawMin = 0; int RawMax = 1023; // Take multiple samples to reduce noise we take the average of these const int sampleSize = 10; // for the arcade button state and its led // pinmodes and pin # int button = 8; int LED = 4; //state of the button int buttonState = 0; int lastButtonState = 0; int LED_state = 0; // Take samples and return the average from the accelemeter -- // readAxis function takes the 10 samples from analog to digital converter of // an Arduino and delivers the average value. int ReadAxis(int axisPin) { long reading = 0; analogRead(axisPin); delay(1); for (int i = 0; i < sampleSize; i++) { reading += analogRead(axisPin); } return reading / sampleSize; } void setup() { analogReference(EXTERNAL); Serial.begin(9600); // We'll use the builtin LED as a status output. // We can't use the serial monitor since the serial connection is // used to communicate to p5js and only one application on the computer // can use a serial port at once. pinMode(LED_BUILTIN, OUTPUT); pinMode(button, INPUT_PULLUP); pinMode(LED, OUTPUT); // start the handshake // while (Serial.available() <= 0) { // digitalWrite(LED_BUILTIN, HIGH); // on/blink while waiting for serial data // Serial.println("0,0"); // send a starting message // delay(300); // wait 1/3 second // digitalWrite(LED_BUILTIN, LOW); // delay(50); // } } void loop() { //Read raw values int xRaw = ReadAxis(xInput); int yRaw = ReadAxis(yInput); int zRaw = ReadAxis(zInput); // Convert raw values to 'milli-Gs" long xScaled = map(xRaw, RawMin, RawMax, -3000, 3000); long yScaled = map(yRaw, RawMin, RawMax, -3000, 3000); long zScaled = map(zRaw, RawMin, RawMax, -3000, 3000); // re-scale to fractional Gs 1/1000 0f g float xAccel = xScaled / 1000.0; float yAccel = yScaled / 1000.0; float zAccel = zScaled / 1000.0; // wait for data from p5 before doing something // while (Serial.available()) { digitalWrite(LED_BUILTIN, HIGH); // led on while receiving data buttonState = digitalRead(button); if (button != lastButtonState && lastButtonState == 1) { // delay helps in false pushes delay(50); if (buttonState == LOW) { if (digitalRead(LED) == 1) { LED_state = 0; // turn LED off digitalWrite(LED, LED_state); } else { LED_state = 1; digitalWrite(LED, LED_state); // Turn on LED } } } lastButtonState = buttonState; // if (Serial.read() == '\n') { // send to p5js // Serial.print(xRaw); // Serial.print(", "); // Serial.print(yRaw); // Serial.print(", "); // Serial.print(zRaw); // Serial.print(" :: "); Serial.print(xAccel); Serial.print(','); Serial.print(yAccel); Serial.print(','); Serial.print(zAccel); Serial.print(','); Serial.println(LED_state); delay(200); // } digitalWrite(LED_BUILTIN, LOW); } // }
When I figured out the Arduino side. I began designing the interface of the project. I had to create different levels so that the users could switch from the start of the experience to the end and back again. I decided to use the LED state to do that. Meaning that when the LED state is 1 the experience starts and when it Is zero it ends. In the States, I added what should happen. In the second state when the LED is 1, the timer starts and it starts looking through the sprite sheet at the rate of the Z-value multiplied by the time, which is linear speed. Unfortunately, I had to assume the physics to make it work and I am not sure how accurate it is. I have noticed that there is a little delay but I can’t figure out why. I think it is because P5js is a little slow.
P5js link ->https://editor.p5js.org/shn202/full/gOx6xjm6X
Reflection:
There is a lot more that can be done to this project. I will admit that I learned a lot implementing it and even though it is not as I expected it to be, I think with all the limitations and problems I faced it turned out to be good. In the future, I would like to make it more interactive with more preferences to it. I feel time was a challenge in this project and that if we had more time it would have turned out better.
https://www.circuits-diy.com/how-adxl335-accelerometer-interface-with-arduino-uno/
https://robu.in/accelerometer-sensor-arduino/
https://docs.arduino.cc/built-in-examples/sensors/ADXL3xx
https://www.youtube.com/watch?v=wTfSfhjhAU0
https://docs.arduino.cc/software/ide-v1/tutorials/installing-libraries
https://forum.pololu.com/t/l3gd20-gyro-unable-to-get-sample-arduino-code-working/5146
https://www.youtube.com/watch?v=KMhbV1p3MWk&t=333s
https://www.youtube.com/watch?v=-QY0jN3gtgs&t=301s
https://en.wikipedia.org/wiki/Potentiometer
https://forum.arduino.cc/t/determine-speed-from-hollow-shaft-potentiometer/143502