For this week project I took inspiration from my one of my previous weekly assignments where I made a device where a user could record a Morse code message and could play it back.
One issue with that project is that a user must know Morse code first. I personally don’t know Morse code but I find the idea to be pretty cool.
For my assignment this week I made a setup where you could interact with processing via the buttons on the breadboard to input a dashes and dot and you could press enter to check if your input was correct.
The user would press the blue button to input a dot and the yellow button to input a dash. The corresponding would be displayed on the processing window. Once the user is satisfied with the input they can press the red button to check if the input is correct. If the input is correct a green light would flash on the breadboard, if not a red light would flash. Once the enter button is pressed a new letter is displayed and the input is cleared.
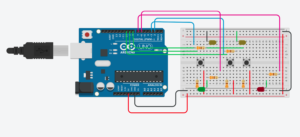
import processing.serial.*; Serial port; int[][] morseCode = { {1, 2}, //a {2, 1, 1, 1}, //b {2, 1, 2, 1}, //c {2, 1, 1}, //d {1}, //e {1, 1, 2, 1}, //f {2, 2, 1}, //g {1, 1, 1, 1}, //h {1, 1}, //i {1, 2, 2, 2}, //j {2, 1, 2}, //k {1, 2, 1, 1}, //l {2, 2}, //m {2, 1}, //n {2, 2, 2}, //o {1, 2, 2, 1}, //p {2, 2, 1, 2}, //q {1, 2, 1}, //r {1, 1, 1}, //s {2}, //t {1, 1, 2}, //u {1, 1, 1, 2}, //v {1, 2, 2}, //w {2, 1, 1, 2}, //x {2, 1, 2, 2}, //y {2, 2, 1, 1} //z }; int currentAlphabet; ArrayList<Integer> input = new ArrayList<Integer>(); int recInput = -1; String inputStr = ""; /* the ascii for uppercase alphahets range from 65[A] to 90[Z] */ void setup() { size(600, 600); //println(morseCode.length); generateNewLetter(); //printArray(Serial.list()); String portname = Serial.list()[0]; port = new Serial(this, portname, 9600); println(portname); } void draw() { background(167); displayLetter(); displayInput(); } void generateNewLetter() { currentAlphabet = floor(random(26)); } void displayLetter() { // (char)(currentAlphabet+65) -> this will give the letter based on current alphabet index pushStyle(); fill(0); textAlign(CENTER); textSize(200); text((char)(currentAlphabet+65), width/2, height/2); popStyle(); } void displayInput() { pushStyle(); fill(0); textAlign(CENTER); textSize(72); text(inputStr, width/2, height/2+200); popStyle(); } void serialEvent(Serial port) { recInput = port.read(); if (recInput == 1) { input.add(recInput); inputStr+=" *"; } else if (recInput==2) { input.add(recInput); inputStr+=" -"; } else if (recInput == 3) { if (checkCorrect()) { port.write(101); } else if (!checkCorrect()) { port.write(100); } inputStr = ""; input.clear(); generateNewLetter(); println("enter was pressed"); } } boolean checkCorrect() { boolean correct = true; if (morseCode[currentAlphabet].length == input.size()) { for (int i=0; i<morseCode[currentAlphabet].length; i++) { if (morseCode[currentAlphabet][i] != input.get(i)) { correct = false; break; } } } else { correct = false; } println(correct); return correct; }
int led1 = 3; int led2 = 2; int enter = 8; int dot = 9; int dash = 10; int buttonDelay = 400; int wrong = 7; int correct = 6; void setup() { // put your setup code here, to run once: Serial.begin(9600); pinMode(led1,OUTPUT); pinMode(led2,OUTPUT); pinMode(enter,INPUT); pinMode(dot,INPUT); pinMode(dash,INPUT); pinMode(wrong,OUTPUT); pinMode(correct,OUTPUT); } void loop() { // put your main code here, to run repeatedly: if (digitalRead(dot)==1){ // send a 1 to processing Serial.write(1); digitalWrite(led1,HIGH); delay(buttonDelay); digitalWrite(led1,LOW); } else if(digitalRead(dash)==1){ // send a 2 to processing Serial.write(2); digitalWrite(led1,HIGH); digitalWrite(led2,HIGH); delay(buttonDelay); digitalWrite(led1,LOW); digitalWrite(led2,LOW); } else if(digitalRead(enter)==1){ Serial.write(3); delay(buttonDelay); } if (Serial.available()>0){ int data = Serial.read(); if (data == 100){ digitalWrite(wrong,HIGH); delay(100); digitalWrite(wrong,LOW); } else if(data == 101){ digitalWrite(correct,HIGH); delay(100); digitalWrite(correct,LOW); } } } /* * 1 for dot * 2 for dash * 3 for enter */