Concept
For this assignment, I wanted to make an art that is hectic, colorful, and mesmerizing which is what comes to my mind when I think of media art. First, I read through the old computer arts magazine for inspiration and one piece of art got stuck in my head which was:
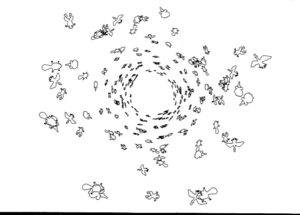
I really liked this piece because the shapes looked like it was spiraling towards the center which feels infinitely faraway. Thus, I decided to implement some spiraling component in my art piece with motion and color. At first, I wanted to have a bezier curve squiggling towards the center from all directions, making a huge squiggling circle. But manipulating with multiple bezier curves turned out to be very difficult so ended up with two bezier curves moving across the canvas, squiggling using the random function which I decided to keep. The shape I wanted to spiral was a circle simply because the shapes were going to orbit around the center in a circle. The color of the background and the circle shapes were randomly set using a random function and were randomly changed as well by calling the random color function which added/subtracted each RGB value randomly.
Code
* IF YOU CLICK THE CENTER CIRCLE, YOU CAN SEE THE PAUSE MENU
in the pause menu, you can control the toggleLeft and toggleRight variable which determines the distance between each circle’s orbit and has a default value of 10. You can also control the sin factor which is the constant multiplied to the sin component that determines the orbit and has a default value of 1/2.
The code has several sections. First, there is the initial section of establishing the global variables and setup function that sets up a random color for the background. Second, the draw function has a two conditional branches based on the boolean variable onOff where if this is true the drawTrue function will be called and thus drawn, and if it is false, drawPaused function will be called and the art will be paused.
function draw() {
if(onOff > 0)
drawTrue();
else{
drawPaused();
}
button = circle(300,300,10);
}
onOff variable can be switched true and false by clicking the button circle in the center. Third, the functions mouseClicked and drawPaused are functions that deal with pausing gimmick and changing the sin factor variable and toggle variables. Lastly, the drawTrue function which is the code that runs the art has also two compartments: one that deals with the squiggling bezier curves and one that draws the circles. The bezier curve gimmick really caused me a headache because initially I didn’t understand how it worked so after trial and error, I made a two separate bezier drawing functions: one going from top left corner to lower right and one vice versa. Both of these functions take a direction parameter and an array of parameter which have four elements for the four parameters of bezier shape which are randomly added/subtracted to make the bezier curve squiggle. The other component that deals with the orbiting circles use the for loop to draw 200 circles orbiting around using the sin() cos() function to constantly move the circle around the orbit. To diversify the speed between each 0rbit, I created an array of angles where each element is the speed of the orbit.
for(let i = 0; i < 100; i++){
let x1 = 0;
let y1 = 0;
strokeWeight(1);
if(i % 2 == 0){
x1 = 300 + toggleLeft * i * sin(angle[i]);
y1 = 300 + toggleLeft * i * cos(angle[i]);
}
else{
x1 = 300 + toggleRight * i * cos(angle[i]);
y1 = 300 + toggleRight * i * factor * sin(angle[i]);
}
randomColor(colorsCircle);
fill(colorsCircle[0], colorsCircle[1], colorsCircle[2])
circle(x1, y1, i * 1);
circle(y1, x1, i * 1);
}
for(let j = 0; j < 100; j++){
angle[j] += 0.0314 + (j * 0.001);
}
And this is my full code:
let onOff = 1;
let xMotion = 0;
let yMotion = 0;
let colors = [0,0,0];
let colorsCircle = [0,0,0];
let paramsA = [0,0,0,0];
let paramsB = [0,0,0,0];
let counter = 0;
let toggleLeft = 10;
let toggleRight = 10;
let factor = 1/2;
let angle = [];
function setup() {
createCanvas(600, 600);
for(let i = 0; i < 3; i++){
colors[i] = random(0,255);
}
for(let j = 0; j < 100; j++){
angle.push(j);
}
}
function draw() {
if(onOff > 0)
drawTrue();
else{
drawPaused();
}
button = circle(300,300,10);
}
function mouseClicked(){
if(dist(mouseX, mouseY, 300, 300) <= 10)
onOff *= -1;
else if(dist(mouseX, mouseY, 110,400) <= 20 && toggleLeft > 1){
toggleLeft--;
console.log(toggleLeft);
}
else if(dist(mouseX, mouseY, 160,400) <= 20 && toggleLeft < 10){
toggleLeft++;
console.log(toggleLeft);
}
else if(dist(mouseX, mouseY, 420,400) <= 20 && toggleRight > 1){
toggleRight--;
console.log(toggleRight);
}
else if(dist(mouseX, mouseY, 510,400) <= 20 && toggleRight < 10){
toggleRight++;
console.log(toggleRight);
}
else if(dist(mouseX, mouseY, 100,525) <= 25){
factor = 1/2;
}
else if(dist(mouseX, mouseY, 300,525) <= 25){
factor = 1;
}
else if(dist(mouseX, mouseY, 500,525) <= 25){
factor = 2;
}
}
function drawPaused(){
background(255);
textSize(100);
fill(0);
text('Paused', 150, 200);
triangle(100, 400, 120, 380, 120, 420);
triangle(170, 400, 150, 380, 150, 420);
triangle(430, 400, 450, 380, 450, 420);
triangle(500, 400, 480, 380, 480, 420);
fill(255);
rect(75, 500, 50,50);
rect(275, 500, 50,50);
rect(475, 500, 50,50);
textSize(25);
fill(0);
text('1/2', 80, 525);
text('1', 290, 525);
text('2', 480, 525);
text("Toggle Left", 75, 350);
text("Toggle Right", 400, 350);
text("Sin Factor", 250, 470);
}
function drawTrue(){
background(colors[0], colors[1], colors[2]);
fill(colors[0],colors[1],colors[2])
stroke(colors[0]);
strokeWeight(10);
if(xMotion < 610 && yMotion > -10){
xMotion ++;
yMotion --;
}
else{
xMotion = -10;
yMotion = 610;
paramsA = [0,0,0,0];
paramsB = [0,0,0,0];
}
if(counter == 10){
randomColor(colors);
counter = 0;
randomParam(paramsA);
randomParam(paramsB);
}
drawBezierX(xMotion, paramsA);
drawBezierY(yMotion, paramsB);
counter++;
for(let i = 0; i < 100; i++){
let x1 = 0;
let y1 = 0;
strokeWeight(1);
if(i % 2 == 0){
x1 = 300 + toggleLeft * i * sin(angle[i]);
y1 = 300 + toggleLeft * i * cos(angle[i]);
}
else{
x1 = 300 + toggleRight * i * cos(angle[i]);
y1 = 300 + toggleRight * i * factor * sin(angle[i]);
}
randomColor(colorsCircle);
fill(colorsCircle[0], colorsCircle[1], colorsCircle[2])
circle(x1, y1, i * 1);
circle(y1, x1, i * 1);
}
for(let j = 0; j < 100; j++){
angle[j] += 0.0314 + (j * 0.001);
}
}
function randomColor(colorsArr){
for(let i = 0; i < 3; i++){
colorsArr[i] += random(-15,15);
}
}
function drawBezierX(dirX, param){
bezier(dirX + param[0], dirX + param[0], dirX + 10, dirX + 10 + param[1], dirX + 20, dirX + 20 + param[2], dirX + 30,dirX + 30 + param[3]);
}
function drawBezierY(dirY, param){
bezier(dirY + param[0], dirY + param[0], dirY + 10, dirY + 10 + param[1], dirY + 20, dirY + 20 + param[2], dirY + 30, dirY + 30 + param[3]);
}
function randomParam(param){
for(let i = 0; i < 4; i++){
param[i] += random(-30,30);
}
}
Reflection
Like the last assignment, for this assignment, I didn’t have a concrete plan on what I wanted to create through p5js but rather I did a lot of exploring, meddling, and experimenting with the code and stumbling upon many gimmicks that I didn’t intend but looked cool. After two practices, I found myself getting more and more used to this language and how to create and use functions and the draw function to achieve what I want. I also learned and got used to the interactive part of the p5js with mouseClicked function and how to have a panel with set of buttons on the canvas using this function.