Our idea for the musical instrument was to use sounds that the servo motor created rather than incorporating the piezo buzzer. So, we decided to try and recreate a gumball machine and the sound it makes when the gumballs fall out of the machine.
The Physical Design Process
We built the structure using a box, water bottle and cardboard. We then prepared the servo motors and prepared a cardboard piece to act as a ball stopper to control the amount of balls that fall through the hole.
We attached the servo motor and started testing out the servo motor with balls made from different materials to see what sound they made. We also decided to create two ramps for the balls to slide on to make more sound. As for the materials, we used pipe-cleaners, paper, and marbles. We found that the marbles made the best sound and rolled nicely down the ramp. The paper balls made a decent sound, whereas the pipe-cleaners were almost silent when they fell.
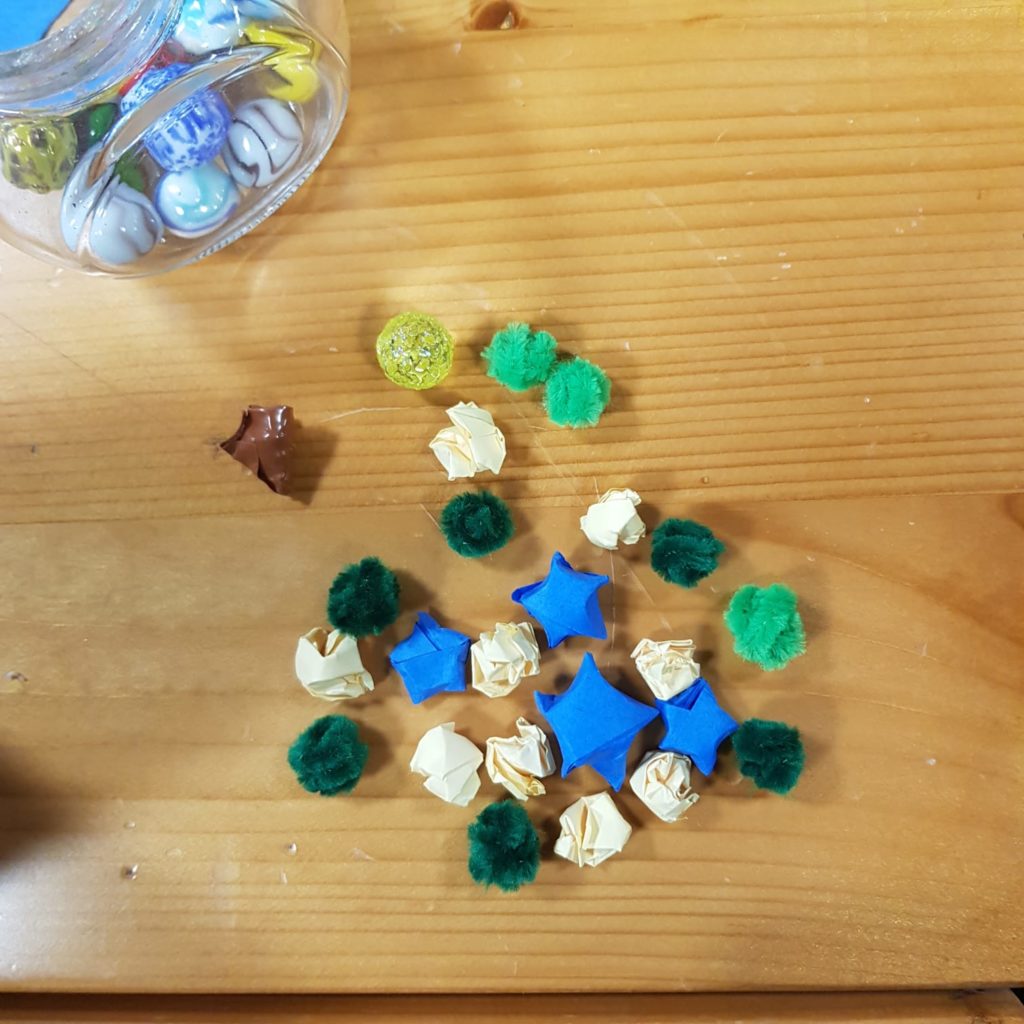
Here is the final version of the physical components:
And here is the schematic of our circuit:
Some things that didn’t work out as expected…
Our initial plan was to have the balls fall down one by one, but that didn’t work as expected. The balls either fell together when the ball stopper opened, or they would get stuck even when the stopper opened. When they do fall down, most of the time they wouldn’t roll down the ramps as intended. Usually, the balls would fall to the side, fall straight down, or when it does fall on the slide it wouldn’t roll all the way down and it would get stuck on the ramp. In this case, the marbles worked best since they were smooth spheres, but the marbles also had the problem with falling to the side. Additionally, at first we wanted the stopper to open and close continuously after one button press, but currently it just does the cycle once and you have to keep pressing the button for the continuous opening and closing.
The Coding Process
For implementing the sensors, we decided to use a push button and potentiometer. We used the button to control the servo motor, which either blocks or unblocks the hole where the balls fall out of. When it is open the balls fall out, and when it is closed the balls are stopped. To do this we used 2 if statements that checked if the button is clicked once, in which case the lever is opened, and if the button is clicked again then the lever is closed again.
if(clicks%2 == 0) { pos = 0; } else{ if (pos <= 70) { pos += servoSpeed; } } myServo.write(pos); delay(100);
Another variation of code was to have one press do both the opening and closing of the ball stopper. This was the code we used:
if (buttonState == 1){ for (pos = 0; pos <= 160; pos += servoSpeed) { myServo.write(pos); delay(15); } for (pos = 70; pos >= 0; pos -= servoSpeed) { myServo.write(pos); delay(100); } } else if (buttonState == 0) { myServo.write(70); delay(15); }
This is the link to the full code
We experimented with the delay, and the number for the position of the servo motor. If we made the delay longer, the servo motor would take longer to reach the position. Moreover, since the potentiometer controls the speed of the servo motor, when the potentiometer is at the maximum number, the servo motor moves faster, but since the delay is longer it doesn’t seem as fast. When the delays were all set to 15 and the potentiometer was at the maximum, the servo motor didn’t sweep the full amount (i.e. it didn’t complete the number of degrees in the for loop). While this was experimental, we ended up liking the sound it created and the movements of it.
Finally, here are a few different videos of our music machine!
This one is the first code, where one press opens and the other press closes it:
This one all the delay amounts are the same. We didn’t experiment with the potentiometer for this version, but the sound was nice:
This one has one long delay:
And this final one we tested with just marbles and shortened the long delay, which was in the previous video: