In class this week, we played around with the coordinate system and making shapes in Processing.
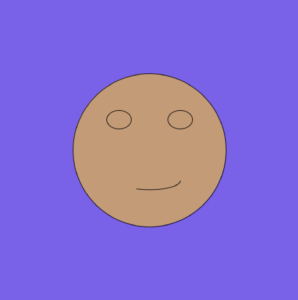
For our practice this week, I wanted to experiment with pixel art but the prospect of measuring and coloring every little rectangle filled me with dread. I remember watching a Coding Train video a while ago where Dan Shiffman used a .get() method to get the color from an image, so I decided to create the rectangles based on an image.
In terms of the pseudocode:
- Load an image (keep in same sketch folder)
- Define the canvas size.
- Pick a random x and y coordinate from the photo.
- Get the color of the photo at that x,y point.
- Create a rectangle at that x,y point with the fill of the color.
Here is my sketch:
Some of the design choices I thought about were the size of the rectangles (how pixellated should the portrait be?), the fill of the rectangles (should they be the same as the image or should there be different colors, alphas, brightnesses?), the framerate of the drawing (how fast should the rectangles be created?).
An interesting part of the code to me was the .get() method I learned about in the Coding Train video. This method essentially gets the color of the image at the x,y point. You can see the code below.
PImage me; //define size of the rectangle int pixelSize = 20; void setup() { size(600,715); me = loadImage("me.jpg"); //controls speed of drawing pixels frameRate(300); } void draw() { //create random x,y point within the sketch float x = random(width); float y = random(height); //learned about .get() from from Daniel Shiffman's Processing tutorial on pixels https://www.youtube.com/watch?v=NbX3RnlAyGU //get color from image at the x,y point color c = me.get(int(x), int(y)); fill(c); noStroke(); //draws rectangle at the x,y point with the color from the image rect(x, y, pixelSize, pixelSize); }
The whole sketch is available here.