INSPIRATION AND EXECUTION
My second week’s project was to create an artwork using loops. Although this sounded very exciting to me initially and I was all charged up to work for it, it took me a long time to think about what I actually wanted to make. Art is relative. There is nothing fixed nor definite. It is one’s imagination driven by creativity and inspiration. I could do anything; the topic was so open-ended that it was difficult to come up with an idea.
I read through some old computer art magazines and was very fascinated by the following picture from the book Computer_Graphics_and_Art_May1976 (p. 6):
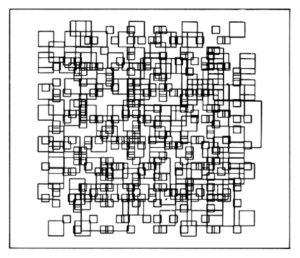
This picture, though simple, conveys the beauty of different blocks co-existing and still being peaceful in chaos. I wanted to create something similar in my art piece which could evoke such a feeling. Thus I started playing with squares on my canvas. To give an effect of chaos, I decided to have squares of different sizes to begin with and then make them move somehow, or rather change their size, to depict the different sizes as seen in the picture above. There was a little problem when I first coded this (see below). Since my width of the rectangle was dependent on frame count, it seemed like the rectangles were growing and shrinking faster and faster with each iteration which created a sense of panic or anxiety rather than calmness.
I solved this using the sine function which gave me a value between 1 and -1 and hence made it easier to reverse direction without affecting the speed (prof. Aaron Sherwood helped me with this). The consistency looked much better and pleasing. It also gave a smooth transition for changing direction. I then decided to add colors in the shades of blue and red to give a bright, happening, yet calming effect (not choosing pastel colors because the sense of chaos and happening still had to be maintained).
However, I still wasn’t fully satisfied with what I was seeing. The white spaces left a void that I wanted to cover. So, I started playing with background colors. In doing so, I accidentally removed the background altogether and what I saw, very well captured the emotion I was trying to bring about. The colors from different origins, each being startling and bright, having their own individuality, growing and shrinking in size, blended with each other so beautifully, producing a soothing effect in its dynamism. I then just tweaked the speed and colors a bit after that to make it look just right.
This is how my final art piece has turned out!
DIFFICULTIES
Besides facing difficulties controlling the speed of my moving boxes, I faced some difficulties initially when coding my boxes. It took me some time to understand how to use loops to code this project. What I was missing was the understanding that whichever loop I use inside my draw() function, would be an additional loop to the draw() loop which is an infinite loop. So, the way to go about it was thinking of it as ‘nested loops’ and exploiting the draw() loop to base the movement of my boxes on it. I first used nested for() loop to position my boxes on the canvas. Then, to move the boxes, I based the rectangle width on the frame count of the draw() loop. It took me sometime to understand that the transition should be based on the draw() loop count because I am not used to thinking of functions as loops and thus initially, I wasn’t using the draw() loop at all to code my assignment.
CODE
float h, w; //to rename height and width float rectW; //size of square -- changes throught the exxecution float rectW0; //initial square size int numBox; //number of boxes void setup() { background(150); size(600, 600); h = height; w = width; rectMode(CENTER); rectW = 100; rectW0 = rectW; numBox = int(w/rectW0); //noLoop(); } void draw() { noFill(); strokeWeight(0.3); int k=0; for (int i=0; i<numBox; i++) { for (int j=0; j<numBox; j++) { stroke(250-(i+j)*30, (0*i+j)*30, (i+j)*20); rect(rectW0/2+rectW0*i, rectW0/2+rectW0*j, rectW-k, rectW-k); k=k-1; } //close inner for } //close outer for rectW = sin(frameCount*.008)*width/2.2; }