Reference: I used a picture of myself for official documents to help me vizualize the self-portrait
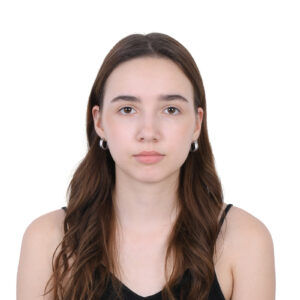
Reflection
This task was a really fun and creative way to dive into p5.js. At first, I was a bit nervous because the idea of creating a portrait entirely through code felt like a challenge. However, as I started working with the basic shapes, I realized how much fun it was to build my self-portrait step by step.
I began with simple shapes like ellipses for the head and hair, and a rectangle for the fringe. It was amazing how easily I could combine these basic elements to create the foundation of the portrait. Once I had the basic structure, I worked on adding details like the eyes, eyebrows, and nose.
My favorite part of the process was creating the blush effect on the cheeks. I used lines to make small strokes under the eyes, which gave the face a lot of character and expression. It felt like a small touch, but it made a big difference in bringing the portrait to life.
By the end of the task, I was surprised by how much I learned about coding and how creative you can be with simple drawing functions. One thing that bothered me is how time-consuming it can be to find the right “coordinates” on the canvas. It would have been helpful if I could hover over a specific spot where I’d want my object to start and see the coordinate number.
background(255, 182, 193);
ellipse(200, 225, 200, 290);
ellipse(200, 200, 150, 180);
fill(110, 70, 50); // Same color as hair
ellipse(170, 200, 30, 20); // Left eye white
ellipse(230, 200, 30, 20); // Right eye white
ellipse(170, 200, 10, 10); // Left iris
ellipse(230, 200, 10, 10); // Right iris
stroke(255, 182, 193); // Light pink color for blush
line(150, 220, 155, 225); // First line
line(160, 220, 165, 225); // Second line
line(170, 220, 175, 225); // Third line
line(220, 220, 225, 225); // First line
line(230, 220, 235, 225); // Second line
line(240, 220, 245, 225); // Third line
arc(170, 190, 40, 30, PI + QUARTER_PI, TWO_PI - QUARTER_PI); // Left eyebrow
arc(230, 190, 40, 30, PI + QUARTER_PI, TWO_PI - QUARTER_PI); // Right eyebrow
arc(200, 260, 50, 30, 0, PI);
ellipse(125, 220, 10, 10); // Left earring
ellipse(275, 220, 10, 10); // Right earring
arc(200, 340, 120, 80, PI, 0);
function setup() {
createCanvas(400, 400);
background(255, 182, 193);
// Hair
fill(110, 70, 50);
ellipse(200, 225, 200, 290);
// Head
fill(240, 209, 190);
noStroke();
ellipse(200, 200, 150, 180);
//Fringe
fill(110, 70, 50); // Same color as hair
noStroke();
rect(140, 110, 120, 30);
// Eyes
fill(255);
ellipse(170, 200, 30, 20); // Left eye white
ellipse(230, 200, 30, 20); // Right eye white
fill(150, 75, 0);
ellipse(170, 200, 10, 10); // Left iris
ellipse(230, 200, 10, 10); // Right iris
// Blush under left eye
stroke(255, 182, 193); // Light pink color for blush
strokeWeight(2);
line(150, 220, 155, 225); // First line
line(160, 220, 165, 225); // Second line
line(170, 220, 175, 225); // Third line
// Blush under right eye
line(220, 220, 225, 225); // First line
line(230, 220, 235, 225); // Second line
line(240, 220, 245, 225); // Third line
// Eyebrows
stroke(80, 50, 40);
strokeWeight(4);
noFill();
arc(170, 190, 40, 30, PI + QUARTER_PI, TWO_PI - QUARTER_PI); // Left eyebrow
arc(230, 190, 40, 30, PI + QUARTER_PI, TWO_PI - QUARTER_PI); // Right eyebrow
// Nose
noFill();
stroke(150, 100, 90);
strokeWeight(2);
beginShape();
vertex(200, 205);
vertex(195, 225);
vertex(200, 230);
endShape();
// Mouth
noStroke();
fill(180, 80, 90);
arc(200, 260, 50, 30, 0, PI);
// Earrings
fill(255, 255, 0);
ellipse(125, 220, 10, 10); // Left earring
ellipse(275, 220, 10, 10); // Right earring
// Shirt
fill(245, 245, 220);
rect(140, 340, 120, 80);
arc(200, 340, 120, 80, PI, 0);
// Neck
fill(240, 209, 190);
rect(175, 270, 50, 35);
}
function setup() {
createCanvas(400, 400);
background(255, 182, 193);
// Hair
fill(110, 70, 50);
ellipse(200, 225, 200, 290);
// Head
fill(240, 209, 190);
noStroke();
ellipse(200, 200, 150, 180);
//Fringe
fill(110, 70, 50); // Same color as hair
noStroke();
rect(140, 110, 120, 30);
// Eyes
fill(255);
ellipse(170, 200, 30, 20); // Left eye white
ellipse(230, 200, 30, 20); // Right eye white
fill(150, 75, 0);
ellipse(170, 200, 10, 10); // Left iris
ellipse(230, 200, 10, 10); // Right iris
// Blush under left eye
stroke(255, 182, 193); // Light pink color for blush
strokeWeight(2);
line(150, 220, 155, 225); // First line
line(160, 220, 165, 225); // Second line
line(170, 220, 175, 225); // Third line
// Blush under right eye
line(220, 220, 225, 225); // First line
line(230, 220, 235, 225); // Second line
line(240, 220, 245, 225); // Third line
// Eyebrows
stroke(80, 50, 40);
strokeWeight(4);
noFill();
arc(170, 190, 40, 30, PI + QUARTER_PI, TWO_PI - QUARTER_PI); // Left eyebrow
arc(230, 190, 40, 30, PI + QUARTER_PI, TWO_PI - QUARTER_PI); // Right eyebrow
// Nose
noFill();
stroke(150, 100, 90);
strokeWeight(2);
beginShape();
vertex(200, 205);
vertex(195, 225);
vertex(200, 230);
endShape();
// Mouth
noStroke();
fill(180, 80, 90);
arc(200, 260, 50, 30, 0, PI);
// Earrings
fill(255, 255, 0);
ellipse(125, 220, 10, 10); // Left earring
ellipse(275, 220, 10, 10); // Right earring
// Shirt
fill(245, 245, 220);
rect(140, 340, 120, 80);
arc(200, 340, 120, 80, PI, 0);
// Neck
fill(240, 209, 190);
rect(175, 270, 50, 35);
}