Concept:
In one of Daniel’s-“The Funny Guy” videos he created something like a buzzing bee effect and I really liked his reaction to it so I thought of making a game that uses the idea of a buzzing bee. For my midterm project, I created a game that mimics the behavior of a bee.
I decided to create a game with a bee theme to immerse the user into the game by making them swerve falling blocks. This is a simple game with 8 different levels that increase in difficulty as you progress. this is to prevent the user from getting bored early.
Design:
Initially, I thought of drawing the background for each level and then uploading the picture onto p5 but then that did not look so good.
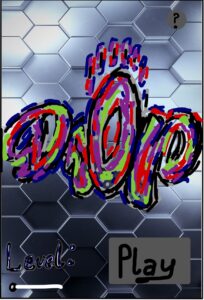
But then this was not nice so I decided to go with a different design. I decided to just use a simple design and add a cool background with the bee theme. something like a honey comb. The rest of the design was mainly thoughts of how to structure the whole game into classes and what each class would end up doing.
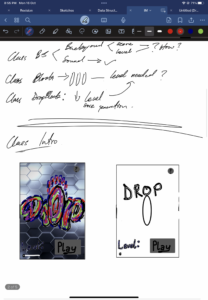
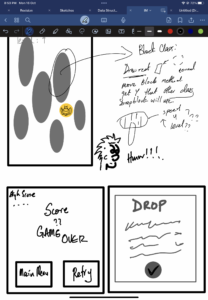
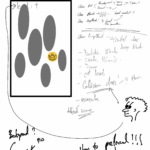
Challenging Parts of this project:
The most challenging part of this project was preloading the different files that I imported into the project. This took me h0urs to figure out and a piece of advice is to always preload all the imports in one class and not various classes.
function preload(){
//in this preload function we will load all the uploads we need before we even start the game. The folder name is always mentioned before the file name to help the compiler to know the location of the file we want to add.
B1=loadImage("Background/B1.jpg");//these sets are for the background
B2=loadImage("Background/B2.jpg");
B3=loadImage("Background/B3.jpg");
B4=loadImage("Background/B4.jpg");
B5=loadImage("Background/B5.jpg");
B6=loadImage("Background/B6.jpg");
B7=loadImage("Background/B7.jpg");
B8=loadImage("Background/B8.jpg");
IB=loadImage("Background/IB.jpg");
GB=loadImage("Background/GB.jpg");
F1=loadFont("Fonts/Font1.ttf");//these sets are for the fonts
F2=loadFont("Fonts/Font2.ttf");
S1=loadSound("Sounds/unplay.mp3");//these sets are for the sounds
S2=loadSound("Sounds/select.mp3");
S3=loadSound("Sounds/hover.mp3");
S4=loadSound("Sounds/hit.mp3");
S5=loadSound("Sounds/play.mp3");
P1=loadImage("Players/bee.png");// this one is to load the player png
}
Another recent challenge was the implementation of the sounds. I learnt something new with this challenge that to pass a variable by reference we do not need to create a variable in class as in “this.variablename” we just have to pass the variable and use it in the class by reference. In that case, the main variable is affected by what ever we do. This helps prevent creating duplicates of the same sound.
S4.play();//play the hit sound
S1.play();//play the sound played when not playing. In this case I use S4 and not this,S4 because i want to access an object by reference to have control of the object that was initially playing in the game regardless of the objects location. creating this.S4 will create a copy and we would either start the sound or end up playing over another preexisting sound
S5.pause();//pause the in game sound... yeah the annoying one
Another tricky aspect was the collision and the deletion of the blocks after they cross the end of the canvas. In my case, each level has its own threshold that needs to be attained before the level is incremented. Silly me, I wanted to incorporate this in another class which wasted a lot of my time all for nothing. I realized that in as much as you want your work to look clean and separated you should know that you can utilize a class as much as you want and that there is nothing wrong with having a large class. Also about the collision, The “png” was resized to be a square so in order to get the collision effect, I had to use the “dist” function in my case to aid me to draw the circle that would be used to detect the intersection
collision(){//this method is the one that checks if the play has collided with the falling blocks. It does this by going through the array and checking if the arrays height and width in accordance to the x and y coordinate intersect with the players circular dimensions.
for(let i=0;i<this.lvl;i++){
if(
mouseX+10>this.blockArray[i].x&&mouseX-10<this.blockArray[i].x+this.blockArray[i].w&&
mouseY+10>this.blockArray[i].y+5&&mouseY-10<this.blockArray[i].y+this.blockArray[i].h-5){//if there is an intersection,
deleteBlock(){//this method deletes blocks...used to delete blocks when they cross the height of the canvas.
for(let i=0;i<(this.lvl);i++){
if(this.blockArray[i].getY()>height){//if the top of the object for each object in the array has passed the height of the canvas
this.score++;//increment the score by one.
//for every level there is a score boundary that one is suppose to reach to move to the next level the if else code block below sets every level's boundary and increments the number of blocks for each level while increasing the level.
if(this.lvl==1&&(this.score%3)==0){//if the score is 3, and we are on level one, increase the level and add one block object to the array
this.blockArray[this.lvl]=new Block(this.lvl);//add the block
this.blockArray[this.lvl].setBlock();//setting the new block that has been added
this.blockArray[this.lvl].drawBlock();//draw the block that was jusr added
this.lvl++//increment the lvl
}
else if(this.lvl==2&&(this.score%13)==0){//similar to above and below but each statement has its own treshold
this.blockArray[this.lvl]=new Block(this.lvl);
this.blockArray[this.lvl].setBlock();
this.blockArray[this.lvl].drawBlock();
this.lvl++
}
else if(this.lvl==3&&(this.score%23)==0){
this.blockArray[this.lvl]=new Block(this.lvl);
this.blockArray[this.lvl].setBlock();
this.blockArray[this.lvl].drawBlock();
this.lvl++
}
else if(this.lvl==4&&(this.score%33)==0){
this.blockArray[this.lvl]=new Block(this.lvl);
this.blockArray[this.lvl].setBlock();
this.blockArray[this.lvl].drawBlock();
this.lvl++
}
else if(this.lvl==5&&(this.score%45)==0){
this.blockArray[this.lvl]=new Block(this.lvl);
this.blockArray[this.lvl].setBlock();
this.blockArray[this.lvl].drawBlock();
this.lvl++
}
else if(this.lvl==6&&(this.score%60)==0){
this.blockArray[this.lvl]=new Block(this.lvl);
this.blockArray[this.lvl].setBlock();
this.blockArray[this.lvl].drawBlock();
this.lvl++
}
else if(this.lvl==7&&(this.score%80)==0){
this.blockArray[this.lvl]=new Block(this.lvl);
this.blockArray[this.lvl].setBlock();
this.blockArray[this.lvl].drawBlock();
this.lvl++
}
else if(this.lvl==8&&(this.score%100)==0){
this.blockArray[this.lvl]=new Block(this.lvl);
this.blockArray[this.lvl].setBlock();
this.blockArray[this.lvl].drawBlock();
this.lvl++
if(this.lvl==9){//since level 8 is the last level, if, the level goes to level 9, decrement the level back to level 8.
this.lvl--;//decrement the level
}
}
delete this.blockArray[i];//if the block has passed the height, delete the block object,
this.blockArray[i]=new Block(this.lvl);//create a new object and replace it with the old one that was deleted
this.blockArray[i].setBlock();//set block to give it a new x coordinate and y coordinate and size etc. we donot draw the block because we need to draw it just once
}
}
}
Another major challenge was figuring out how the background moves which also took a lot of time to understand but it was cool after implementing it. However I realized that at the page_end and the page_front, the distinction is visible so next time I will look for Images that have this incorporated.
image(this.BG,0,this.y1,width,height);//create image at this.y1
image(this.BG,0,this.y2,width,height);//create image at this.y2
this.y1 -= this.scrollspeed;//decrement the speedd for both
this.y2 -= this.scrollspeed;
if (this.y1 < -height){//if the top of this background is at -height reset it to height
this.y1 = height;
}
if (this.y2 < -height){//repeat the above for this y2 too
this.y2 = height;
}
}
Project Snapshots:
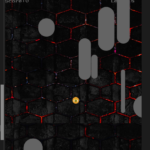
Thoughts for the Future:
I hope that in future I can fix or get Backgrounds that do not have visible transitions. And also I hope to be able to incorporate motion detecting to allow the user to control the player by just moving their head.
Final work: