The concept for this piece was inspired by the kids toy, “Sensory Liquid Floor” which creates a nice colorful aesthetic. The same concept was applied on the canvas making the mouse be the “kid” and when moving, the mouse acts as if there is movement on the floor, changing the color of the screen to the background color. Another function a “mouse pressed” function that changes the color of the screen to the background color when the mouse is pressed.
Figure 1 – Liquid Floor Toy:
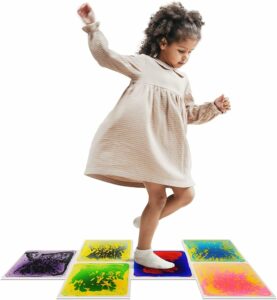
Move the mouse around! Mouse click!
The first notable layer was a grid made of squares just as figure 2 shows. Then, a colorful gradient background with rainbow highlights that was painstakingly created using color interpolation to guarantee a fluid, flawless movement across the spectrum. Then, each square was programmed to react to the mouse cursor’s proximity, giving the piece its interactive quality. The vibrant colors beneath the squares were gradually revealed as the cursor traveled over them; the color blending and visibility were expertly calibrated to produce a fluid, organic interaction. The hard edges of the grid were softened, which improved the harmony of the squares against the background. The strokes of the squares were matched with the rainbow background to give it a smooth look and also hide the grid that was created using the loops. By doing this, the piece looked more interactive as the user is now able to move the mouse around and see colors appear without seeing the color of the whole background or the grid itself.
Figure 2:
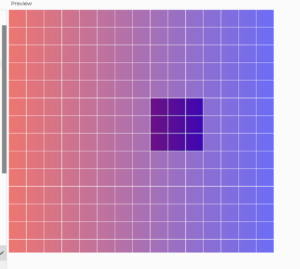
I am proud of two parts of my code. The first part being:
// Calculate grid cell of current mouse position
let hoverX = Math.floor(mouseX / spacing) * spacing;
let hoverY = Math.floor(mouseY / spacing) * spacing;
// Loop through grid of squares
for (let x = 0; x <= width; x += spacing) {
for (let y = 0; y <= height; y += spacing) {
// Calculate distance from current square to mouse square
let distance = max(abs(hoverX - x), abs(hoverY - y)) / spacing;
// Decide transparency based on distance from mouse
if (distance === 0) {
// Directly under mouse
noFill();
} else if (distance === 1) {
// Immediately adjacent to the selected square
fill(255, 128);
} else if (distance === 2) {
// Set of squares after adjacent ones
fill(255, 192);
} else {
// Squares further away are completely white.
fill(255);
}
This part of the code is responsible for creating an interactive grid where the color and transparency of each square dynamically respond to the position of the mouse cursor. The purpose is to provide a visual representation of proximity, with the nearest square to the cursor revealing the background color and the surrounding squares fading out based on their distance from the cursor. First, hoverX and hoverY variables are calculated by dividing the mouse’s x and y coordinates (mouseX and mouseY) by the spacing between the squares, using Math.floor to round down to the nearest whole number, then multiplying by spacing again. This snaps the mouse position to the nearest top-left corner of the grid squares, effectively determining which square the mouse is hovering over. Two nested loops run through each square in the grid. The outer loop (x) runs across the width of the canvas, and the inner loop (y) runs down the height of the canvas. The loops use spacing to step from one square to the next. For each square, the code calculates its distance from the square the mouse is over (hoverX, hoverY). This is done by finding the maximum of the absolute differences in x and y coordinates (max(abs(hoverX – x), abs(hoverY – y)) and dividing by spacing to get the distance in terms of squares, not pixels.
The second part was the function that created the rainbow background:
// Function to draw rainbow background
function drawRainbowBackground() {
noFill();
// Loop through the width of canvas to create gradient effect
for (let i = 0; i < width; i++) {
// Map position to range between 0 and 1 for color interpolation
let inter = map(i, 0, width, 0, 1);
// Interpolate between two colors based on current position
let c = lerpColor(color(255, 0, 0), color(0, 0, 255), inter);
// Set stroke color for line
stroke(c);
// Draw vertical line at position 'i' spanning height of canvas
line(i, 0, i, height);
}
}
This part of the code is responsible for creating a visually appealing rainbow gradient background across the canvas. The gradient smoothly transitions between two colors horizontally, providing a vibrant backdrop for the interactive squares. First, ‘for (let i = 0; i <width; i++)’ is a loop that runs once for every pixel along the canvas’s width. ‘i’ represents the current x-coordinate. It starts at 0 and increases by 1 until it reaches the canvas’s width, ensuring that the gradient effect spans the entire horizontal width of the canvas. Then, ‘let inter = map(i, 0, width, 0, 1)’ re-maps the x-coordinate (i) from the range of 0 to width (the canvas’s width) to a new range of 0 to 1. Finally, let c = lerpColor(color(255, 0, 0), color(0, 0, 255), inter) blends two colors together. Here, it’s used to create a transition between red and blue.