Sketch:
link to fullscreen: https://editor.p5js.org/GhadirMadani/full/e7SLueayo
Concept:
The concept of my project was to make the user interact with more than one game using only one game. So my idea was to make an arcade game with five arcade machines, and when the user clicks on the screen of one of the arcade machines they will be able to play the game linked to that screen, and then they can go back to the arcade room to play the other games. By creating this game, I incorporated a lot of what we have studied in class and I made it more user-friendly and interactive.
How the Project Works:
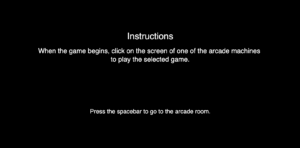
When you enter the game this is the first screen that is shown, if you follow the instructions correctly it will link you to the arcade room code.
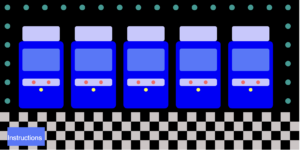
This is the arcade room. You can go back to the instructions page by clicking the “Instructions” button and it will link you back to the instructions code. Or you can play on the screen of one of the arcade machines (as mentioned in the instructions page) to play the game linked to it.
function mousePressed() {
// Check clicks only when in the arcade room
// First arcade machine (redirects to skeleton.html)
if (mouseX >= 60 && mouseX <= 160 && mouseY >= 130 && mouseY <= 190) {
window.location.href = "skeleton.html";
}
// Second arcade machine (redirects to squares.html)
if (mouseX >= 190 && mouseX <= 290 && mouseY >= 130 && mouseY <= 190) {
window.location.href = "squares.html";
}
// Third arcade machine (redirects to error.html)
if (mouseX >= 320 && mouseX <= 420 && mouseY >= 130 && mouseY <= 190) {
window.location.href = "error.html";
}
// Fourth arcade machine (redirects to jukebox.html)
if (mouseX >= 450 && mouseX <= 550 && mouseY >= 130 && mouseY <= 190) {
window.location.href = "jukebox.html";
}
// Fifth arcade machine (redirects to jackpot.html)
if (mouseX >= 580 && mouseX <= 680 && mouseY >= 130 && mouseY <= 190) {
window.location.href = "jackpot.html";
}
// Check if the "Instruction" button is clicked
if (mouseX >= 20 && mouseX <= 120 && mouseY >= height - 60 && mouseY <= height - 10) {
// Redirect to the arcade home page
window.location.href = "index.html";
}
}
The code I am mostly proud of is linking each screen with a different game, for example the first one is linked to the skeleton game, the second to the squares and so on.
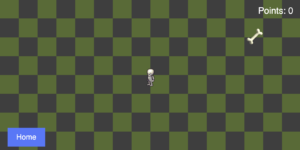
When you click on the first screen you are directed to the skeleton game. You will have an instruction page before playing the game and if you follow the instructions carefully you will be able to play the game. The way this game works is you have to move the skeleton (spritesheet) to collect the bones, when you reach 10 points you win the game and you can press the “R” key to restart the game. But you can also lose the game if you touch the borders of the screen and you can also restart the game by pressing the “R” key. If you want to go back to the arcade room you have to press the “Home” button.
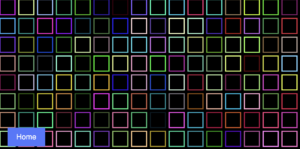
The second game (the second arcade machine) is a previous assignment that I added to this project. You will have to press on the mouse to let the squares change and press it again to change the speed of the squares. What I added to this project was the instruction page, where if you follow the instructions you will be able to play the game. If you want to restart the game you will have to press the spacebar twice, one to stop the game and one to reset the squares and then you will be able to play the game again. If you want to go back to the arcade room you have to press on the “Home” button.
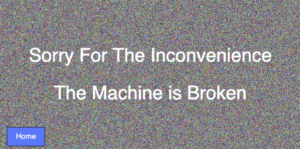
For my third game I created non functional game, and this is because whenever I go to an arcade room most of the machines are broken. You can go back to the arcade room by pressing the “Home” button.
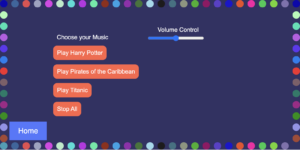
For my fourth game I created a jukebox, this was to implement music to my game. When you click on one of the “Play” buttons the corresponding music will be played and if you press on the stop button the music will stop playing. You can control the volume levels using the “Volume Control” slider. You can go back to the arcade room by pressing the “Home” button.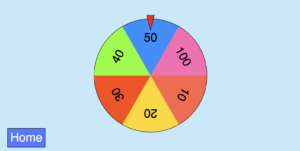
For my last game I created a Jackpot where you have to press your mouse so the wheel spins. You can then go back to the arcade room by pressing the “Home” button.
Areas of Improvement and Problems:
Since my project had many javascript codes and the games were dependent on the size of the screen that was chosen (width=800 and height=400), it was really difficult for me to code all the different javascripts to be in full size and responsive to the size of the screen. So as an improvement for my future project I want to implement the full screen option and make my project responsive to the size of the screen being used.