Concept
I was originally playing around on p5js with Perlin nose trying to learn it, until it finally began taking shape and I thought of the opening credits of Monsters Inc., specially the scene with the doors being at the end before the title of the movie was shown. (I’ve put a link to the video, it is from minute 1:05 to around 1:10-1:15)
https://www.youtube.com/watch?v=FCfv5P8GXU4
With my inspiration clear, I looked for ways to add different colors while still being in the same color pallet, and that’s when I found this sketch of a background changing colors with Perlin noise.
The part that was of most relevance to the code was the following, as it allowed me to get the colors I wanted
let n = noise(offset) colorMode(HSB) background(n*360, 100, 100); offset += 0.01
code
As for the code, that was a completely different adventure. It was originally difficult to try and find an idea, or get my grasps on how things worked with Perlin noise, specially with getting my head around mapping.
I think that my code ended up how it did as I did not place anything to make the area the ball moved in back to the background, but I believe it made it work better.
My code ended up looking like this:
//This code was in part done while learning about Perlin noise by the Coding Train on youtube and following his tutorials// let xOff = 0; //offset on X axis// let yOff = 10000; // offset on Y axis// let offset = 0; //offset for color// function setup() { createCanvas(400, 400); background(0); } function draw() { // used the mapping function in order for the Perlin noise to scale around with the canvas // let x = map(noise(xOff), 0, 1, 0, 400); let y = map(noise(yOff), 0, 1, 0, 400); n = noise(offset); // declared the offset in for the colors// // found out about HSB coloring with Perlin noise by this sketch https://editor.p5js.org/amcc/sketches/XcXWNTPB-// colorMode(HSB); // I wanted to make the strokes also change colors, maybe it could be done with the same result with noStroke// stroke(n * 260, 100, 100); //Making the offsets change a bit in order for the circle to move around the screen// xOff += 0.01; yOff += 0.01; //filling in with Perlin noise in order to have different but somewhat matching colors// fill(n * 255, 180, 180); ellipse(x, y, 50); offset += 1; }
My only one complain is that I wish I could have figured out how to use a specific color pallet, as having more variations would have been interesting.
Art Works
I made multiple versions of the code, either by adding or removing some strands of code.
This was the first attempt and what lead to me finding my project:
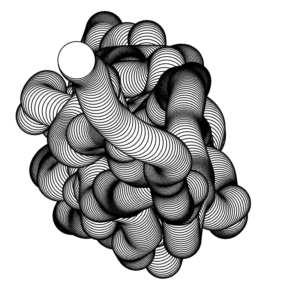
The second version I made was similar, as it had lines and in a white canvas, but the ellipses were filled with colors, this is the second version of my design:
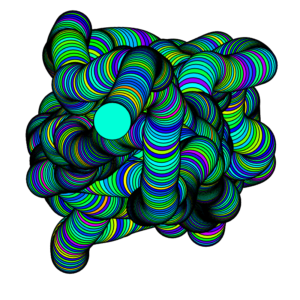
And the final version of it came a bit later, where I made the lines also change color, and made the background black in order to make the colors pop-out more:
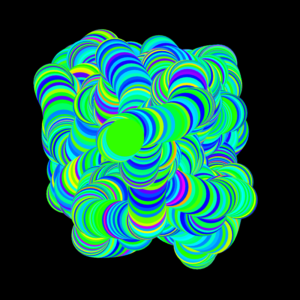
And here is a loop version of it:
reflection/future work
Personally I am happy with my end result, however I wish I could have understood more the process of creating it instead of randomly stumbling into it.
Secondly, I would have liked it to be able to use different color pallets, however I lack the skill and knowledge to do so at the moment.
Lastly it would be interesting if I could have added a mouseX or mouseY function, but with the time constraint I did not want to.