Introduction (week 11)
Coming up with an idea for this project was a challenge. I struggled with creative block and external pressures, which delayed the process. After two weeks of reflection, I decided to revisit and expand on a concept from my midterm project.
In the midterm project, the idea was to guide the main character through a maze. The narrative was that the character (the player) journeys through the maze to finally meet their parents. However, the maze portion was not fully realized. For this project, I wanted to bring this maze concept to life using p5.js and Arduino.
This final project builds on that narrative and integrates digital and physical interaction, creating an engaging and immersive experience.
Game Design and Features (week 12)
Overview
The game combines p5.js visuals with Arduino-based physical interactivity. The player navigates a conductive wire maze using a loop object. The goal is to reach the end of the maze without touching the maze walls and within a set time limit. As a key design element, the maze design spells out the word “ROBINSON,” tying back to the narrative of the midterm project. More on this later.
Arduino Program
The following are the functionalities of the project:
Inputs:
- A0 (Touch Detection): Detects if the loop touches the maze wire.
- Behavior: When a touch is detected, it sends a “Touch detected!” message to p5.js.
- A3 (Win Detection): Detects if the loop reaches the end of the maze.
- Behavior: When contact is made, it sends “WIN” to p5.js.
Outputs:
- BUZZER_PIN (Buzzer): Plays a short tone when a touch is detected during gameplay.
- Behavior: Activates only while the countdown timer is active.
The following is code snippets of how this is brought to life:
void loop() { int touchValue = digitalRead(TOUCH_PIN); // Check for touches int winValue = digitalRead(WIN_PIN); // Check for win condition if (touchValue == HIGH) { tone(BUZZER_PIN, 1000); // Activate buzzer delay(100); noTone(BUZZER_PIN); Serial.println("Touch detected!"); // Notify p5.js } if (winValue == HIGH) { Serial.println("WIN"); // Notify p5.js of win delay(500); } delay(50); // Stability delay }
p5.js Program
Inputs from Arduino:
- ‘Touch detection’
- Deducts 5 seconds from the timer.
- Triggers a red glow effect on the screen edges.
- ‘WIN’ detection
- Displays a “You Won!” message with a fireworks animation.
Outputs to Arduino:
- START: Sent when the game begins, activating Arduino’s detection logic.
- STOP: Sent when the game ends, deactivating Arduino outputs.
Key Features:
- Dynamic Timer Countdown: Starts a countdown when a timer button is selected, with a 3-second preparation countdown before the game begins.
- Touch Feedback: Deducts time and triggers a glow effect when the maze walls are touched.
- Win and Lose States: Celebrates a win with fireworks or displays a loss message if time runs out
the following are some key code snippets for how this would work:
function readSerial(data) { if (gameState === "playing") { const trimmedData = data.trim(); if (trimmedData === "Touch detected!") { glowEffect = true; clearTimeout(glowTimer); glowTimer = setTimeout(() => (glowEffect = false), 100); timeLeft = Math.max(0, timeLeft - 5); // Deduct 5 seconds } if (trimmedData === "WIN" && timeLeft > 0) { clearInterval(timerInterval); writeSerial("STOP\n"); gameState = "win"; // Transition to the win state } } } function startGame(time) { timeLeft = time; gameState = "countdown"; let countdownTime = 3; let countdownInterval = setInterval(() => { countdownTime--; if (countdownTime <= 0) { clearInterval(countdownInterval); gameState = "playing"; writeSerial("START\n"); startTimer(); } }, 1000); }
Execution Process (progress)
Physical Setup
- Maze Design: The maze spells out “ROBINSON,” aligning with the narrative. This design adds a storytelling element to the game while providing a challenging maze path.
- Material Used: Conductive wire shaped into the letters of “ROBINSON.”
- Support Structure: Cut wood panels to create a stable base for the maze.
- Process: Shaped the conductive wire carefully to form the letters and attached it to the wood base for durability and aesthetics.
the following image is an attempt made to spell the word mentioned above using conductive wire:
The following images show the progress of this project through wires, woodcutting and soldering:
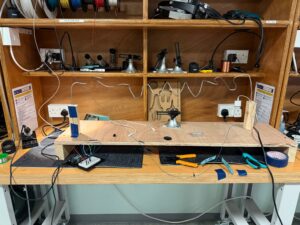
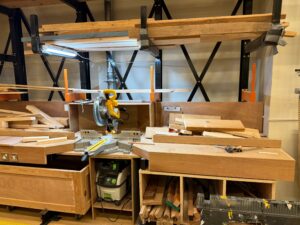
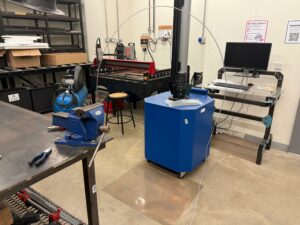
Wiring Schematic
- A0: Connected to the loop object and configured with a pull-down resistor for touch detection.
- A3: Configured for detecting contact at the maze endpoint.
- BUZZER_PIN: Connected to a larger external buzzer to provide audible feedback during gameplay.
The following is the schematic used for this project:
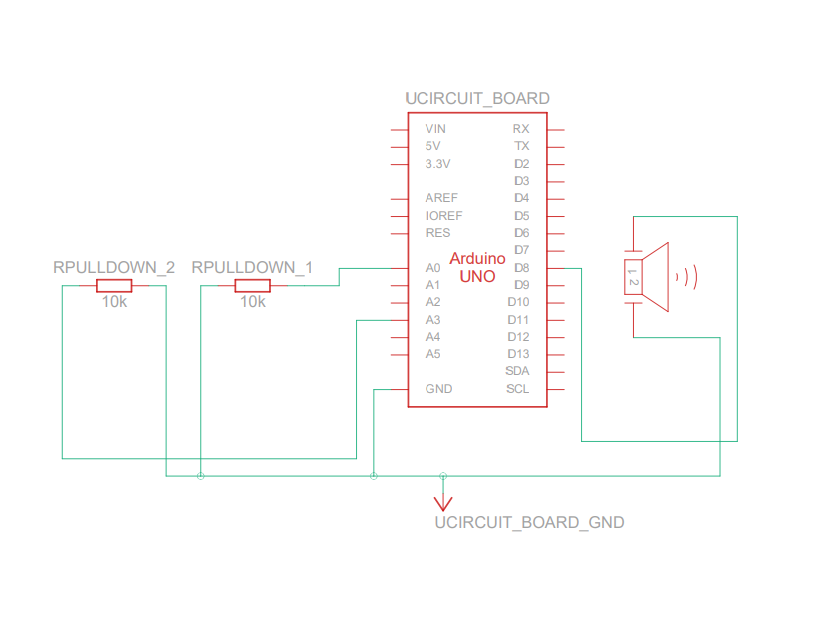
This concludes the progress made for the maze program. After completing this progress, this is when I came up with the name for it: The Robinson Maze.