Concept
Imagine a universe where two planets exist on the same orbit. For many years, I’ve been working on fictional stories, and I wanted to bring one of these concepts to life using p5.js for this assignment. The idea emerged a while ago, and although it was inspired by sci-fi media, I can’t say I’m directly mimicking anyone else’s work. The concept for this project is to make the two planets move 180 degrees apart, as if they are locked in orbit, to prevent them from crashing into each other (I’m aware that real planets don’t behave like this). This is how the project began. During the planning stage, I used Adobe Photoshop to composite some images, which helped me visualize how I would approach the project.
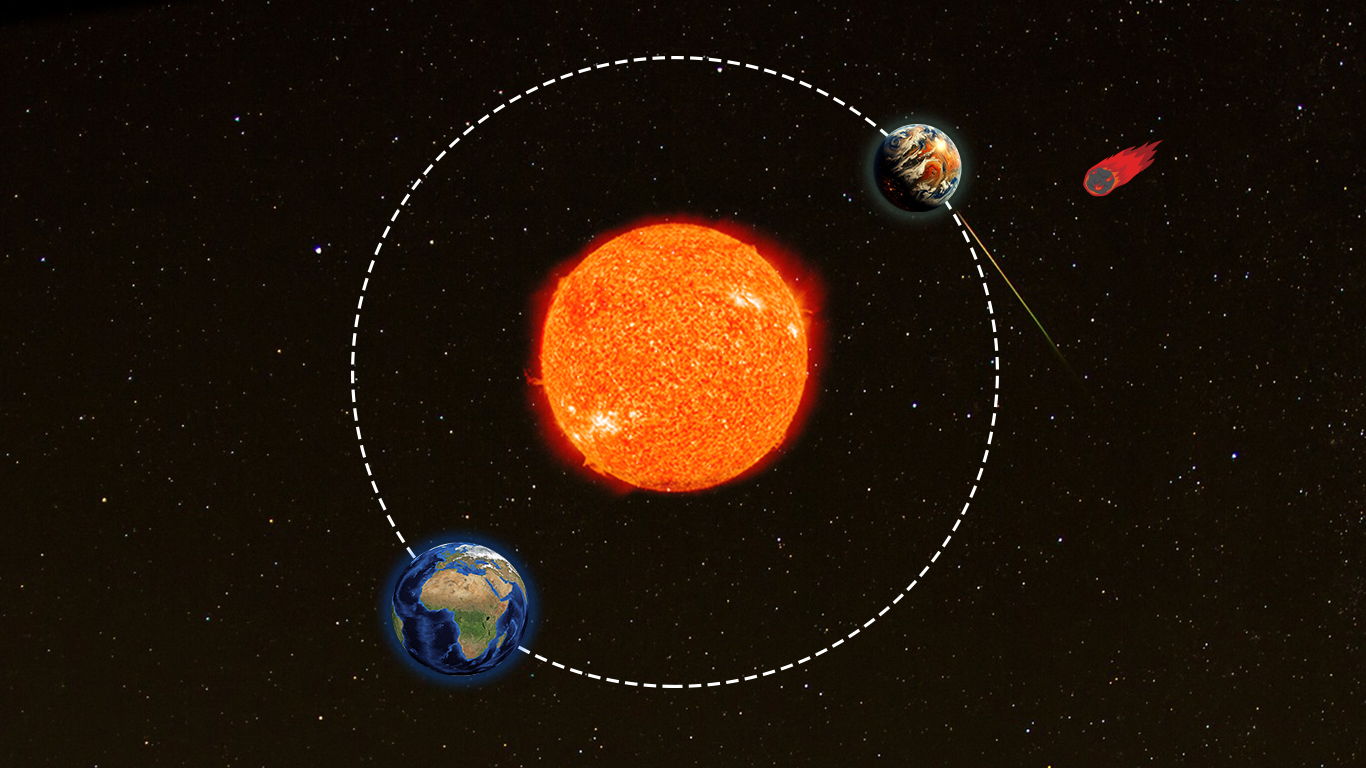
The Procedure
First, I began by creating functions to generate the stars. I didn’t want the stars to move, but they needed to be positioned randomly. The following code snippet demonstrates how I randomly generated the stars and created another function to store their positions.
// Function to generate stars and store their positions, size, and color function generateStars(numStars) { for (let i = 0; i < numStars; i++) { let x = random(0, width); // Random x position let y = random(0, height); // Random y position let size = random(1, 3); // Random size // Colors for stars (magenta, blue, yellow, and white) let colr = [color('magenta'), color('blue'), color('yellow'), color(255)]; // Store each star's position, size, and color starPositions.push({ x: x, y: y, size: size, colr: random(colr) }); } } // Function to draw stars from stored positions function drawStars() { for (let i = 0; i < starPositions.length; i++) { let star = starPositions[i]; stroke(star.colr); // Set color for each star strokeWeight(star.size); // Set size for each star point(star.x, star.y); // Draw the star } }
In this project, I used various functions, including one to detect comet collisions with planets and another for collisions with the sun. The comets were created using a class and stored in a dynamic array, making memory management simpler compared to other programming languages. The project involved a lot of mathematical concepts, especially for the comet class, and I drew inspiration from p5.js projects and AI-assisted planning. I experimented through trial and error to execute certain parts.
Code I’m most proud of:
The code I’m most proud of is the Planet class, which brings the entire concept to life using trigonometry. By applying cosine and sine functions to measure angles, I was able to make the planets behave as intended. This idea came from Google searches. Here’s a snippet of my Planet class:
class Planet { constructor(size, colr, strokecolor) { this.size = size; this.colr = color(colr); // Fill color this.strokecolor = strokecolor; // Outline color this.x = 0; // X position (calculated later) this.y = 0; // Y position (calculated later) } // Update the planet's position based on the angle and radius update(angle, radius) { this.x = width / 2 + cos(angle) * radius; // X position this.y = height / 2 + sin(angle) * radius; // Y position } // Show the planet show() { stroke(this.strokecolor); // Outline color strokeWeight(2) fill(this.colr); // Fill color ellipse(this.x, this.y, this.size); // Draw the planet } }
In my draw function, I used the following code, utilizing the principles of Object Oriented Programming to bring this idea to fruition.
// Update and draw the planets planet1.update(angle, radius); planet1.show(); planet2.update(angle + PI, radius); // The PI angle ensures planets are always opposite each other planet2.show(); // Increment the angle for continuous rotation angle += 0.01;
The Final Project:
Reflection:
For this project, I was a bit ambitious when I first started. I prioritized realism over functionality to give the viewer a great experience. However, I learned that this wasn’t necessary and settled for a simpler approach using Object-Oriented Programming. After reading The Art of Interactive Design, I realized my final project lacked key elements of interactivity, like even a simple mouse click. In my defense, I removed interactivity because, in the storyline, humans don’t have the power to move objects in space. However, I would improve the project by adding music, moving stars, mouse-click-generated comets, and perhaps better visuals. I faced challenges in ensuring the planets always rotated 180 degrees apart, but after solving this, I was amazed at what code can achieve. I’m excited to see what it can do with more advanced graphics in the future. For now, this is it.