Catch The Dates
Why not use the “Fruit Ninja” game, which I spent years of my childhood playing, serve as the inspiration for this minigame? With little consideration, “Catch the dates” turned into a mini game.
Dates and explosives will begin to fall from the NYUAD palm trees that serve as the game’s backdrop as soon as you begin playing. The objective is to avoid receiving a score of zero or below. The player can score two points on dates, but their score will be reduced by three if they catch the bomb in the basket.
One thing that I’m most proud of is the game itself as a whole. Being an extremely indecisive person, it was hard for me to just stick with one idea. However, with careful consideration I came to being excited to code this small game itself while including a part of my childhood in it while embedding culture and symbolizing the UAE’S culture and heritage by using dates instead of fruits.
One challenge I have encountered when coding this midterm, was the basket leaving a trace at the bottom as well as the background being not too flexible with height and fit on the screen.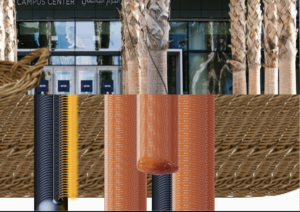
However, I solved that issue with adjusting the image width and height in order to fit perfectly.
In conclusion, this midterm really made me step out of my comfort zone. In reality I am not too proud of the work that I achieve nor do I like to share it, however, with this course, I was able to achieve that and step out of my comfort zone a little bit and be proud of the work I have accomplished especially when creating this little game.
let basketScale = 0.5; // Adjust basket scale
let nextDateFrame = 0; // Frame count for next date
let nextBombFrame = 0; // Frame count for next bomb
let scoreBoxWidth = 200; // Width of score box
let scoreBoxHeight = 50; // Height of score box
bg = loadImage('palm trees.png');
basketImg = loadImage('basket.png');
// Setup canvas and game state
createCanvas(800, 800); // Larger canvas size
basket = createVector(width / 2, height - basketImg.height * basketScale); // Adjusted basket size
nextDateFrame = 0; // Reset frame count for next date
nextBombFrame = 0; // Reset frame count for next bomb
image(bg, 0, 0,width *1.5 , height*1.5);
// Display instructions if game not started
// Display game over screen if game is over
image(basketImg, basket.x, basket.y, basketImg.width * basketScale, basketImg.height * basketScale); // Adjusted basket size
// Move basket continuously when arrow keys are held down
if (keyIsDown(LEFT_ARROW) && basket.x > 0) {
if (keyIsDown(RIGHT_ARROW) && basket.x < width - basketImg.width * basketScale) {
// Move and display dates
if (frameCount >= nextDateFrame) {
let date = new FallingObject('dates');
nextDateFrame = frameCount + int(random(120, 240)); // Randomize next date appearance
for (let date of dates) {
if (date.checkCollision(basket.x, basket.y, basketImg.width * basketScale, basketImg.height * basketScale)) {
// Move and display bombs
if (frameCount >= nextBombFrame) {
let bomb = new FallingObject('bomb');
nextBombFrame = frameCount + int(random(120, 240)); // Randomize next bomb appearance
for (let bomb of bombs) {
if (bomb.checkCollision(basket.x, basket.y, basketImg.width * basketScale, basketImg.height * basketScale)) {
rect(width - scoreBoxWidth, 0, scoreBoxWidth, scoreBoxHeight);
text(`Score: ${score}`, width - 10, 10);
// Start game on spacebar press
if (!gameStarted && key === ' ') {
// Reset game on 'r' press
if (gameOver && key === 'r') {
// Display game instructions
function displayInstructions() {
text("CATCH THE DATES", width/2 +190, height/2 -150);
text('Instructions:',width / 2 +120 , height / 2 -90);
text('Use arrow keys to move the basket',width / 2 + 300 , height / 2 -40);
text('Catch dates to score points',width / 2 + 250 , height / 2);
text('Avoid bombs',width / 2 + 120 , height / 2 + 40);
text('Press space to start',width / 2 + 180 , height / 2 + 80);
// Display game over screen
function displayGameOver() {
triangle(width / 2, height / 2 - 100, width / 2 - 150, height / 2 + 150, width / 2 + 150, height / 2 + 150);
textAlign(CENTER, CENTER);
text('Game Over!', width / 2, height / 2 + 30);
text('Press "r" to play again', width / 2, height / 2 + 118);
this.speed = random(2.5, 3.5); // Adjusted speed
this.image = loadImage(`${type}.png`);
// Move the falling object
// Display the falling object
image(this.image, this.x, this.y, 50, 50);
// Reset the falling object's position
this.speed = random(2.5, 3.5); // Adjusted speed
// Check for collision with another object
checkCollision(objX, objY, objWidth, objHeight) {
return this.x > objX && this.x < objX + objWidth && this.y > objY && this.y < objY + objHeight;
// Declare variables
let titleSize = 35
let bg;
let basketImg;
let dates = [];
let bombs = [];
let score = 0;
let gameStarted = false;
let gameOver = false;
let basket;
let basketScale = 0.5; // Adjust basket scale
let basketSpeed = 7;
let nextDateFrame = 0; // Frame count for next date
let nextBombFrame = 0; // Frame count for next bomb
let scoreBoxWidth = 200; // Width of score box
let scoreBoxHeight = 50; // Height of score box
// Preload images
function preload() {
bg = loadImage('palm trees.png');
basketImg = loadImage('basket.png');
}
// Setup canvas and game state
function setup() {
createCanvas(800, 800); // Larger canvas size
textAlign(RIGHT, TOP);
textSize(24);
resetGame();
}
// Reset game state
function resetGame() {
score = 0;
gameStarted = false;
gameOver = false;
dates = [];
bombs = [];
basket = createVector(width / 2, height - basketImg.height * basketScale); // Adjusted basket size
nextDateFrame = 0; // Reset frame count for next date
nextBombFrame = 0; // Reset frame count for next bomb
}
// Main game loop
function draw() {
// Display background
image(bg, 0, 0,width *1.5 , height*1.5);
// Display instructions if game not started
if (!gameStarted) {
displayInstructions();
return;
}
// Display game over screen if game is over
if (gameOver) {
displayGameOver();
return;
}
// Display basket
image(basketImg, basket.x, basket.y, basketImg.width * basketScale, basketImg.height * basketScale); // Adjusted basket size
// Move basket continuously when arrow keys are held down
if (keyIsDown(LEFT_ARROW) && basket.x > 0) {
basket.x -= basketSpeed;
}
if (keyIsDown(RIGHT_ARROW) && basket.x < width - basketImg.width * basketScale) {
basket.x += basketSpeed;
}
// Move and display dates
if (frameCount >= nextDateFrame) {
let date = new FallingObject('dates');
dates.push(date);
nextDateFrame = frameCount + int(random(120, 240)); // Randomize next date appearance
}
for (let date of dates) {
date.move();
date.display();
if (date.checkCollision(basket.x, basket.y, basketImg.width * basketScale, basketImg.height * basketScale)) {
date.reset();
score += 2;
}
}
// Move and display bombs
if (frameCount >= nextBombFrame) {
let bomb = new FallingObject('bomb');
bombs.push(bomb);
nextBombFrame = frameCount + int(random(120, 240)); // Randomize next bomb appearance
}
for (let bomb of bombs) {
bomb.move();
bomb.display();
if (bomb.checkCollision(basket.x, basket.y, basketImg.width * basketScale, basketImg.height * basketScale)) {
bomb.reset();
score -= 3;
if (score <= 0) {
score = 0;
gameOver = true;
}
}
}
// Display score
fill(0, 0, 255);
rect(width - scoreBoxWidth, 0, scoreBoxWidth, scoreBoxHeight);
fill(255);
textAlign(RIGHT, TOP);
text(`Score: ${score}`, width - 10, 10);
}
// Handle key presses
function keyPressed() {
// Start game on spacebar press
if (!gameStarted && key === ' ') {
gameStarted = true;
}
// Reset game on 'r' press
if (gameOver && key === 'r') {
resetGame();
}
}
// Display game instructions
function displayInstructions() {
fill(255);
stroke(2);
strokeWeight(5);
textSize(titleSize);
text("CATCH THE DATES", width/2 +190, height/2 -150);
fill(255);
text('Instructions:',width / 2 +120 , height / 2 -90);
text('Use arrow keys to move the basket',width / 2 + 300 , height / 2 -40);
text('Catch dates to score points',width / 2 + 250 , height / 2);
text('Avoid bombs',width / 2 + 120 , height / 2 + 40);
text('Press space to start',width / 2 + 180 , height / 2 + 80);
}
// Display game over screen
function displayGameOver() {
fill(255, 0, 0);
triangle(width / 2, height / 2 - 100, width / 2 - 150, height / 2 + 150, width / 2 + 150, height / 2 + 150);
fill(255);
textAlign(CENTER, CENTER);
textSize(25);
stroke(1);
strokeWeight(3);
text('Game Over!', width / 2, height / 2 + 30);
text('Press "r" to play again', width / 2, height / 2 + 118);
}
// FallingObject class
class FallingObject {
constructor(type) {
this.x = random(width);
this.y = -50;
this.speed = random(2.5, 3.5); // Adjusted speed
this.type = type;
this.image = loadImage(`${type}.png`);
}
// Move the falling object
move() {
this.y += this.speed;
if (this.y > height) {
this.reset();
}
}
// Display the falling object
display() {
image(this.image, this.x, this.y, 50, 50);
}
// Reset the falling object's position
reset() {
this.x = random(width);
this.y = -50;
this.speed = random(2.5, 3.5); // Adjusted speed
}
// Check for collision with another object
checkCollision(objX, objY, objWidth, objHeight) {
return this.x > objX && this.x < objX + objWidth && this.y > objY && this.y < objY + objHeight;
}
}
// Declare variables
let titleSize = 35
let bg;
let basketImg;
let dates = [];
let bombs = [];
let score = 0;
let gameStarted = false;
let gameOver = false;
let basket;
let basketScale = 0.5; // Adjust basket scale
let basketSpeed = 7;
let nextDateFrame = 0; // Frame count for next date
let nextBombFrame = 0; // Frame count for next bomb
let scoreBoxWidth = 200; // Width of score box
let scoreBoxHeight = 50; // Height of score box
// Preload images
function preload() {
bg = loadImage('palm trees.png');
basketImg = loadImage('basket.png');
}
// Setup canvas and game state
function setup() {
createCanvas(800, 800); // Larger canvas size
textAlign(RIGHT, TOP);
textSize(24);
resetGame();
}
// Reset game state
function resetGame() {
score = 0;
gameStarted = false;
gameOver = false;
dates = [];
bombs = [];
basket = createVector(width / 2, height - basketImg.height * basketScale); // Adjusted basket size
nextDateFrame = 0; // Reset frame count for next date
nextBombFrame = 0; // Reset frame count for next bomb
}
// Main game loop
function draw() {
// Display background
image(bg, 0, 0,width *1.5 , height*1.5);
// Display instructions if game not started
if (!gameStarted) {
displayInstructions();
return;
}
// Display game over screen if game is over
if (gameOver) {
displayGameOver();
return;
}
// Display basket
image(basketImg, basket.x, basket.y, basketImg.width * basketScale, basketImg.height * basketScale); // Adjusted basket size
// Move basket continuously when arrow keys are held down
if (keyIsDown(LEFT_ARROW) && basket.x > 0) {
basket.x -= basketSpeed;
}
if (keyIsDown(RIGHT_ARROW) && basket.x < width - basketImg.width * basketScale) {
basket.x += basketSpeed;
}
// Move and display dates
if (frameCount >= nextDateFrame) {
let date = new FallingObject('dates');
dates.push(date);
nextDateFrame = frameCount + int(random(120, 240)); // Randomize next date appearance
}
for (let date of dates) {
date.move();
date.display();
if (date.checkCollision(basket.x, basket.y, basketImg.width * basketScale, basketImg.height * basketScale)) {
date.reset();
score += 2;
}
}
// Move and display bombs
if (frameCount >= nextBombFrame) {
let bomb = new FallingObject('bomb');
bombs.push(bomb);
nextBombFrame = frameCount + int(random(120, 240)); // Randomize next bomb appearance
}
for (let bomb of bombs) {
bomb.move();
bomb.display();
if (bomb.checkCollision(basket.x, basket.y, basketImg.width * basketScale, basketImg.height * basketScale)) {
bomb.reset();
score -= 3;
if (score <= 0) {
score = 0;
gameOver = true;
}
}
}
// Display score
fill(0, 0, 255);
rect(width - scoreBoxWidth, 0, scoreBoxWidth, scoreBoxHeight);
fill(255);
textAlign(RIGHT, TOP);
text(`Score: ${score}`, width - 10, 10);
}
// Handle key presses
function keyPressed() {
// Start game on spacebar press
if (!gameStarted && key === ' ') {
gameStarted = true;
}
// Reset game on 'r' press
if (gameOver && key === 'r') {
resetGame();
}
}
// Display game instructions
function displayInstructions() {
fill(255);
stroke(2);
strokeWeight(5);
textSize(titleSize);
text("CATCH THE DATES", width/2 +190, height/2 -150);
fill(255);
text('Instructions:',width / 2 +120 , height / 2 -90);
text('Use arrow keys to move the basket',width / 2 + 300 , height / 2 -40);
text('Catch dates to score points',width / 2 + 250 , height / 2);
text('Avoid bombs',width / 2 + 120 , height / 2 + 40);
text('Press space to start',width / 2 + 180 , height / 2 + 80);
}
// Display game over screen
function displayGameOver() {
fill(255, 0, 0);
triangle(width / 2, height / 2 - 100, width / 2 - 150, height / 2 + 150, width / 2 + 150, height / 2 + 150);
fill(255);
textAlign(CENTER, CENTER);
textSize(25);
stroke(1);
strokeWeight(3);
text('Game Over!', width / 2, height / 2 + 30);
text('Press "r" to play again', width / 2, height / 2 + 118);
}
// FallingObject class
class FallingObject {
constructor(type) {
this.x = random(width);
this.y = -50;
this.speed = random(2.5, 3.5); // Adjusted speed
this.type = type;
this.image = loadImage(`${type}.png`);
}
// Move the falling object
move() {
this.y += this.speed;
if (this.y > height) {
this.reset();
}
}
// Display the falling object
display() {
image(this.image, this.x, this.y, 50, 50);
}
// Reset the falling object's position
reset() {
this.x = random(width);
this.y = -50;
this.speed = random(2.5, 3.5); // Adjusted speed
}
// Check for collision with another object
checkCollision(objX, objY, objWidth, objHeight) {
return this.x > objX && this.x < objX + objWidth && this.y > objY && this.y < objY + objHeight;
}
}