In this assignment, I developed a serene landscape populated by butterflies, clouds, and flowers, inviting viewers to immerse themselves in a tranquil virtual environment.
Key Elements:
– Butterflies: Colorful and graceful, the butterflies are the focal point of the scene. Programmed to flutter across the canvas, their movements are dynamic and captivating.
– Background: The canvas serves as a backdrop for the scene, featuring a gradient sky and soft, rolling clouds that drift lazily across the horizon.
– Flowers: Adding depth and detail to the landscape, flowers bloom sporadically, their vibrant colors contrasting against the lush greenery.
Conceptual Framework:
The project draws inspiration from the beauty and harmony found in the natural world. By combining artistic vision with computational techniques, the goal is to evoke a sense of wonder and serenity, inviting viewers to momentarily escape the confines of reality and immerse themselves in a digital oasis of creativity and beauty.
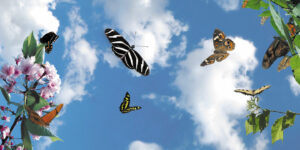
This assignment provided an opportunity to explore the intersection of art and technology, demonstrating how code can be used to create immersive digital experiences that transport viewers to captivating virtual worlds. Through careful design and implementation, the project aims to evoke a sense of wonder and appreciation for the beauty of nature in the digital realm.
code:
let butterflies = [];
let clouds = [];
let flowers = [];
function setup() {
createCanvas(800, 600);
// Create a flock of butterflies
for (let i = 0; i < 10; i++) {
let butterfly = new Butterfly(random(width), random(height));
butterflies.push(butterfly);
}
// Create some clouds
for (let i = 0; i < 5; i++) {
let cloud = new Cloud(random(width), random(height));
clouds.push(cloud);
}
// Create some flowers
for (let i = 0; i < 20; i++) {
let flower = new Flower(random(width), random(height));
flowers.push(flower);
}
}
function draw() {
// Draw gradient sky background
background(135, 206, 250); // Light blue
for (let y = 0; y < height; y++) {
let inter = map(y, 0, height, 0, 1);
let c = lerpColor(color(135, 206, 250), color(255, 255, 255), inter);
stroke(c);
line(0, y, width, y);
}
// Display and update clouds
for (let cloud of clouds) {
cloud.display();
cloud.update();
}
// Update and display each butterfly
for (let i = 0; i < butterflies.length; i++) {
butterflies[i].update();
butterflies[i].display();
}
// Display flowers
for (let flower of flowers) {
flower.display();
}
}
class Butterfly {
constructor(x, y) {
this.position = createVector(x, y);
this.velocity = createVector(random(-1, 1), random(-1, 1));
this.color = color(random(255), random(255), random(255));
this.angle = random(TWO_PI);
this.size = random(20, 40);
}
update() {
// Update position
this.position.add(this.velocity);
// Change direction randomly
if (random() < 0.01) {
this.velocity.rotate(random(-PI / 4, PI / 4));
}
// Wrap around screen
this.position.x = (this.position.x + width) % width;
this.position.y = (this.position.y + height) % height;
}
display() {
// Draw butterfly wings with pattern
fill(this.color);
noStroke();
ellipse(this.position.x, this.position.y, this.size, this.size / 2);
let wingOffset = this.size / 4;
let wingSize = this.size / 2;
push();
translate(this.position.x, this.position.y);
rotate(this.angle);
fill(255, 100); // Semi-transparent white
ellipse(-wingOffset, 0, wingSize, wingSize * 2);
ellipse(wingOffset, 0, wingSize, wingSize * 2);
fill(0, 100, 255); // Semi-transparent blue
ellipse(-wingOffset, 0, wingSize / 2, wingSize);
ellipse(wingOffset, 0, wingSize / 2, wingSize);
pop();
}
}
class Cloud {
constructor(x, y) {
this.position = createVector(x, y);
this.velocity = createVector(random(-0.5, 0.5), 0);
this.size = random(50, 100);
}
update() {
this.position.add(this.velocity);
if (this.position.x > width + this.size) {
this.position.x = -this.size;
}
if (this.position.x < -this.size) {
this.position.x = width + this.size;
}
}
display() {
noStroke();
fill(255);
ellipse(this.position.x, this.position.y, this.size * 1.5, this.size);
ellipse(this.position.x - this.size / 3, this.position.y - this.size / 4, this.size, this.size * 0.8);
ellipse(this.position.x + this.size / 3, this.position.y - this.size / 4, this.size, this.size * 0.8);
}
}
class Flower {
constructor(x, y) {
this.position = createVector(x, y);
this.size = random(10, 20);
this.color = color(random(255), random(255), random(255));
}
display() {
fill(this.color);
noStroke();
ellipse(this.position.x, this.position.y, this.size, this.size * 1.5);
fill(255, 0, 0); // Red
ellipse(this.position.x, this.position.y - this.size / 1.5, this.size * 0.8, this.size * 0.8);
}
}
Reading Response:
The first chapter of “The Art of Interactive Design” presents a thorough and insightful exploration into the true essence of interactivity. The author, Chris Crawford, dismantles the common misuse and overuse of the term by defining interactivity as a cyclic process in which two actors alternately listen, think, and speak. This definition, grounded in the analogy of a conversation, strips away the ambiguity surrounding the concept and refocuses our attention on the quality of the engagement rather than mere action and reaction.
Crawford’s critical perspective on the buzzword nature of interactivity in the 1990s and the superficiality of its application to products and technologies drives home the importance of discernment when evaluating interactive experiences. His reference to the frenzied rat race for ‘new and improved’ interactivity, often without substance, highlights the need for more profound and meaningful interactions rather than mere novelty.
The conversation example between Fredegund and Gomer excellently illustrates Crawford’s definition of interactivity, emphasizing the importance of understanding and responding within the context of an exchange. Moreover, his critique of non-interactive activities, like books and movies, offers a clear boundary between passive and interactive experiences.
Crawford’s commentary on the degrees of interactivity is particularly thought-provoking. He challenges the binary view of interactivity as either present or absent, proposing instead a continuum where the depth and richness of the interactive experience can vary. This nuanced approach allows for a more sophisticated analysis of interactivity in various contexts, from refrigerator doors to video games.
In summary, Crawford’s elucidation on interactivity is not only eye-opening but also serves as a call to action for designers and users alike to aspire for deeper, more meaningful interactions. His insistence on the necessity of all three components of the interactive process (listening, thinking, and speaking) provides a clear framework for evaluating and designing interactive systems. The first chapter sets the stage for a deeper dive into the intricacies of interactivity design and its implications for technology and society.