Concept:
For the midterm project, I plan to create the classic Angry Birds game. In addition, I got inspiration from the movie Rio, so I plan to join both universes and incorporate elements from the movie such as characters, settings, and storyline. In this game, players will use a slingshot mechanism to launch birds towards targets (e.g., enemy characters or objects) to progress through levels.
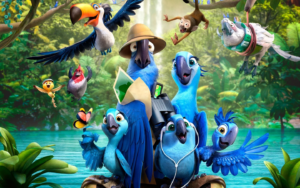
Code design:
When it comes to the code design, I will define functions for launching birds, detecting collisions, scoring points, and advancing through levels. I will also use classes for birds, targets, obstacles, and any other interactive elements I plan to implement in the game. Then, the user input mechanisms will be added for controlling the slingshot, aiming, and launching birds against the enemies.
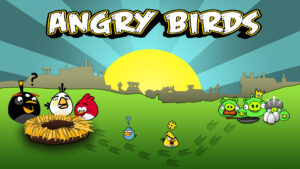
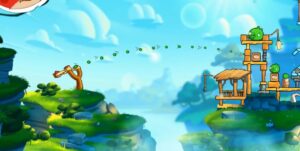
Example of a class structure from my code:
class Bird {
constructor(x, y) {
this.position = createVector(x, y);
this.velocity = createVector();
this.acceleration = createVector();
this.r = 20;
this.released = false;
}
display() {
fill(255, 255, 0);
ellipse(this.position.x, this.position.y, this.r * 2);
}
update() {
if (this.released) {
this.velocity.add(this.acceleration);
this.position.add(this.velocity);
this.acceleration.mult(0); // Reset acceleration
this.velocity.mult(0.99); // Air resistance
}
}
Challenges:
One of the most challenging aspects of my project would be implementing realistic physics for bird movement and collision detection. To minimize this risk, I will start by writing code to simulate basic projectile motion and collision detection. I also plan to use existing libraries or frameworks for physics simulations, which can help for the structure of the game.
Conclusion:
In conclusion, there are several additional elements that I plan to incorporate to improve the overall gameplay experience. Beyond the existing mechanics of slingshot aiming and bird launching, future iterations of the game will feature dynamic sound effects to accompany actions such as bird launches and target destruction. Furthermore, I aim to enhance the visual elements of the game by incorporating more detailed character sprites, vibrant backgrounds, and visually appealing animations. The current sketch provides a foundational framework for the game, and that’s how it looks like so far: