For this week’s assignment, I decided to stay away from displaying only basic shapes (not really) and instead do something different, as in having no inspiration from other sources. This code could arguably not be considered art in the sense that it does not portray an intriguing, thoughtful work; instead, it is more of an interactive piece of media that showcases data in an interactive way.
Since I also have a passion to try new and challenging ideas, I thought about implementing “gravity” and collisions in a database, and I decided to use the following CSV: Diamond Prices 2022. From this data, I removed some information that was not useful for this canvas. So, once I finish implementing my idea, we have the following canvas that displays falling diamonds, each one with a different price and size:
Even though it appears simple at first hindsight, it was difficult for me to properly implement the collisions without creating a code that could be considered spaghetti. I easily spent around 4–5 hours to make it work properly without the use of external tools for guidance; I generally tried to stay away from them since the idea of having a challenge is compelling for me. Furthermore, since I was also thinking about the user, I tried to implement as much information as possible to help the user understand what was going on “behind the cameras”.
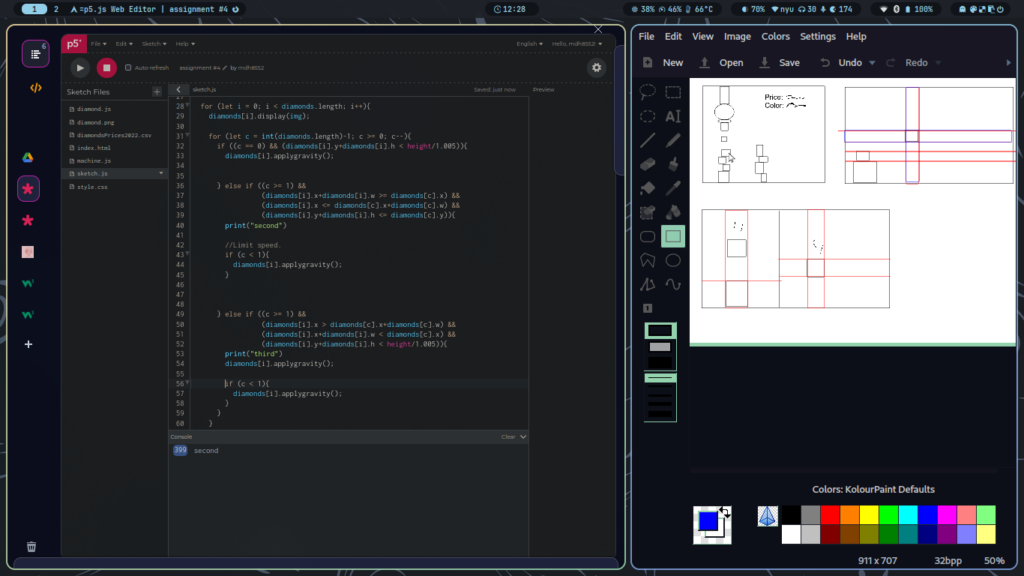
As mentioned, the implementation of the collision was challenging, although I could find a way to implement it with the following code:
//Check for collision. for (let i = 0; i < diamonds.length; i++) { diamonds[i].display(img); if (i > 0) { for (let c = diamonds.length - 2; c >= 0; c--) { if ( diamonds[i].x <= diamonds[c].x + diamonds[c].w && diamonds[i].x + diamonds[i].w >= diamonds[c].x ) { if (diamonds[c].y < diamonds[i].tempy && diamonds[c].landed == 1) { diamonds[i].tempy = diamonds[c].y; } } } if (diamonds[i].y + diamonds[i].h < diamonds[i].tempy) { diamonds[i].applygravity(); } else { diamonds[i].landed = 1; } } else if (diamonds[i].y + diamonds[i].h < height / 1.005) { diamonds[i].applygravity(); } else { diamonds[i].landed = 1; } //Check if it is safe to release another diamond given the Y and X coordinates. if (diamonds[i].y > machine.y + 140) { machine.safetorelease = 1; } else if ( machine.x + machine.w - 15 > diamonds[i].x && machine.x + machine.w - 15 < diamonds[i].x + diamonds[i].w && diamonds[i].landed == 1 ) { machine.safetorelease = 0; } else { machine.safetorelease = 1; } } //Helps to erase the last displayed diamond to avoid overlapping. if (machine.safetorelease == 0) { diamonds.pop(diamonds.length - 2); machine.safetorelease = 1; }
This code also helps to avoid overlapping between diamonds, since the machine will not stop releasing diamonds.
In conclusion, I learned a lot with this assignment, and while I wish I could find ways to implement sin()
and cos()
, this time at least I could do something different and challenging.