While brainstorming for my assignment, my initial thoughts centered around creating an art piece featuring several repetitive elements in motion. Suddenly, I recalled an image of Turkey I had seen before, specifically a picture of hot air balloons in Cappadocia.
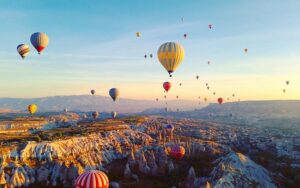
The sight of these balloons, each a different color, struck me as particularly appealing, and I decided to base my art on the concept of hot air balloons moving upwards. This idea aligned perfectly with my original concept of incorporating moving, repetitive elements.
Upon completing the hot air balloon portion of my project, I noticed the background felt somewhat empty, so I decided to make moving clouds in the background. Thus, the combination of clouds and hot air balloons became my final concept.
I started with the hot air balloons by creating a Balloon Class, aiming to generate several balloons simultaneously using the same code. Within this class, I implemented a move() function to enable the balloons to move upwards.
//move Hot Air Balloons move() { this.x = this.x + this.xspeed; this.y = this.y + this.yspeed; }
Next, I used the show() function to draw the hot air balloon.
//draw hot air balloons show() { strokeWeight(4) stroke('black') angleMode(DEGREES) line(this.x - 100, this.y + 50, this.x - 30, this.y + 183); line(this.x + 100, this.y + 50, this.x + 30, this.y + 183); fill(this.balloonColor) circle(this.x, this.y, 230); fill('rgb(172,96,96)') rect(this.x - 30, this.y + 180, 60, 40, 10); fill(this.highlightColor) arc(this.x, this.y, 110, 230, 90, 270) arc(this.x, this.y, 110, 230, 270, 90) }
To ensure each hot air balloon displayed different colors, I introduced variables like “balloonColor” and “highlightColor,” assigning them random RGB colors. The balloon featured two colors: a primary color and a “highlightColor,” which was derived by adjusting the blue component of the RGB value to complement the primary color.
//Randomize Balloon colors let red = random(255); let blue = random(255); let green = random(255); this.balloonColor = color(red, green, blue); //Highlight Color in the middle which is similar to the sum of R and B of the main color let b = (red + blue / 1.3) this.highlightColor = color(red, green, b)
To continually regenerate hot air balloons and ensure upward movement, keeping the canvas filled, I created a reset() function.
//create a new balloon and reset the animation if the hot air balloon reaches the end of the canvas reset() { if (this.y < -250) { this.y = height + 100 this.yspeed = random(-2, -5) this.x = random(width) this.xspeed = 0 }
As mentioned earlier, I wanted to include clouds in the background. Thus, I crafted a separate Cloud Class and utilized similar methods to those in the Balloon Class for showCloud(), moveCloud(), and resetCloud(). The clouds moved from left to right, and their reset function triggered upon reaching the canvas’s right edge. Cloud sizes varied randomly.
//create Cloud Class class Cloud { constructor(cloudx, cloudy, cloudspeedx, cloudspeedy) { this.x = cloudx; this.y = cloudy; this.speedx = cloudspeedx; this.speedy = cloudspeedy; this.cloudsize = random(100, 200) //randomize cloud size }
First I defined a variable “cloudsize” which should be generated randomly between 100 and 200.
//draw clouds with random sizes showCloud() { noStroke() fill('rgb(255,255,255)') circle(this.x - this.cloudsize/3, this.y, this.cloudsize - this.cloudsize/5) circle(this.x, this.y, this.cloudsize) circle(this.x + this.cloudsize/3, this.y, this.cloudsize - this.cloudsize/5) }
I then utilized “this.cloudsize” within the showCloud() function to render a cloud.
To populate the scene with multiple clouds and hot air balloons, I established arrays for each, allowing for automated element addition up to a predefined number.
let balloons = []; //create balloons array let clouds = []; //create clouds array function setup() { createCanvas(1000, 1000); //create 7 hot air balloons positioned randomly in the canvas with random speed (moving up) and add them to the balloons array for (let i = 0; i < 8; i++) balloons[i] = new Balloon(random(width), random(height), 0, random (-2, -5)) //create 9 clouds positioned randomly in the canvas with random speed (moving from left to right) and add them to the clouds array for (let i1 = 0; i1 < 10; i1++) clouds[i1] = new Cloud(random(width), random(height), random(2, 5), 0) }
It was very interesting to learn how to use arrays and classes together to create elements. The process of simultaneously producing multiple elements became significantly simpler. One challenge I encountered while crafting the art piece involved randomizing colors for each air balloon. Initially, I placed the code for randomizing colors in the sketch.js file within the “new Balloon” constructor, which resulted in the color of a single balloon changing randomly each frame. I then realized that I should incorporate this code inside the Balloon Class, utilizing `this.balloonColor` to ensure the randomness of color could be applied specifically to each balloon. Overall, this assignment was highly engaging, and I feel like I gained a considerable amount of knowledge.