“frenzy jump”
so previously i thought about making an instrument using the serial communication between arduino and p5, that turned out to be very difficult , i could have done it, but unfortunately with the time on hand i could not get it finished on time. therefore, i decided to create a very simple game on p5js that consists of a ball jumping over obstacles, and for the arduino part, i have created a remote switch/button panel that contains the arduino, and 4 different components, but i will use only two. The 4 components are 3 paddle switches, and one button, i will use one paddle switch, and one button to control the ball in the game.
My switch panel controller :
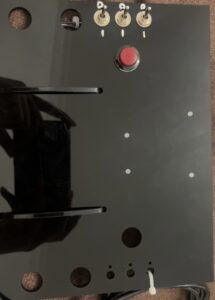
components:
Arduino protoshield
arduino board
3 paddle switches
one button
Initial code :
const floorPosition = 100;
createCanvas(window.innerWidth, window.innerHeight);
player.posY = height - floorPosition - 100;
// Check for serial connection
text("Press space to select serial port.", 20, 30);
text("Connected", 20, 30);
rect(0, height - floorPosition, width, floorPosition);
ellipse(player.posX, player.posY, player.size, player.size);
function drawObstacles() {
rect(o.posX, height - floorPosition, o.width, -o.height);
function testCollisions(x, y) {
// Test collide with floor
if(player.forceY > 0 && y > height - floorPosition - player.size/2) {
if(x + player.size / 2 > o.posX && x - player.size / 2 < o.posX + o.width && y > height - floorPosition - o.height - player.size / 2) {
if(testCollisions(player.posX, player.posY)) {
player.isJumping = false;
if(player.posX < 0 - player.size) {
else if(player.posX < width / 3) {
const nextPos = player.posY + player.forceY;
if(testCollisions(player.posX, nextPos)) {
player.isJumping = false;
player.posY += player.forceY;
player.forceY += gravity;
function moveObstacles() {
o.posX = random(width, width*1.5);
o.width = random(50, 200);
o.height = random(20, 200);
function readSerial(data) {
const pin = data.split(':')[0];
const action = data.split(':')[1];
if(action == "pressed") {
if(key == 'z' && !player.isJumping) {
const player = {
posX: 0,
posY: 0,
size: 50,
forceY: 0,
isJumping: false
}
const obstacles = [{
posX: 600,
height: 50,
width: 70
},{
posX: 1800,
height: 50,
width: 70
},{
posX: 2500,
height: 50,
width: 70
}];
const gravity = 0.3;
const mapSpeed = 5;
const floorPosition = 100;
let gameover = false;
function setup() {
createCanvas(window.innerWidth, window.innerHeight);
player.posX = width / 3;
player.posY = height - floorPosition - 100;
}
function draw() {
background(0);
if(!gameover) {
// Check for serial connection
if(!serialActive) {
fill(255);
text("Press space to select serial port.", 20, 30);
}
else {
fill(255);
text("Connected", 20, 30);
}
// Draw game
drawFloor();
drawObstacles();
drawPlayer();
// Move game
movePlayer();
moveObstacles();
}
}
function drawFloor() {
fill(255);
rect(0, height - floorPosition, width, floorPosition);
}
function drawPlayer() {
fill(255, 150, 0);
ellipse(player.posX, player.posY, player.size, player.size);
}
function drawObstacles() {
fill(255);
for(o of obstacles) {
rect(o.posX, height - floorPosition, o.width, -o.height);
}
}
function testCollisions(x, y) {
// Test collide with floor
if(player.forceY > 0 && y > height - floorPosition - player.size/2) {
return true;
}
for(o of obstacles) {
if(x + player.size / 2 > o.posX && x - player.size / 2 < o.posX + o.width && y > height - floorPosition - o.height - player.size / 2) {
return true;
}
}
return false;
}
function movePlayer() {
if(testCollisions(player.posX, player.posY)) {
player.posX -= mapSpeed;
player.isJumping = false;
if(player.posX < 0 - player.size) {
// gameover
gameover = true;
}
}
else if(player.posX < width / 3) {
player.posX += 0.5;
}
const nextPos = player.posY + player.forceY;
if(testCollisions(player.posX, nextPos)) {
player.forceY = 0;
player.isJumping = false;
}
else {
player.posY += player.forceY;
player.forceY += gravity;
}
}
function moveObstacles() {
for(o of obstacles) {
o.posX -= mapSpeed;
if(o.posX < -o.width) {
o.posX = random(width, width*1.5);
o.width = random(50, 200);
o.height = random(20, 200);
}
}
}
function readSerial(data) {
const pin = data.split(':')[0];
const action = data.split(':')[1];
console.log(action);
if(action == "pressed") {
pressed = true;
}
else {
pressed = false;
}
}
function keyPressed() {
if(key == " ") {
setUpSerial();
}
if(key == 'z' && !player.isJumping) {
player.isJumping = true;
player.forceY = -8;
}
}
const player = {
posX: 0,
posY: 0,
size: 50,
forceY: 0,
isJumping: false
}
const obstacles = [{
posX: 600,
height: 50,
width: 70
},{
posX: 1800,
height: 50,
width: 70
},{
posX: 2500,
height: 50,
width: 70
}];
const gravity = 0.3;
const mapSpeed = 5;
const floorPosition = 100;
let gameover = false;
function setup() {
createCanvas(window.innerWidth, window.innerHeight);
player.posX = width / 3;
player.posY = height - floorPosition - 100;
}
function draw() {
background(0);
if(!gameover) {
// Check for serial connection
if(!serialActive) {
fill(255);
text("Press space to select serial port.", 20, 30);
}
else {
fill(255);
text("Connected", 20, 30);
}
// Draw game
drawFloor();
drawObstacles();
drawPlayer();
// Move game
movePlayer();
moveObstacles();
}
}
function drawFloor() {
fill(255);
rect(0, height - floorPosition, width, floorPosition);
}
function drawPlayer() {
fill(255, 150, 0);
ellipse(player.posX, player.posY, player.size, player.size);
}
function drawObstacles() {
fill(255);
for(o of obstacles) {
rect(o.posX, height - floorPosition, o.width, -o.height);
}
}
function testCollisions(x, y) {
// Test collide with floor
if(player.forceY > 0 && y > height - floorPosition - player.size/2) {
return true;
}
for(o of obstacles) {
if(x + player.size / 2 > o.posX && x - player.size / 2 < o.posX + o.width && y > height - floorPosition - o.height - player.size / 2) {
return true;
}
}
return false;
}
function movePlayer() {
if(testCollisions(player.posX, player.posY)) {
player.posX -= mapSpeed;
player.isJumping = false;
if(player.posX < 0 - player.size) {
// gameover
gameover = true;
}
}
else if(player.posX < width / 3) {
player.posX += 0.5;
}
const nextPos = player.posY + player.forceY;
if(testCollisions(player.posX, nextPos)) {
player.forceY = 0;
player.isJumping = false;
}
else {
player.posY += player.forceY;
player.forceY += gravity;
}
}
function moveObstacles() {
for(o of obstacles) {
o.posX -= mapSpeed;
if(o.posX < -o.width) {
o.posX = random(width, width*1.5);
o.width = random(50, 200);
o.height = random(20, 200);
}
}
}
function readSerial(data) {
const pin = data.split(':')[0];
const action = data.split(':')[1];
console.log(action);
if(action == "pressed") {
pressed = true;
}
else {
pressed = false;
}
}
function keyPressed() {
if(key == " ") {
setUpSerial();
}
if(key == 'z' && !player.isJumping) {
player.isJumping = true;
player.forceY = -8;
}
}
idea of possibly how the game will look like:
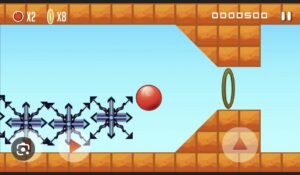