Connectivity:
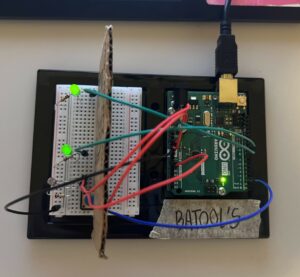
if its not THAT clear from the pic:
- I connected a jumper wire from the ground (GND) rail on the breadboard to the GND (ground) pin on the Arduino.
- I then connected a jumper wire from the 5V rail on the breadboard to the 5V pin on the Arduino.
- I then connect another jumper wire from the 5V rail on the breadboard to the row where the side of the photocell connected to 5V is placed.
- Finally I used a jumper wire to connect the other side of the photocell to the same row as the 10k-ohm resistor’s other end.
Code:
const int led1Pin = 8; // Define a constant integer variable for the pin of the first LED.
const int led2Pin = 9; // Define a constant integer variable for the pin of the second LED.
const int buttonPin = 2; // Define a constant integer variable for the pin of the tactile button.
const int photocellPin = A0; // Define a constant integer variable for the pin of the photocell sensor.
int led1State = LOW; // Initialize an integer variable to store the state of the first LED as LOW.
int led2State = LOW; // Initialize an integer variable to store the state of the second LED as LOW.
int buttonState = LOW; // Initialize an integer variable to store the state of the tactile button as LOW.
int lastButtonState = LOW; // Initialize an integer variable to store the previous state of the button as LOW.
int lightValue = 0; // Initialize an integer variable to store the light reading from the photocell.
int threshold = 500; // Set a threshold value for the light reading. You can adjust this value based on your environment.
void setup() {
pinMode(led1Pin, OUTPUT); // Set the pin of the first LED as an OUTPUT.
pinMode(led2Pin, OUTPUT); // Set the pin of the second LED as an OUTPUT.
pinMode(buttonPin, INPUT_PULLUP); // Set the pin of the tactile button as an INPUT with internal pull-up resistor.
pinMode(photocellPin, INPUT); // Set the pin of the photocell sensor as an INPUT.
}
void loop() {
lightValue = analogRead(photocellPin); // Read the analog value from the photocell sensor.
// Check the photocell value to determine whether it's dark or light.
if (lightValue < threshold) {
// It's dark, turn both LEDs on.
digitalWrite(led1Pin, HIGH); // Turn on the first LED.
digitalWrite(led2Pin, HIGH); // Turn on the second LED.
} else {
// It's light, turn both LEDs off.
digitalWrite(led1Pin, LOW); // Turn off the first LED.
digitalWrite(led2Pin, LOW); // Turn off the second LED.
}
buttonState = digitalRead(buttonPin); // Read the state of the tactile button.
if (buttonState != lastButtonState) {
if (buttonState == LOW) {
// Button pressed, toggle LED states based on previous states.
if (led1State == LOW && led2State == LOW) {
led1State = HIGH;
} else if (led1State == HIGH && led2State == LOW) {
led2State = HIGH;
led1State = LOW;
} else {
led2State = LOW;
}
digitalWrite(led1Pin, led1State); // Set the state of the first LED.
digitalWrite(led2Pin, led2State); // Set the state of the second LED.
}
delay(50); // Introduce a debounce delay to prevent rapid button presses.
}
lastButtonState = buttonState; // Store the current button state for comparison in the next loop iteration.
}
reflection:
This might not have been a supper Idea, However I faced a little creativity block this week that took me a hard time to set a side