For my midterm project, I decided to change my initial idea and created a unique card matching game “Paws and Puzzles”. The game revolves around Simba (named after my own dog<3), a lovable dog who has scattered his toys in the park and needs players’ assistance to match these toys through a card game. The objective is to pair up cards that represent Simba’s toys, including items like balls, a rubber duck, a squeaky chicken and more. The twist is that players must complete the matching within 30 attempts; otherwise, Simba becomes sad, leading to a game over scenario. Paws and Puzzles is not just about matching; it’s wrapped in a delightful storyline, making it engaging and fun.
Here are a few images of the cards I designed:
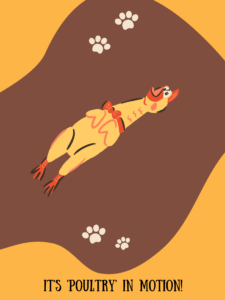
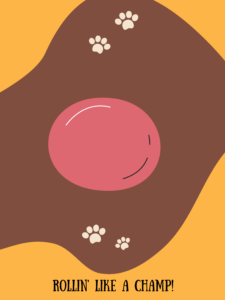
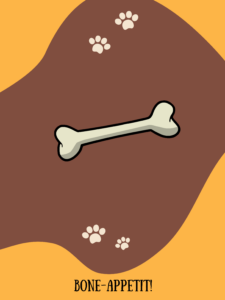
How It Works:
In “Paws and Puzzles,” the game’s core mechanics involve flipping and matching cards. It starts with a visually appealing menu page, and players can choose between starting the game or viewing instructions. There’s also a win condition, and the restart functionality is in place, enhancing the overall user experience. Additionally, the integration of audio elements specific to each scene complements the game, making it more engaging for players.
What I’m particularly proud of is the game’s visual design, which includes amusing captions for the cards, adding an enjoyable element to the gameplay. I’m especially happy with the mousePressed function and the card-matching logic in my code. It did take some time, but I got them working just the way I wanted.
function mousePressed() { if (!gameStarted) { if (instructionMode) { // Clicked on "Start Game" button on instructions page const startGameButtonX = 31; const startGameButtonY = 598; const buttonWidth = 150; const buttonHeight = 50; if (mouseX > startGameButtonX && mouseX < startGameButtonX + buttonWidth && mouseY > startGameButtonY && mouseY < startGameButtonY + buttonHeight) { gameStarted = true; initializeCards(); } }else { const startButtonX = 222; const startButtonY = 235; const buttonWidth = 150; const buttonHeight = 50; if (mouseX > startButtonX && mouseX < startButtonX + buttonWidth && mouseY > startButtonY && mouseY < startButtonY + buttonHeight) { //clicked on start game button gameStarted = true; initializeCards(); } else if (mouseX > startButtonX && mouseX < startButtonX + buttonWidth && mouseY > startButtonY + buttonHeight + 20 && mouseY < startButtonY + buttonHeight + 20 + buttonHeight) { //clicked on instructions button instructionMode = !instructionMode; } } } else { for (let card of cards) { if (card.contains(mouseX, mouseY) && !card.isFlipped() && flippedCards.length < 2) { card.setFlipped(true); flippedCards.push(card); audioCard.play(); if (flippedCards.length === 2) { tries++; //increment the tries if (millis() - startTime >= flipDelay) { checkMatch(); startTime = millis(); //reset the timer } } } } //restart button click on game win if (gameWon && mouseX >= 210 && mouseX <= 420 && mouseY >= 297 && mouseY <= 347) { restartGame(); } //restart button click on the loss if (tries > 30 && mouseX >= width / 2 - 75 && mouseX <= width / 2 + 75 && mouseY >= height - 100 && mouseY <= height - 50) { restartGame(); } } } function checkMatch() { //check if flipped cards match if (flippedCards.length === 2) { if (flippedCards[0].getValue() === flippedCards[1].getValue()) { score++; flippedCards = []; } else { //not a match, unflip the cards after a delay setTimeout(function () { flippedCards[0].setFlipped(false); flippedCards[1].setFlipped(false); flippedCards = []; }, flipDelay); } //checking win condition if (score === cards.length / 2) { gameWon = true; audioGame.stop(); audioWin.play(); //lose game if more than 30 tries } else if (tries > 30) { gameWon = false; audioGame.stop(); audioLose.loop(); } } }
Challenges Faced and Areas for Improvement:
I encountered some challenges, notably a performance issue on the Safari browser. Although I tried various online solutions, none seemed to work, and I eventually opted to playing the game on Chrome for better performance. An area for improvement is adding more levels to the game to increase the difficulty and provide players with a sense of progression. And, of course, there were a couple of bugs that I had to wrestle with – but that’s all part of the coding “fun”, right? Creating this game was a lot of fun, and I hope that people enjoy playing it just as much:)