Concept and Approach:
I have always been fascinated by spirals. I remember books on ‘mental illusions’ that would often consist of spirals that looked procedurally generated, but could seemingly trick you into believing anything. My work for this week is inspired by such spirals – but I wanted to go beyond the procedurally generated symmetries and explore combining movement, elements of randomness, and interactivity with such spirals.
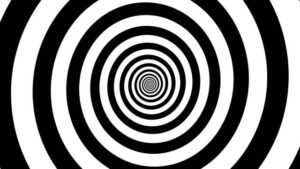
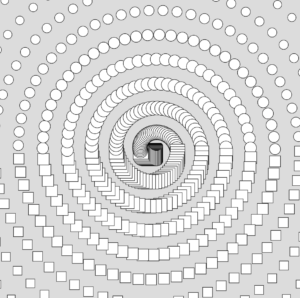
The code design and the movement itself were pretty simple. Early on, I decided to have my spirals be made of circles and squares that would move. Later, I would randomly vary whether the next generated shape was a circle or square, and the size/color of each circle/square. I used Perlin Noise for colors so they would change relatively smoothly. Later, I also added spirals to the four corners of my canvas and made their colors change based on mouse clicks.
Still, after all this, perhaps one of the best decisions I took was making sure I kept my editable parameters as global variables which could be changed easily. Once I had my spiral effect in place, I started tweaking the ‘distance’ and ‘increment’ variables that tweaked the shape of my spiral – and chanced upon an artwork which I found even more satisfying than my initial vision!
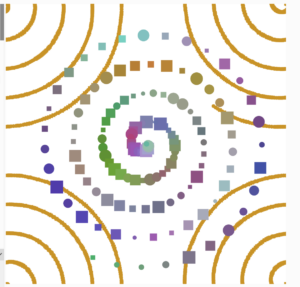
Highlights:
My favorite part of the code has to be the actual calculation for having the shapes move in a spiral. It’s actually based on simple math. To be precise, when covering an angle from 0 to theta across a circle. The new coordinates will be center_x+radius*cos(theta), center_y-radius*sin(theta). Moreover, since it’s a spiral we also increase the radius constantly.
for (let i = 0; i < shapes.length; i++) { let shape = shapes[i]; offset+=0.1 let x = shape.r * cos(shape.theta); let y = shape.r * sin(shape.theta); fill(shape.redval,shape.greenval,shape.blueval) if (shape.isCircle) { ellipse(x, y, shape.size); } else { rect(x, y, shape.size, shape.size); } // Update position shape.theta += increment; shape.r += distance; shape.r+=noise(offset)*0.1; }
Reflections and Future Work:
I think this project has a lot of scope for improvement. Firstly, the actual code is just the same loops duplicated multiple times. The code thus lacks any sort of modularization. This can be improved relatively simply and will enhance the readability and editability of the code by several times.
Second, there are several other interactive elements that can be added to the project. For example, the loop may get tough to look at for a longer period of time – so, a mouse click may be used to stop all the motion.
Lastly, even though my theme for this week’s assignment was ‘chaos’ I believe I can still improve this project’s aesthetics significantly, if I spend more time with it!