by Ajla and Hana
Concept
This week’s assignment was to create a simple musical instrument using the Arduino. Hana and Ajla decided to create a simplified piano and drum. In order to do so, we utilized switches and a servo motor. We controlled the speed of the servo motor using a potentiometer. The notes are played by pressing the buttons. The buttons stop producing noise when released.
Implementation
To implement our musical instrument, we used a potentiometer, switches, a piezoelectric buzzer, resistors, wires and an Arduino. By connecting the switches in parallel we were able to preserve voltage across all components. Even though it was possible to connect everything on one breadboard, we decided to separate it in order to make the instrument user friendly. Furthermore, since many digital inputs were used, the separation ensured that there would not be any overcrowding while presenting the final product.The sketches below show both the two Arduino setup we used and one Arduino setup.
The code was written for both Arduino. Hana wrote the code for the switches and Ajla wrote the code for the drum. The drum was made using a metal screw, silk ribbon and tape which was attached to the servo hand. By pressing the four switches, notes, stored in an array, were played. The drum hit a piece of cardboard in order to produce noise.
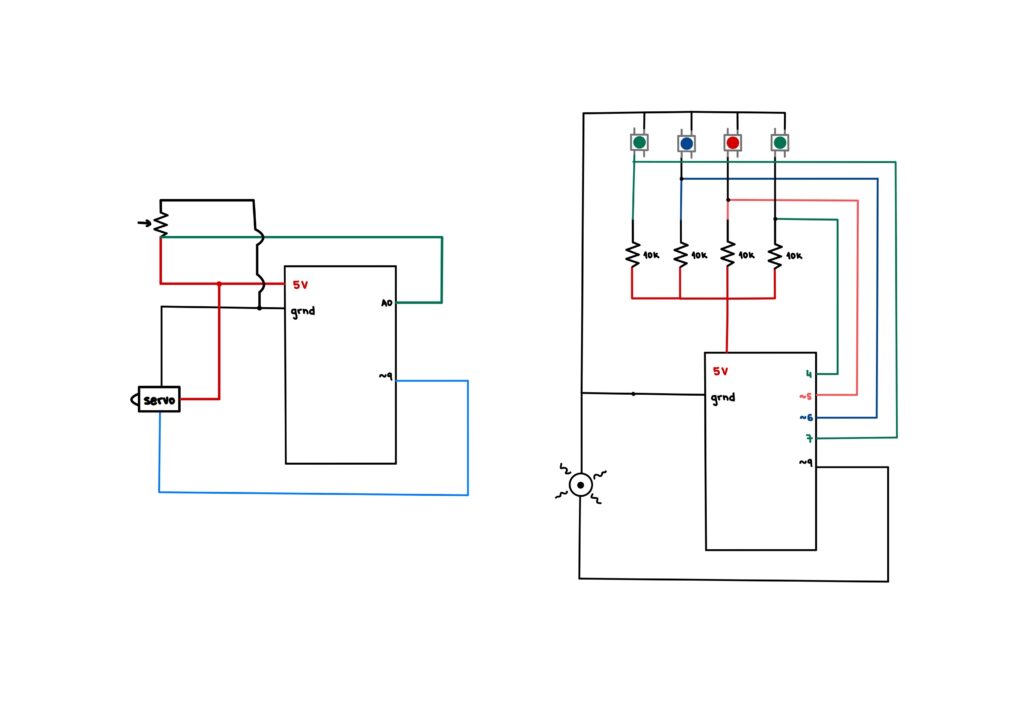
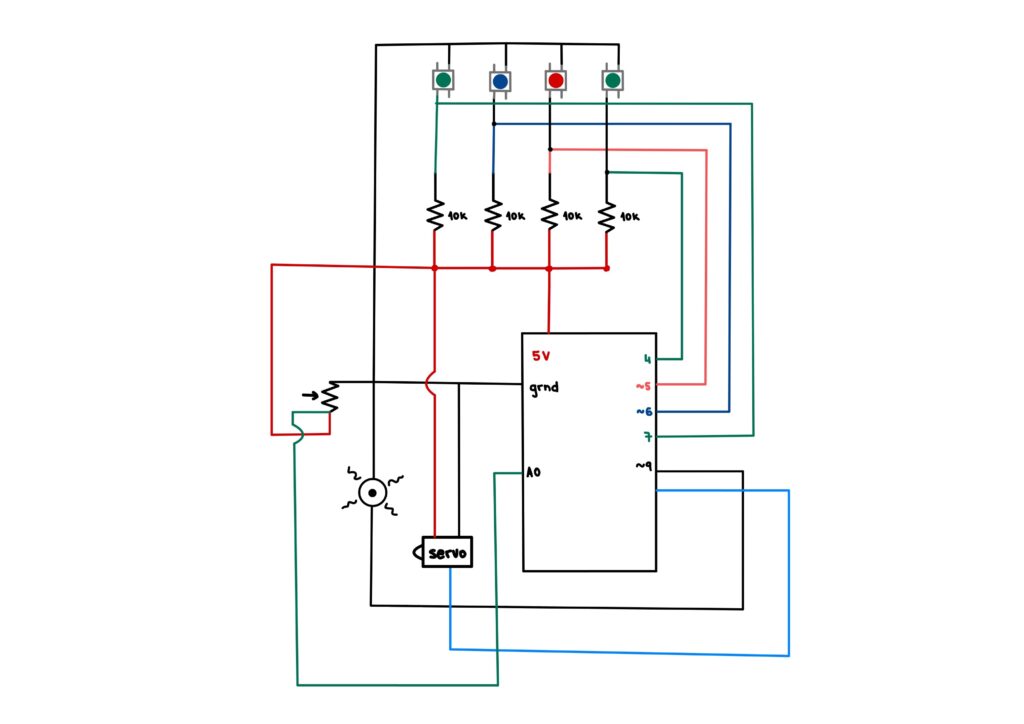
Here is the code for playing the notes:
// if button1 is pressed, playes first note if(button1State==1) { tone(9, notes[0]); } // if button2 is pressed, playes second note if(button2State==1) { tone(9, notes[1]); } // if button3 is pressed, playes third note if(button3State==1) { tone(9, notes[2]); } // if button4 is pressed, playes fourth note if(button4State==1) { tone(9, notes[3]); } // if buttons are release, no note is played if(button1State==0 && button2State==0 && button3State==0 && button4State==0) { noTone(9); } delay(1); // delay in between reads for stability
Here is the code that ran the servo in a 90 degree range:
void loop() { int p_value = analogRead(p_meter); int value = map(p_value, 0, 1024, 10, 20); for (int i=45; i<90; i += value) { pos1 = 0 + (i*2); // servo1 from 0 to 90 degrees myservo1.write(pos1); // move the servo delay(50); } for (int i=45; i<90; i += value) { pos1 = 90 - i*2; // servo1 from 90 to 0 degrees myservo1.write(pos1); // move the servo delay(50); } }
Challenges
The assignment came with a few challenges. Firstly, since the piezoelectric buzzer cannot take multiple inputs at once, it was important to play each note once, without pressing others since that would destroy the quality of the sound. Secondly, the notes play continuously if the button is pressed, even once. That is why we added the last if statement that checks whether all buttons are not pressed, in which case it plays no sound. Thirdly, the servo is quite tricky to use at high speeds. It gets more imprecise as the speed goes up. So, controlling it with the potentiometer was quite hard to do precisely. Lastly, since the angle of motion changes as the speed increases, it was hard to mount the servo on anything as it would immediately fall down once the speed was changed. This became quite frustrating that we decided to just hold the servo in the end to showcase the tempo of the “drum”. All in all, this assignment came with quite a few challenges. Some of them we tried to attempt and won, such as the notes, but some of them remain to be defeated due to the lack of time and materials we had at the time of making this project.
Reflection (Ajla)
I am not very musically inclined. That is why it was quite hard to come up with any ideas for this project and why I wish the theme was a bit more open. However, I have learned quite a bit by attempting this project. The servo, although precise at low speeds, is something that gave me quite a headache when connected to a potentiometer. Since it rotates only 180 degrees, there is a limited amount of freedom I had while working on it which is something I do not like as a Computer Science major. The code could not make up for its shortcomings and I became quite frustrated because of that. However, it is important to learn from such experiences and try again in the future. Although I finished this assignment, I plan to work with theservo more in the future such as to learn how to make up for the drawbacks of its hardware.