This is a fun instrument that lets you play simple tunes by waving your hands in the air!!
The basic idea is to be able to generate different frequencies of sound based on the position of your hand, which is sensed using an ultra-sonic sensor.
For this to work, the sensor has to be an open space such that there are no obstacles in front of it for at least a meter. Place your palm or any other obstacle in front of the sensor to obtain varying frequencies.
Schematics using Fritzing:
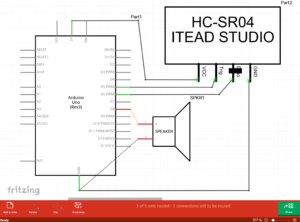
Here’s the code that makes it all work:
long duration; // variable for the duration of sound wave travel
int distance; // variable for the distance measurement
int tim=200;// duration for which one sound pulse will last
pinMode(trigPin, OUTPUT); // Sets the trigPin as an OUTPUT
pinMode(echoPin, INPUT); // Sets the echoPin as an INPUT
pinMode(buzzerPin, OUTPUT);
//Finding distance of obstacle using ultrasonic sensor
digitalWrite(trigPin, LOW);
digitalWrite(trigPin, HIGH);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH); // Reads the echoPin, returns the sound wave travel time in microseconds
distance = duration * 0.034 / 2; // Distance = time*speed/2
//Emitting sound as per the distance
tones =20+distance*5; //calculating the frequency that has to be emitted
tone(buzzerPin, tones, tim); //emits sound at the specified frequency and for the specified duration
delay (space); //delay between each beat
#define echoPin 6
#define trigPin 5
long duration; // variable for the duration of sound wave travel
int distance; // variable for the distance measurement
int tones;
int tim=200;// duration for which one sound pulse will last
int buzzerPin = 8;
int space =500;
void setup() {
pinMode(trigPin, OUTPUT); // Sets the trigPin as an OUTPUT
pinMode(echoPin, INPUT); // Sets the echoPin as an INPUT
pinMode(buzzerPin, OUTPUT);
}
void loop() {
//Finding distance of obstacle using ultrasonic sensor
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH); // Reads the echoPin, returns the sound wave travel time in microseconds
distance = duration * 0.034 / 2; // Distance = time*speed/2
//Emitting sound as per the distance
tones =20+distance*5; //calculating the frequency that has to be emitted
tone(buzzerPin, tones, tim); //emits sound at the specified frequency and for the specified duration
delay (space); //delay between each beat
}
#define echoPin 6
#define trigPin 5
long duration; // variable for the duration of sound wave travel
int distance; // variable for the distance measurement
int tones;
int tim=200;// duration for which one sound pulse will last
int buzzerPin = 8;
int space =500;
void setup() {
pinMode(trigPin, OUTPUT); // Sets the trigPin as an OUTPUT
pinMode(echoPin, INPUT); // Sets the echoPin as an INPUT
pinMode(buzzerPin, OUTPUT);
}
void loop() {
//Finding distance of obstacle using ultrasonic sensor
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH); // Reads the echoPin, returns the sound wave travel time in microseconds
distance = duration * 0.034 / 2; // Distance = time*speed/2
//Emitting sound as per the distance
tones =20+distance*5; //calculating the frequency that has to be emitted
tone(buzzerPin, tones, tim); //emits sound at the specified frequency and for the specified duration
delay (space); //delay between each beat
}
Improvements:
In the given code the sounds are emitted at a fixed time interval for a fixed duration. We can play around with this by changing the value of the variables ‘tim’ and ‘space’. Also, we can play around with the way the frequency is calculated from the distance.