This assignment seemed similar to the previous one except how I approached the problem. The goal is to generate art Through Object Oriented Programming (OOP). After seeing an art in Eyeo2012 – Casey Reas video, I wanted to create something similar to the the below image:
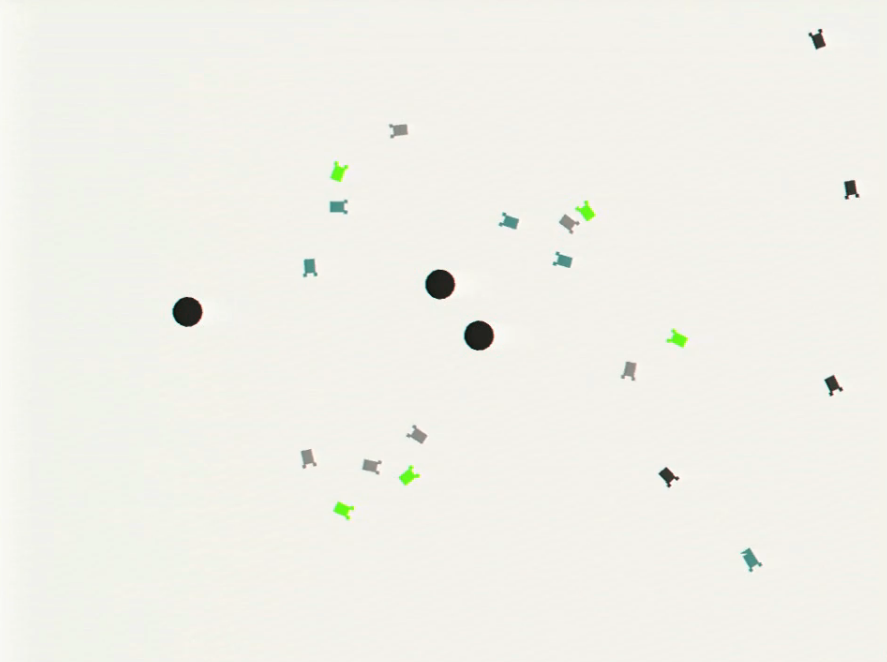
Small, individual creature-like objects moving around black dots. This looked interesting to work on. So, initially, I thought about placing food, creatures, and their predators. But while simulating how my code will work, I realized that it will require a lot of resources(avoiding collisions, having a proper line of sight, etc.) to populate even hundreds of creatures. So, instead of having creature-like objects, I decided to make something simpler: objects that follow gravity.
The above is done as a class work. However, it does not seem like a generative art because it looks too vacant. Also, I do not want the center of gravity to shoot away objects. So I worked on details.
Better. There are 2 major changes other than fancy colors. First, there are tails to the moving objects. As objects move, they leave traces of their previous positions. This is done by a simple trick: instead of overwriting the board with a solid color, use a color with high transparency. The below is the code.
background('rgba(0,0,0, 0.08)');
Second, when particles move too close to the center (and therefore are at risk of being launched back in high velocity), they become reinitialized through init() function. The function is basically a constructor but has a callable name. The below shows how it is done.
constructor() { this.init(); } init() { this.size = random(2,8); // random size this.vx = random(-3,3); // initial x velocity this.vy = random(-3,3); // initial y velocity this.x=this.size/2 + random(width-this.size); // initial x position this.y=this.size/2 + random(height-this.size); // initial y position this.px=this.x; this.py=this.y; // previous x and y (for smoothing shapes) } ... if (d<30) {this.init();} ...
While working on the above code, I worked on another branch of the project in parallel. Its features include mouse position being the center of gravity and changing the direction of gravity (positive and negative gravity). The sketch is created for the purpose of practice (thus labeled “PR”), so the code is a bit messy and commented not-so-greatly.
Wait… This reminds me of a firework…
Inspired by what was seen in the above sketch, I created a firework as a side project (“PR”actice). In a big picture, it operates the same way as the gravity function. A circle (point of negative gravity) moves toward the center, and when it reaches its maximum height, 500 colorful circles get teleported around the circle and get pushed away. For more realistic visuals, particles follow gravity toward the floor and their size become smaller as they “burn” over time.
I know. There are too many sketches in one post. I promise to finish my post with one last thing, the generative art. Now that I think of it, it has much less code and complexity compared to the firework, but it does what it needs to do. It should be alright. It is very similar to the second sketch, with slight modifications such as smaller particles and having no velocity other than toward the center.
The biggest problem I encountered during this project was not the difficulties in coding, but headaches I got from my own artwork. Before realizing it was a terrible idea (I just realized as I write this post), I made my generative artwork colorful, which looked like the below.
Click the canvas to see colored version.
Imagine seeing thousands of these colorful moving dots and fine-tuning parameters and functions for hours. Definitely a terrible idea. Now I learned that more color does not mean a better art. It was a costly lesson. I hope other people to not make the same mistake as I did this time.