Concept—
Outline:
For my first assignment, I utilized the coding information we learned about in class to use simple shapes to create a self-portrait. I used ellipses, circles, squares, rectangles, lines, arcs, and triangles to create an image that looks like a cartoonish version of myself, while also recoloring specific parts, modifying the stroke weight, and removing the stroke completely for some elements of my portrait.
Inspiration:
The inspiration for my self-portrait was based on the animated characters from the game Animal Crossing. I borrowed elements from the human characters’ physical appearance, notably the reddish-orange triangle-shaped nose, the white glimmer in the pupils, and the circular blush under the eyes for a ‘cutesy’ effect:
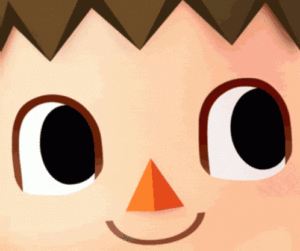
Code highlight—
I made sure to section the different elements of my portrait in order to easily locate them in case I wanted to tweak something specific in one of the features. My favorite lines of code were the ones I used to make the eyes because I made them look cartoonish using the white glimmer + they were a bit more detailed in comparison to the other elements of my portrait as I added a code for the eye movement:
// movement of eyes -- used this tutorial to figure it out https://youtu.be/dcxmJtioamg?t=1033 // right and left let e_x1 = map(mouseX, 0, 200, 148, 150) let e_x2 = map(mouseX, 0, 200, 248, 250) let e_x3 = map(mouseX, 0, 200, 148, 150) let e_x4 = map(mouseX, 0, 200, 248, 250) // up and down let e_y1 = map(mouseX, 0, 200, 183, 185) let e_y2 = map(mouseX, 0, 200, 183, 185) let e_y3 = map(mouseX, 0, 200, 183, 185) let e_y4 = map(mouseX, 0, 200, 183, 185) // eyes ellipseMode(CENTER); stroke (200) // color of white eyes' outline strokeWeight (2) fill (255, 255, 255) ellipse(150, 185, 40, 40) // (x1, y1, width, height) ellipse(250,185, 40, 40) fill (122, 65, 44) stroke (110, 60, 42) // colour of brown pupils' outline strokeWeight (2) ellipse(e_x3, e_y3, 20, 20) // colored right pupil ellipse(e_x4,e_y4, 20, 20) // colored left pupil fill (0, 0, 0) stroke(0) // color of stroke/ outline (color of glasses) strokeWeight (2) ellipse(e_x1, e_y1, 8, 8) // right pupil ellipse(e_x2,e_y2, 8, 8) // left pupil stroke (180) // color of white eyes' outline strokeWeight (2) fill (255, 255, 255) ellipse(158, 180, 6, 6) // right white glow ellipse(258, 180, 6, 6) // left white glow // x: right or left, y: up or down
Embedded sketch—
(p.s. the pupils move with a very limited range of movement)
Reflections and improvements—
I struggled with a few things when trying to design my portrait. This includes:
- Trying to get the noStroke effect to work without removing the strokes on every shape. I tried to remove the strokes off of every shape in my image except the glasses and eyebrows, but even after I put the noStroke code after where I wrote the code for the eyebrows and glasses, it still removed their stroke. I realized later that I had to specify the stroke color as well as the strokeWeight for the glasses and eyebrows before I could put the noStroke effect for anything underneath. I then realized I liked the strokes surrounding the shapes, just not in black—hence why most of the features in my portrait are outlined with a darker shade of their respective color rather than black.
- Forgetting to center the rectangle shapes for the glasses—which made it take much longer because I had to figure out where to plot the coordinates. This also caused a domino effect because if I had to type another code for a rectangle element below the code for the glasses and tried to center it, it would also center the glasses and mess up their coordinates completely.
- Not having many shapes to work with. I felt very limited by the shapes, and in some cases, I wanted a semi-circle (like for the mouth), but I didn’t know the code for it. I had to look up a video on how to draw an arc, and this solved my problem—until I realized a 180° arc doesn’t have an outline on top. This meant that I had to place an outlined ellipse underneath the arc to make the mouth look complete and fully outlined (ref. to mouth section of code).
- Trying to figure out how to give the pupils a full range of movement. I only figured out how to move them diagonally through a YouTube video.
Nicely done. For mapping the eye movement to a larger range you can use the builtin variables *width* and *height* in your call to map. Instead of mapping the mouse position in your fixed range of 0,200 you could do e.g. 0,width