Building up on the progress I posted a while ago, I am happy the process went smoothly. I did face a few minor issues but the help from professor Aaron and Jack, aided me in getting through them.
Issues and solutions:
First of all an initial issue that I had regarding the game was that I wasn’t able to fix the issue that the thief was above the darkness. Initially I was using load pixels/ image processing with pixels which did make the flashlight effect, but after professor Aaron’s help, the conclusion that in order to make the thief behind the darkness as well I had to switch to using a PGraphic object was reached. I drew the nyu background and the thief image into the pgraphic object and so I can access the pixels of that pgraphic object in the for loops, instead of just the background image.
void theGame(){ black = createGraphics(1200, 800); black.beginDraw(); black.background(0); black.endDraw(); mask = createGraphics(1200, 800); mask.beginDraw(); mask.background(0); mask.endDraw(); pg=createGraphics(1200,800); pg.beginDraw(); pg.image(nyu,0,0); pg.image(thief,x,y); if(dist(x,y,mouseX,mouseY)<100){ if(mousePressed==true){ gameState="WIN"; file.stop(); if(!sound.isPlaying()); sound.play(); } } pg.endDraw(); mask.beginDraw(); mask.background(0); mask.noStroke(); mask.fill(255); mask.ellipse(mouseX, mouseY, 200, 200); mask.endDraw(); pg.mask(mask);
Another issue I faced was that using keyPressed after game over to return to the play mode did not work as I had to keep pressing the key, hence in order to fix this I created a new function “reset game”, which helped fix this issue as well as replay the sounds and the reset the time back to countdown from 5.
void resetGame(){ if (keyPressed==true){ gameState="PLAY"; file.loop(); laugh.stop(); sound.stop(); timeLeft=6; }
Further development:
Developments I mad since the progress post are extensive. First of all I added the start page with instructions and the “win” or “lose” pages as well, with the transitions from each one to the other.
Intro page:
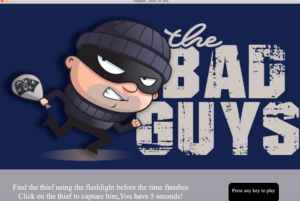
Win page:
Game Over page: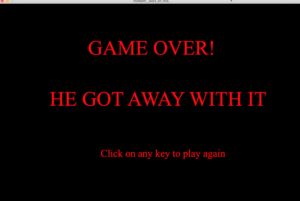
I also made the thief image appear at random locations every time a new game is played, and included the mouse pressed event for the player to be able to press on the thief and win.
Finalizing minor details:
In my opinion the finer details brought the whole project together. The sound effects of the thief laughing when you lose the game as well as the alarm sirens makes the game more interesting. The timer makes the suspenseful mood , making the game much more fun to play. Adding the timer was bit confusing but with the help of this youtube video I was able to do it (https://www.youtube.com/watch?v=JXqukW44Dhs&t=813s) .
The sounds I used were gotten from (https://freesound.org/)
Final Result:
This is the zip code if anyone wants to try out the game.
Code:
import processing.sound.*; SoundFile file; SoundFile sound; SoundFile laugh; PImage nyu; PImage thief; PImage introPic; PImage winningPic; PImage gameOver; PImage image; float r1 ; float r2 ; int buttonX, buttonY; int buttonWidth, buttonHeight; color buttonColor, baseColor; //colors of play button PFont f; PGraphics pg; PGraphics black, mask; String gameState; float x; float y; Timer countdownTimer; int timeLeft; void setup() { size(1200, 800); gameState=("START"); PFont.list(); f = createFont("Times New Roman",75); x = random(-20, 1100); y = random(-20, 600); nyu=loadImage("nyu.png"); thief=loadImage("thief.png"); buttonColor = color(0); baseColor = color(102); buttonWidth = 200; buttonHeight = 80; buttonX = width/2+300; buttonY = 3*height/4 + 100; countdownTimer=new Timer(1000); timeLeft=6; file= new SoundFile(this,"theme.mp3"); sound= new SoundFile(this,"alarm.wav"); laugh= new SoundFile(this,"evil laugh.wav"); } void draw() { background(0); if (gameState=="START"){ introScreen(); if (keyPressed==true){ file.loop(); gameState="PLAY"; //transition to play state if key pressed countdownTimer.start(); } } else if (gameState=="PLAY"){ theGame(); } else if (gameState=="WIN"){ congratsScreen(); if (keyPressed==true){ gameState="PLAY"; resetGame(); x = random(-20, 1100); y = random(-20, 600); } } else if (gameState=="LOSE"){ gameOver(); resetGame(); x = random(-20, 1100); y = random(-20, 600); } }
//code for the actual game void theGame(){ //pgraphics black = createGraphics(1200, 800); black.beginDraw(); black.background(0); black.endDraw(); mask = createGraphics(1200, 800); mask.beginDraw(); mask.background(0); mask.endDraw(); pg=createGraphics(1200,800); pg.beginDraw(); //code for allowing the players to click on the thief image pg.image(nyu,0,0); pg.image(thief,x,y); if(dist(x,y,mouseX,mouseY)<100){ if(mousePressed==true){ gameState="WIN"; //if the player presses on the image the player wins file.stop(); if(!sound.isPlaying()); sound.play(); } } pg.endDraw(); mask.beginDraw(); mask.background(0); mask.noStroke(); mask.fill(255); mask.ellipse(mouseX, mouseY, 200, 200); mask.endDraw(); pg.mask(mask); image(black,0,0); image(pg, 0, 0); //countdown timer logic if (countdownTimer.complete()==true){ if(timeLeft>1){ timeLeft--; countdownTimer.start(); }else{ gameState="LOSE"; //code for if the timer finishes the player loses file.stop(); sound.stop(); if(!laugh.isPlaying()); laugh.play(); } } //showing the timer String s="Time Left:"+timeLeft; textAlign(LEFT); textSize(20); fill(255,0,0); text(s,20,100); }
//code for the start/intro screen void introScreen(){ background(157,157,165); fill(buttonColor); imageMode(CORNERS); introPic=loadImage("introPic.png"); image(introPic, 0,0,1200, 650); stroke(255); rect(buttonX, buttonY, buttonWidth, buttonHeight, 20); //key pressed play button textFont(f,25); textAlign(CENTER, CENTER); fill(255); textSize(20); String play = "Press any key to play"; //text in play button to clarify it text(play, buttonX+100 , buttonY+30); textSize(30); String instructions="Find the thief using the flashlight before the time finishes"; text(instructions, buttonX-500 , buttonY+15); String instructionsCont="Click on the thief to capture him,You have 5 seconds!"; text(instructionsCont,buttonX-500 , buttonY+50); }
//code for the congrats screen when the player wins void congratsScreen(){ winningPic=loadImage("busted2.png"); image(winningPic, 0,height/5,1200,800); textFont(f,40); textAlign(CENTER, CENTER); fill(255); textSize(40); String congrats = "Congrats You Busted Him!"; text(congrats, width/2,80); String replay = "Click any key to play again"; text(replay, width/2,130); }
//code for the gameover screen when a player loses void gameOver(){ background(0); textSize(80); String gameover = "GAME OVER!"; String gameovercont="HE GOT AWAY WITH IT"; text(gameover, width/2-250,height/4); text(gameovercont, width/2-400,height/2); textSize(40); String replay = "Click on any key to play again"; text(replay, width/2-200,height/2+200); }
//code to reset the game, along with the sound and the timer void resetGame(){ if (keyPressed==true){ gameState="PLAY"; file.loop(); laugh.stop(); sound.stop(); timeLeft=6; } }