For this week, I created an art piece using primitive Processing functions and loops that we have learned in class so far. Putting all this into practice, I decided to implement a digital carpet design. Something to look forward to: It changes color 60 times in a second! Now let us look into the design process.
Production Process:
To imitate the carpet look, I went with a 400×600 pixel frame size. Being clueless about the creative process, in the beginning, I tried several designs and struggled with the implementation. So after a couple of hours of crying and disappointment, I started to sketch using pen and paper; ok, digital pen and paper. Here is how it went:
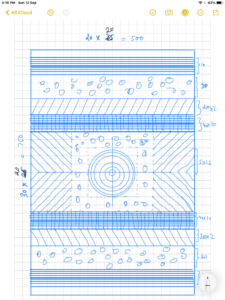
With the design and proportions sketched out, it made the implementation so easy. I started with initializing the variables that would help control the size of everything to keep the design proportional. I am so glad that I was able to not hard-code and still implement the code efficiently. The magic behind, simple maths!
Then, I started working on the different parts of the design, row by row. While the implementation of a particular design in a row was simpler, the desired design was a trial and error process. After writing the code of each line, it was important to check how it displays on the screen and interacts with the existing design. Often times the design would be messed up (see the attached production process images) and then this would require different parts of code to be shifted around to achieve the desired look.
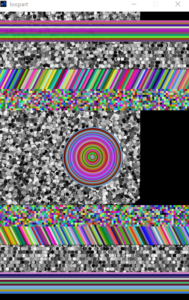
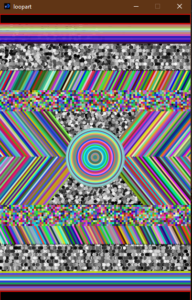
To make the artwork more bright and happening, I made the color of the different parts of the design change but within a color palette. Might sound like something very complex, but random() got the job done. Restricting the values to random() enabled the beautiful color scheme that you can see below. My screen in the video does not do justice to colors, so below is a screenshot also.
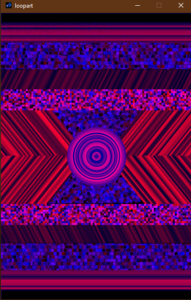
If only I was creative enough, I could have implemented additional design elements into my art. However, I am delighted that I was able to finish this project to the best of my ability.
The final design might not be what I originally had high hopes about, I still believe that I have made significant progress with my design process. I look forward to several other design assignments as an opportunity to improve my designs and production process.
Here is the final code for the design:
//Global variables to keep it proportionate
int boxsize = 20;
int horizontalbox = 20;
int verticalbox = 30;
int circlesize = 7;
void setup(){
size(400,600);
background(0);
}
void draw(){
//Random small Circles in the middle of canvas
for(int x = boxsize*5; x<=boxsize*15; x+=circlesize){
for(int y = boxsize*10; y<=boxsize*20; y+=circlesize){
if(y>=boxsize*10 && y<=boxsize*20 && x>=boxsize*5 && x<=boxsize*15 ){
noStroke();
fill(random(100),0,random(250));
circle(random(x),random(y),circlesize);
}
}
}
//Random circles in 3rd row
for(int x = 0; x<=width; x+=circlesize){
for(int y = 0; y<=height; y+=circlesize){
if(y>=boxsize*3 && y<=boxsize*6){
noStroke();
fill(random(100),0,random(250));
circle(random(x),y,circlesize);
}
}
}
//Random circles in te third last row
for(int x = 0; x<=width; x+=circlesize){
for(int y = 0; y<=height; y+=circlesize){
if(y>=boxsize*24 && y<=boxsize*27){
noStroke();
fill(random(100),0,random(250));
circle(random(x),y,circlesize);
}
}
}
//Line Strokes in 2nd row
for(int i = boxsize; i <= boxsize*3; i+=(boxsize*2)/10){
strokeWeight((boxsize*2)/10);
stroke(random(250),0,75);
line(0,i,width,i);
}
//Line strokes in 2nd last row
for(int i = boxsize*27; i <= boxsize*29; i+=(boxsize*2)/10){
strokeWeight((boxsize*2)/10);
stroke(random(250),0,75);
line(0,i,width,i);
}
stroke(0);
line(0,boxsize*6,width,boxsize*6);
//slanted lines in 4th Row
for(int j = 0; j< width+boxsize; j+=boxsize/5){
strokeWeight(4);
stroke(random(100),0,50);
line(j, boxsize*6, j-boxsize, boxsize*8);
}
stroke(0);
line(0,boxsize*24,width,boxsize*24);
//Slanted lines in 4th last row
for(int j = 0; j< width+boxsize; j+=boxsize/5){
strokeWeight(4);
stroke(random(100),0,50);
line(j-boxsize, boxsize*22, j, boxsize*24);
}
//fills at top and bottom for finish
fill(0);
strokeWeight(3);
rect(0,0,width,boxsize);
rect(0,boxsize*29,width,height);
//slanted lines 6th row , right
for(int j = boxsize*15; j< width+boxsize*4; j+=boxsize/5){
strokeWeight(4);
stroke(random(250),0,50);
line(j, boxsize*10, j-boxsize*4, boxsize*15);
}
//slanted lines 7th row, right
for(int j = width+boxsize*4; j>boxsize*11 ; j-=boxsize/5){
strokeWeight(4);
stroke(random(250),0,50);
line(j, boxsize*15, j+boxsize*4, boxsize*20);
}
//slanted lines 6th row, left
for(int j = boxsize*5; j>-boxsize*5 ; j-=boxsize/5){
strokeWeight(4);
stroke(random(250),0,50);
line(j, boxsize*10, j+boxsize*4, boxsize*15);
}
//slanted lines 7th row, left
for(int j = 0; j< boxsize*9; j+=boxsize/5){
strokeWeight(4);
stroke(random(250),0,50);
line(j, boxsize*15, j-boxsize*4, boxsize*20);
}
//small boxed grid 5th row
for(int x=0; x<=width; x+=(boxsize*2)/10){
for(int y=boxsize*8; y<=boxsize*10; y+=(boxsize*2)/10){
noStroke();
fill(random(250),0,random(250));
rect(x,y,(boxsize*2)/10,(boxsize*2)/10);
}
}
//small boxed grid 8th row
for(int x=0; x<=width; x+=(boxsize*2)/10){
for(int y=boxsize*20; y<=boxsize*22; y+=(boxsize*2)/10){
noStroke();
fill(random(250),0,random(250));
rect(x,y,(boxsize*2)/10,(boxsize*2)/10);
}
}
//big circles in the middle of screen
for(int x=boxsize*6; x>=boxsize/4; x-=boxsize/4){
stroke(random(250),0,150);
circle(width/2,height/2,x);
}
}