No, that is not a typo. Yes, I used a nickname so that my name would have the same number of syllables as ‘Alice’ and this is a pun on Through the Looking Glass, which really would have worked better if there had actually been any classes involved in this assignment but please, cut me (and my long sentences) some slack (Slack, hehe). It’s hard to think of any new puns after the first idea gets stuck in your head, as terrible as it might be.
This week’s assignment was to make a self-portrait using Processing’s basic shape tools and other functions. Being a little bit familiar with Processing, I had thought that this could be done fairly quickly– boy was I wrong. It took me two long sessions to get everything looking a way I was satisfied with. I had underestimated my ability to keep changing things infinitesimally to see what was better, and I had not realised the depth of Processing’s library, never really having worked much with it before.
I was blessed with a lot of tools to make my work easier – for example, I discovered that the rect() function allows you to add angle parameters at the end if you want curved corners. So instead of making a normal rectangle and then an arc below it for the neck, I simply made it a rectangle with very rounded corners, and it helped give my hair shape too (but you can barely see it because it’s hidden behind the shirt). The point() function was perfect for drawing my birthmarks, and the alpha parameter for changing opacity which we discussed in class helped me make my glass lenses look like, well, glass.
When we had been asked to attempt to draw a face in class, I had been making an emoji smiley but had been stumped when it came to the smile. That’s when Professor (I’m sorry, it’s hard to unlearn years of cultural traditions in a week) had talked about the arc() function, and it took me some time to wrap my head around it but I was finally able to figure out how to work it and start incorporating it in my code. This is also a good time to mention that I have copied a lot of variable names and some code verbatim from the face example we had discussed in class, it helped a lot.
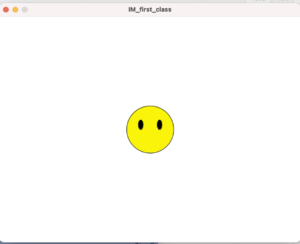
But, now that I had learnt how a lot of functions worked through trial and error, it was time to start making a resemblance of my face. I had been going through a lot of random past students’ blogs from the first day out of excitement for this course, so I had some sort of idea of which features I would like to draw which way: for example I knew I would like to use triangles for my eyebrows instead of arches and an ellipse for my face. But because of these mental images, I never really ended up drawing any physical sketch so the end result was sort of a surprise to me too.
Something I hadn’t seen anyone draw was straight, long hair and I spent a lot of time trying to get it right. Since I usually end up wearing it in a middle-partition, the symmetry part was easy but it required a lot of adjustments and a combination of the rounded rectangle, two quadrilaterals and a circle which rather looks like a halo in the sketch. My face had been looking rather, to put it mildly, pensive, so I knew I had to make a smile somehow, but just drawing an arc didn’t fit in with the level of “realism” I had for the rest of my sketch. I was going to go through a long process of drawing arcs on the top and bottom, but then I realised that an ellipse with a line through it should do the trick too. I had almost given up on drawing my glasses because the eyes section was looking too crowded, but then I thought that I really can’t lie to myself and the world about this, I need those glasses. So I ended up adjusting the eye spacing and going through with them, and I’m glad I did.
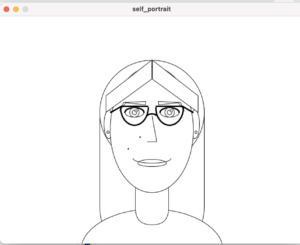
It was still a bit messy, but it had to do. Then I chose one of my favourite shades of aqua for the background and a shade of violet for the top because I’d like to think I’m wearing some NYU merch here, and after using varying strokeWeights for my “hipster” glasses I was done. This is the final code with some of the small details written in comments:
int faceHeight = 265; int faceWidth = 175; int eyeSpacing = 37; int eyeWidth = 40; int eyeHeight = 20; int eyeOffset = 37; int noseOffset = 30; int noseHeight = 25; int noseWidth = 20; float earSpacing = 90.5; int earHeight = 60; int earWidth = 20; int mouthOffset = 65; int x, y; void setup(){ size(640, 480); x = width/2; y = height/2; } void draw(){ background(148, 243, 252); //hair length fill(0); rectMode(CENTER); rect(x, y+noseHeight+40, 220, 350, 80); circle(x, y-eyeOffset, 220); //ears and earrings fill(245, 182, 133); ellipse(x-earSpacing, y-eyeOffset+25, earWidth, earHeight); ellipse(x+earSpacing, y-eyeOffset+25, earWidth, earHeight); fill(227, 238, 240); circle(x-earSpacing-2, y+5, 5); circle(x+earSpacing+2, y+5, 5); //t-shirt fill(71, 1, 147); ellipse(x, y*2+15, 300, 175); //neck fill(245, 182, 133); rectMode(CORNER); rect(x-noseWidth-15, y, noseWidth*2+30, 200, 0, 0, 70, 70); //head ellipse(x, y, faceWidth, faceHeight); strokeWeight(3); //for the moles to be visible point(x-noseWidth-5, y+15); point(x-50, y+40); strokeWeight(1); //hair parting fill(0); quad(x-1, y-faceHeight/2-16, x-1, y-faceHeight/2+25, x-faceWidth/2-10, y-eyeOffset, x-faceWidth/2-10, y-eyeOffset-35); quad(x+1, y-faceHeight/2-16, x+1, y-faceHeight/2+25, x+faceWidth/2+10, y-eyeOffset, x+faceWidth/2+10, y-eyeOffset-35); //eyebrows triangle(x-eyeSpacing+eyeWidth/2+4, y-(eyeOffset+3)-eyeHeight, x-eyeSpacing+eyeWidth/2+3, y-(eyeOffset+3)-eyeHeight/2-3, x-eyeSpacing-eyeWidth/2-3, y-(eyeOffset+3)-eyeHeight/2-8); triangle(x+eyeSpacing-eyeWidth/2-4, y-(eyeOffset+3)-eyeHeight, x+eyeSpacing-eyeWidth/2-3, y-(eyeOffset+3)-eyeHeight/2-3, x+eyeSpacing+eyeWidth/2+3, y-(eyeOffset+3)-eyeHeight/2-8); //discarded idea for the ends of the eyebrows //strokeWeight(2); //line(x-eyeSpacing-eyeWidth/2-3, y-eyeOffset-eyeHeight/2-8, x-eyeSpacing-eyeWidth/2-10, y-eyeOffset-2); //strokeWeight(1); triangle(x-eyeSpacing-eyeWidth/2-3, y-(eyeOffset+3)-eyeHeight/2-8, x-eyeSpacing-eyeWidth/2-10, y-(eyeOffset+3)-2, x-eyeSpacing-eyeWidth/2+2, y-(eyeOffset+3)-eyeHeight/2-8); triangle(x+eyeSpacing+eyeWidth/2+3, y-(eyeOffset+3)-eyeHeight/2-8, x+eyeSpacing+eyeWidth/2+10, y-(eyeOffset+3)-2, x+eyeSpacing+eyeWidth/2-2, y-(eyeOffset+3)-eyeHeight/2-8); //eyes fill(255); ellipse(x-eyeSpacing, y-eyeOffset, eyeWidth, eyeHeight); ellipse(x+eyeSpacing, y-eyeOffset, eyeWidth, eyeHeight); strokeWeight(3); //for the eyelashes arc(x-eyeSpacing, y-eyeOffset, eyeWidth, eyeHeight, PI, TWO_PI); arc(x+eyeSpacing, y-eyeOffset, eyeWidth, eyeHeight, PI, TWO_PI); strokeWeight(1); fill(49,0,7); //iris circle(x-eyeSpacing, y-eyeOffset, 20); circle(x+eyeSpacing, y-eyeOffset, 20); fill(0); //pupil circle(x-eyeSpacing, y-eyeOffset, 10); circle(x+eyeSpacing, y-eyeOffset, 10); fill(255); //highlights circle(x-eyeSpacing+3.5, y-eyeOffset-3, 5); circle(x+eyeSpacing+3.5, y-eyeOffset-3, 5); //nose //discarded original shape //triangle(x, y-noseOffset, x-(noseWidth/2), y+noseHeight, x+(noseWidth/2), y+noseHeight); line(x, y-noseOffset, x+(noseWidth/2), y+noseHeight); line(x-(noseWidth/2), y+noseHeight, x+(noseWidth/2), y+noseHeight); //glasses strokeWeight(3); stroke(103, 22, 22); //stroke(77, 224, 211); the colour of one of my old glasses line(x-noseWidth/2+1, y-eyeOffset-eyeHeight/2-2.5, x-noseWidth-eyeWidth-4, y-eyeOffset-eyeHeight/2-2.5); line(x+noseWidth/2-1, y-eyeOffset-eyeHeight/2-2.5, x+noseWidth+eyeWidth+4, y-eyeOffset-eyeHeight/2-2.5); line(x-noseWidth-eyeWidth-4, y-eyeOffset-eyeHeight/2-2.5, x-earSpacing+4, y-eyeOffset); line(x+noseWidth+eyeWidth+4, y-eyeOffset-eyeHeight/2-2.5, x+earSpacing-4, y-eyeOffset); fill(255, 60); //glass lenses, opacity adjusted arc(x-eyeSpacing, y-eyeOffset-10, eyeWidth+18, eyeWidth+35, 0, PI); arc(x+eyeSpacing, y-eyeOffset-10, eyeWidth+18, eyeWidth+35, 0, PI); noFill(); arc(x, y-noseOffset-1, 17, 5, PI, TWO_PI); strokeWeight(1); stroke(0); //mouth fill(240, 90, 112); ellipse(x, y+mouthOffset+5, noseWidth*2+20, 15); noFill(); arc(x, y+mouthOffset-5, noseWidth*2+40, 20, 0, PI); }
I know a lot of stuff is hard-coded despite me using a lot of variables, but that’s just how it came down to in the end because of trial and error and I would like to change that in the future. This was plain drawing so I didn’t think of using methods for everything either, but maybe I should have.
But voila! Here we have it, the results of this piece of code, a little bad in image quality:
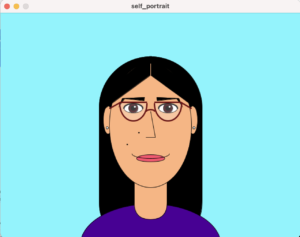
I know it ended up quite simplistic, but I’m content with the small details I worked on. It probably looks like a nicer version of me in real life. And hey, this is the most realistic photo you’re going to get from a photo averse person!
In conclusion, I am so so sorry for making this blog post the ramble it was, and will definitely try to keep it shorter and maybe more succinct in the future. I really don’t know what got into me when I started this. But, I’m looking forward to the future assignments, and hopefully I’ll have figured out how to make WordPress not reduce the quality of photos so much by next time.
For anyone who has made it till the end, I’ll now let y’all go from this post so that your brains can recover and start processing (ba dum tss, sorry I already had this planned when I was in the middle of writing the blog, I’ll actually go now, peace).