Idea
The experiences I had with the LED light in class reminded me of the character of HAL 9000 from the classic film ‘2001: A Space Odyssey’ and so for this week’s project I wanted to do something surrounding that.
After spending quite sometime brainstorming, I decided to use the LEDS and the digital and analog sensors to make an interactive movie wherein the player/audience defeats HAL and sabotages his attempts to conquer the world.
Implementation
In order to implement this idea, I decided to play out a famous dialogue from the movie which HAL says in the climax and convert it into Morse so that the dialogue can be communicated through the LED and that it would appear as if HAL is saying this. And then in order to show that HAL is attempting to destroy the world, I decided to show a sequence of LEDs light up as if HAL has now gained access to nuclear codes or something and then the player has to turn off the LEDs using a potentiometer to beat HAL. This entire sequence from the narration of the dialogue by HAL and his defeat at the hands of player commences when the player presses the button and can be played over and over again.
The defeat of HAL is signified by his LED turning dimmer and finally off (showing that he dead).
Phases of the Project
In order to make things easier for me, I decided to test everything out in tinkercad and then once I was sure everything worked the way i wanted it to, i implemented it on my Arduino UNO.
So the phases of the project were as follows:
Morse Code
For this I searched up a morse code letter translator (see image) and represented the dots with short bursts of brightness on the LEDs and the dashes as long bursts.
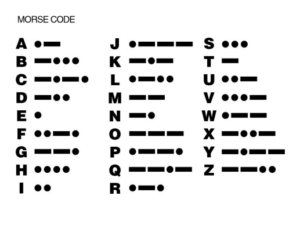
The dialogue to be converted was “I am sorry Dave.”
The below code and circuit outlet helped do this.
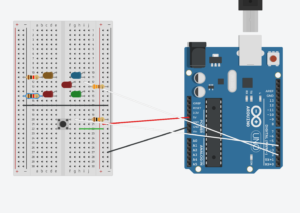
Code:
int ledPin = 2; int buttonPin = 4; int shortTime = 300; int longTime = 900; int prevButtonState = LOW; int play_dialogue = 0; void flash_led(int timing) { digitalWrite(ledPin, HIGH); delay(timing); digitalWrite(ledPin, LOW); delay(shortTime); } void to_morse(int order, int code0 =0, int code1 = 0, int code2 = 0, int code3 = 0) { int code_array[4]; code_array[0] = code0; code_array[1] = code1; code_array[2] = code2; code_array[3] = code3; for (int i = 0; i <order; i++) { flash_led(code_array[i]); } delay(longTime); } void setup() { pinMode(ledPin, OUTPUT); pinMode(buttonPin, INPUT); } void loop() { int currentButtonState = digitalRead(buttonPin); // if the button is currently being prssed down, AND during the last frame is wasn't pressed down if (currentButtonState == HIGH && prevButtonState == LOW) { // flip the LED state if (play_dialogue == HIGH){ play_dialogue = LOW; } else if (play_dialogue == LOW){ play_dialogue = HIGH; } } if(play_dialogue){ to_morse(2, shortTime, shortTime); //I to_morse(2, shortTime, longTime); //A to_morse(2, longTime, longTime); //M to_morse(3, shortTime, shortTime, shortTime); //S to_morse(3, longTime, longTime, longTime); //O to_morse(3, shortTime, longTime, shortTime); //R to_morse(3, shortTime, longTime, shortTime); //R to_morse(4, longTime, shortTime, longTime, longTime); //Y to_morse(3, longTime, shortTime, shortTime); //D to_morse(2, shortTime, longTime); //A to_morse(4, shortTime, shortTime, shortTime, longTime); //V to_morse(1, shortTime); //E digitalWrite(ledPin, HIGH); play_dialogue = 0; } }
Carousel
The carousel had two components:
- Showing the code by lighting all the LEDS in a sequence
- This was implemented using a digitalWrite and delay
- Turning off the LEDS using a potentiometer
- For this specific parts of the knobValue had to section (divided into four parts) and the brightness of each LED was dependent on the particular section of the knobValue (see code for reference)
Below is a circuit and the accompanying code for this part
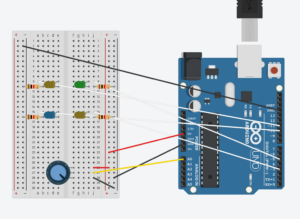
int ledPin1 = 3; int ledPin2 = 5; int ledPin3 = 11; int ledPin4 = 10; int knobPin = A0; int b_led1 = 255; int b_led2 = 255; int b_led3 = 255; int b_led4 = 255; void setup() { pinMode(ledPin1, OUTPUT); pinMode(ledPin2, OUTPUT); pinMode(ledPin3, OUTPUT); pinMode(ledPin4, OUTPUT); Serial.begin(9600); } void loop() { int knobValue = analogRead(knobPin); if(knobValue <= 255) b_led4 = map(knobValue,0,255,255,0); if(knobValue > 255 && knobValue <= 511) b_led3 = map(knobValue,256,511,255,0); if(knobValue > 511 && knobValue <= 767) b_led2 = map(knobValue,512,767,255,0); if(knobValue > 767 && knobValue <= 1023) b_led1 = map(knobValue,768,1023,255,0); Serial.println("This is knobValue: "); Serial.println(knobValue); Serial.println("This is brightness of LED4 "); Serial.println(b_led4); Serial.println("This is brightness of LED3 "); Serial.println(b_led3); Serial.println("This is brightness of LED2 "); Serial.println(b_led2); Serial.println("This is brightness of LED1 "); Serial.println(b_led1); digitalWrite(ledPin1, HIGH); delay(1500); // Wait for 1000 millisecond(s) digitalWrite(ledPin2, HIGH); delay(1500); // Wait for 1000 millisecond(s) digitalWrite(ledPin3, HIGH); delay(1500); // Wait for 1000 millisecond(s) digitalWrite(ledPin4, HIGH); delay(1500); // Wait for 1000 millisecond(s) analogWrite(ledPin1, b_led1); analogWrite(ledPin2, b_led2); analogWrite(ledPin3, b_led3); analogWrite(ledPin4, b_led4); }
Bringing them Together
Finally here is a video showing how it all came together (included outputs in the serial Monitor to make it more fun!)
And here is the final code and circuit
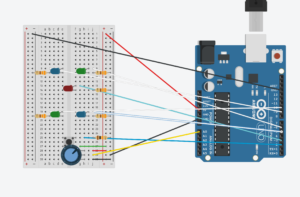
int halPin = 3; int buttonPin = 2; int ledPin1 = 5; int ledPin2 = 6; int ledPin3 = 9; int ledPin4 = 10; int knobPin = A0; int b_led1 = 255; int b_led2 = 255; int b_led3 = 255; int b_led4 = 255; int shortTime = 225; int longTime = 675; int prevButtonState = LOW; int play_dialogue = 0; int stop_carousel = 0; int hal_destruction =0; int hal_defeated =0; void flash_led(int timing) { digitalWrite(halPin, HIGH); delay(timing); digitalWrite(halPin, LOW); delay(shortTime); } void to_morse(int order, int code0 =0, int code1 = 0, int code2 = 0, int code3 = 0) { int code_array[4]; code_array[0] = code0; code_array[1] = code1; code_array[2] = code2; code_array[3] = code3; for (int i = 0; i <order; i++) { flash_led(code_array[i]); } delay(longTime); } void setup() { pinMode(halPin, OUTPUT); pinMode(buttonPin, INPUT); pinMode(ledPin1, OUTPUT); pinMode(ledPin2, OUTPUT); pinMode(ledPin3, OUTPUT); pinMode(ledPin4, OUTPUT); Serial.begin(9600); } void loop() { int currentButtonState = digitalRead(buttonPin); // if the button is currently being prssed down, AND during the last frame is wasn't pressed down if (currentButtonState == HIGH && prevButtonState == LOW) { // flip the LED state if (play_dialogue == HIGH){ play_dialogue = LOW; } else if (play_dialogue == LOW){ play_dialogue = HIGH; b_led1 = 255; b_led2 = 255; b_led3 = 255; b_led4 = 255; } } if(play_dialogue){ Serial.println("Dave: Welcome to the.....oh my god it is Hal"); hal_defeated = 0; Serial.print("Hal: "); to_morse(2, shortTime, shortTime); //I Serial.print("I"); Serial.print(" "); to_morse(2, shortTime, longTime); //A Serial.print("A"); to_morse(2, longTime, longTime); //M Serial.print("M"); Serial.print(" "); to_morse(3, shortTime, shortTime, shortTime); //S Serial.print("S"); to_morse(3, longTime, longTime, longTime); //O Serial.print("O"); to_morse(3, shortTime, longTime, shortTime); //R Serial.print("R"); to_morse(3, shortTime, longTime, shortTime); //R Serial.print("R"); to_morse(4, longTime, shortTime, longTime, longTime); //Y Serial.print("Y"); Serial.print(" "); to_morse(3, longTime, shortTime, shortTime); //D Serial.print("D"); to_morse(2, shortTime, longTime); //A Serial.print("A"); to_morse(4, shortTime, shortTime, shortTime, longTime); //V Serial.print("V"); to_morse(1, shortTime); //E Serial.print("E"); Serial.println(" "); digitalWrite(halPin, HIGH); play_dialogue = 0; //start_carousel = 1; hal_destruction = 1; } //if (start_carousel){ if (hal_destruction){ digitalWrite(ledPin1, HIGH); delay(1500); // Wait for 1000 millisecond(s) digitalWrite(ledPin2, HIGH); delay(1500); // Wait for 1000 millisecond(s) digitalWrite(ledPin3, HIGH); delay(1500); // Wait for 1000 millisecond(s) digitalWrite(ledPin4, HIGH); delay(1500); // Wait for 1000 millisecond(s) Serial.println("Dave: Oh No! Hal has started the code that would blow up the planet! You must stop him! "); stop_carousel = 1; } while(stop_carousel){ int knobValue = analogRead(knobPin); if(knobValue > 0) hal_destruction = 0; if(knobValue > 0 && knobValue <= 255){ b_led4 = map(knobValue,0,255,255,0); } if(knobValue > 255 && knobValue <= 511){ b_led4 = 0; b_led3 = map(knobValue,256,511,255,0); } if(knobValue > 511 && knobValue <= 767){ b_led3 = 0; b_led2 = map(knobValue,512,767,255,0); } if(knobValue > 767 && knobValue <= 1023){ b_led2 = 0; b_led1 = map(knobValue,768,1023,255,0); } analogWrite(ledPin1, b_led1); analogWrite(ledPin2, b_led2); analogWrite(ledPin3, b_led3); analogWrite(ledPin4, b_led4); if(knobValue == 1023) { hal_defeated = 1; stop_carousel = 0; } } //} if(hal_defeated){ //start_carousel = 0; digitalWrite(halPin, LOW); //if(ending_dialogue){ Serial.println("Dave: Thank God! You defeated Hal!"); Serial.println("To play again, press the button."); //} hal_defeated = 0; } }
Hurdles along the way
To be honest, there weren’t many. This project went forth pretty smoothly and really helped me solidify the concepts we learned in week 8 and 9. And I had lots of fun making this!