This weeks exercise took a lot of time, or should I rather say, I spend a lot of time playing around and getting to know Processing better? I rewatched and followed along the different video tutorials by The Coding Train which helped a lot but also led to endless possibilities.
I took inspiration from a Ginkgo leaf and the kaleidoscopes I used to like as a child. I wanted to create several layers of ginkgo leaves that rotate in different directions but are layered on top of one another in the center of the screen. However, when I started writing the code, other ideas and possibilities came to my mind so the first challenge was to make decisions. In-between I tried something else and my final version is also not quite what I initially had in mind but I was able to learn along the way.
The second challenge was whether to start with highest part of the code hierarchy that should just look simple and ‘pretty’ at the end or with creating my own objects and functions. As I am still pretty new to processing, I had no ‘best practice’ but after this exercise I think it’s easier to start with the “pretty” part and then build the “background code” depending on how I want the hierarchy to look like.
My code for this exercise is not fully polished and simplified yet because I ran into some difficulties where I will need to check ‘pass by reference’ and ‘pass by copy’ again but enjoy this little Ginkgo leaf inspired kaleidoscope which reacts to the position of the mouse.
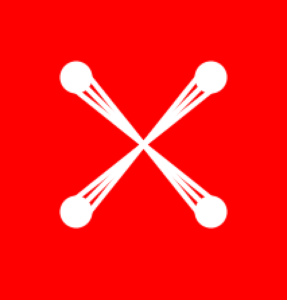
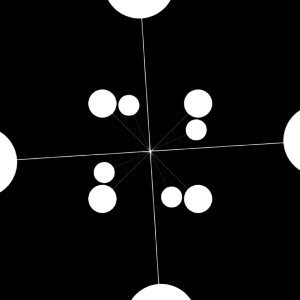
This is the main code, followed by the code on the additional tab for the Leaf class.
float x, y, r; float a, b; float angle; Leaf l1; Leaf l2; Leaf l3; void setup() { size(640, 640); //Instagram format background(0); l1 = new Leaf(); l2 = new Leaf(); l3 = new Leaf(); } void draw() { background(0); translate(width/2, height/2); //this centers the shape on the screen push(); //I am using push() and pop() because otherwise the scale would apply to the previous value. need to solve this. l1.display(mouseY/250); //this will change the size according to the mouse position pop(); push(); rotate(angle); angle = angle + 0.5; l2.display(1.5); pop(); push(); rotate(-angle); angle = angle + 0.3; l3.display(mouseX/100); //this will change the size according to the mouse position pop(); }
class Leaf { float a; float r; float angle; float s; Leaf() { //this sets up the parameters that we can later determine when calling the function } void display(float tempS) { //this is the basic shape of the leaf //translate(width/2, height/2); s = tempS; scale(s); stroke(255); strokeWeight(0.5); //thin stroke weight to make leaf structure finer fill(255); //this is the basic shape of the leaf (somewhat hardcoded still) //these are the basic two intersecting lines with a stronger strokeWeight than the other lines line(-50, -50, +50, +50); line(+50, -50, -50, +50); strokeWeight(0.01); //this makes the extra lines thinner line(-50+r, -50, +50-r, +50); line(-50, -50+r, +50, +50-r); line(+50-r, -50, -50+r, +50); line(+50, -50+r, -50, +50-r); ellipse(-50, -50, r*2, r*2); ellipse(+50, +50, r*2, r*2); ellipse(+50, -50, r*2, r*2); ellipse(-50, +50, r*2, r*2); //this adds a smaller ellipse into the bigger ellipse fill(255); ellipse(-50, -50, r*1.5, r*1.5); ellipse(+50, +50, r*1.5, r*1.5); ellipse(+50, -50, r*1.5, r*1.5); ellipse(-50, +50, r*1.5, r*1.5); r=15; } void move() { rotate(angle); angle = angle + 1; } }
Was it intentional to make it flash? It doesn’t seem like it was based on your code. It makes me think things are moving really really fast (faster than they should). It’d be good to figure out why it’s flashing and either do it on purpose or not flash on purpose.