There she is, Processing Sarah and her two moods!
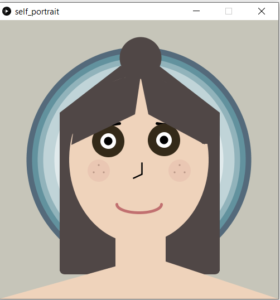
I’m not sure what the second mood is, but maybe a video will make it clearer.
I started working by looking for inspiration, I looked through the old blog posts here, openprocessing.org (which is a great source to look at the works of people from all over the world and different age and skill levels), and youtube.
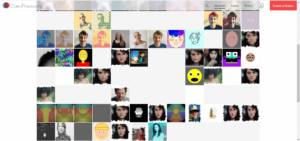
This research helped me find new tools I could use for the more complex shapes such as the beginShape(), endShape() function. Additionally, thanks to Jana from last(?) year, I found a cursor tracker code that helped a lot in finding the coordinates of certain points on the screen. You can find that code at the end of my work.
Next, I sketched a really rough draft to brainstorm and figure out the geometric shapes and functions needed to create my portrait. I then realized that some elements of the portrait will use the arc function, so I spent some time playing around with it and inserting different values and angles.
I got to coding the static elements of my portrait. I tried my best to make it resemble me somehow and I found that giving it a half-bun would be perfect to mark that resemblance.
Finally, I wanted to add a factor of animation and thought that going for a moving background could be cool. I settled for making something that makes my character look like she’s in a state of hypnosis…? I drew multiple circles in the code and created an “expand” variable that was added with every iteration of the draw function until the circle width and height reached a specific value which corresponds to the screen size (or the previous circle). Then, it restarts from the default width and height. When you hover over the eyes, you get a similar effect which was done using the same logic.
here’s the code!
// color palette: color bgColor = color(198,197,185); color skinColor = color(239,211,187); color noseColor = color(220,190,200); color blushColor = color(193,112,122,30); color lipColor = color(193,112,112); color frecklesColor = color(184,150,133); color hairColor = color(80,71,70); color firstBlue = color(84,106,123); color secondBlue = color(98,146,158); color thirdBlue = color(224,234,236); color irisColor = color(52,42,25); //declaring variables int x; int y; int ellipse1WH; int ellipse2WH; int ellipse3WH; int ellipse4WH; int ellipse5WH; int iris1WH; int expand = 3; int irisExpand = 1; void setup(){ size(500,500); x = width/ 2; y = height / 2; ellipse1WH = 100; ellipse2WH = 80; ellipse3WH = 60; ellipse4WH = 40; ellipse5WH = 20; iris1WH = 6; } void draw(){ //moving bg background(bgColor); stroke(firstBlue); strokeWeight(60); noFill(); //drawing the circles ellipse(x,y,ellipse1WH,ellipse1WH); stroke(secondBlue); ellipse(x,y,ellipse2WH,ellipse2WH); stroke(145,179,187); ellipse(x,y,ellipse3WH, ellipse3WH); stroke(192,212,216); ellipse(x,y,ellipse4WH,ellipse4WH); stroke(thirdBlue); strokeWeight(40); ellipse(x,y,ellipse5WH,ellipse5WH); //circles expanding ellipse1WH = ellipse1WH + expand; ellipse2WH = ellipse2WH + expand; ellipse3WH = ellipse3WH + expand; ellipse4WH = ellipse4WH + expand; ellipse5WH = ellipse5WH + expand; //upper limit if (ellipse1WH > 500 ){ ellipse1WH = 100; } if (ellipse2WH > 480 ){ ellipse2WH = 80; } if (ellipse3WH > 460 ){ ellipse3WH = 60; } if (ellipse4WH > 440 ){ ellipse4WH = 40; } if (ellipse5WH > 420 ){ ellipse5WH = 20; } //hair1, behind the face noStroke(); fill(hairColor); //right side beginShape(); vertex(286,82); vertex(395,166); vertex(395,450); vertex(360,450); vertex(269,96); endShape(); rect(108,356,287,100,10); beginShape(); vertex(217,82); vertex(108,166); vertex(108,450); vertex(143,450); vertex(269,96); endShape(); //face fill(skinColor); noStroke(); ellipse(x,y,250, 300); //neck rect(208,380,90,100,7); //torso triangle(0,500,271,424,271,500); triangle(500,500,271,424,271,500); //eyebrows strokeWeight(5); stroke(0); line(180,190,215,185); line(282,185,317,190); //eyes arc(197,213,35,13,0, PI); //left arc(295,211,35,13,0,PI); // right //eyelashes strokeWeight(1); //left eye line(179,215,174,219); line(186,219,183,224); line(195,218,195,225); line(203,218,207,225); line(213,216,219,220); //right eye line(279,215,274,219); line(286,219,283,222); line(295,218,295,223); line(303,218,307,223); line(310,212,318,219); //eye animation if (mouseX >= 176 && mouseX <= 215 && mouseY >= 208 && mouseY <= 222 || mouseX >= 276 && mouseX <= 313 && mouseY >= 208 && mouseY <= 222 ){ //covers closed eyes stroke(skinColor); fill(skinColor); ellipse(195,213,55,30); ellipse(295,211,55,30); //open eyes fill(255); ellipse(195,217,50,50); ellipse(295,215,50,50); stroke(irisColor); strokeWeight(15); ellipse(195,217,iris1WH,iris1WH); ellipse(295,215,iris1WH,iris1WH); noStroke(); fill(0); ellipse(195,217,15, 15); ellipse(295,215,15,15); //eye expanding iris1WH = iris1WH + irisExpand; if (iris1WH > 45) { iris1WH = 6; } } ////nose noFill(); stroke(0); strokeWeight(2.5); beginShape(); vertex(255,256); vertex(255,276); vertex(240,283); endShape(); //cheeks/blush //left noStroke(); fill(blushColor); ellipse(178,270,40,40); //right ellipse(323,270,40,40); //freckles fill(frecklesColor); //left ellipse(178,260,3,3); ellipse(169,273,3,3); ellipse(187,273,3,3); //right ellipse(323,260,3,3); ellipse(314,273,3,3); ellipse(332,273,3,3); //mouth stroke(lipColor); strokeWeight(5); noFill(); arc(250,330,80,30,0,PI); //hair2, bangs noStroke(); fill(hairColor); //left beginShape(); vertex(253,101); vertex(243,169); vertex(129,224); vertex(132,202); vertex(228,100); endShape(); beginShape(); vertex(224,102); vertex(161,127); vertex(116,200); vertex(113,221); vertex(230,113); endShape(); //right beginShape(); vertex(253,101); vertex(267,169); vertex(368,223); vertex(371,202); vertex(280,100); endShape(); beginShape(); vertex(284,104); vertex(352,137); vertex(387,183); vertex(387,222); vertex(364,222); vertex(277,128); vertex(286,94); endShape(CLOSE); //bun ellipse(253,68,75,75); //cursor tracker from Jana print(mouseX); print(" "); println(mouseY); }