Several weeks ago I went to a school-organized paintball trip. It was pretty fun, though the paintball gun is heavy, my aiming is the worst, and it actually hurt when you’re hit. So, I made a paintball game with processing that allows you to shoot your enemies WITHOUT being shoot back. Of course, to make it a bit fairer, the enemy have a few barriers to hide behind.
When the game starts, it looks like this:
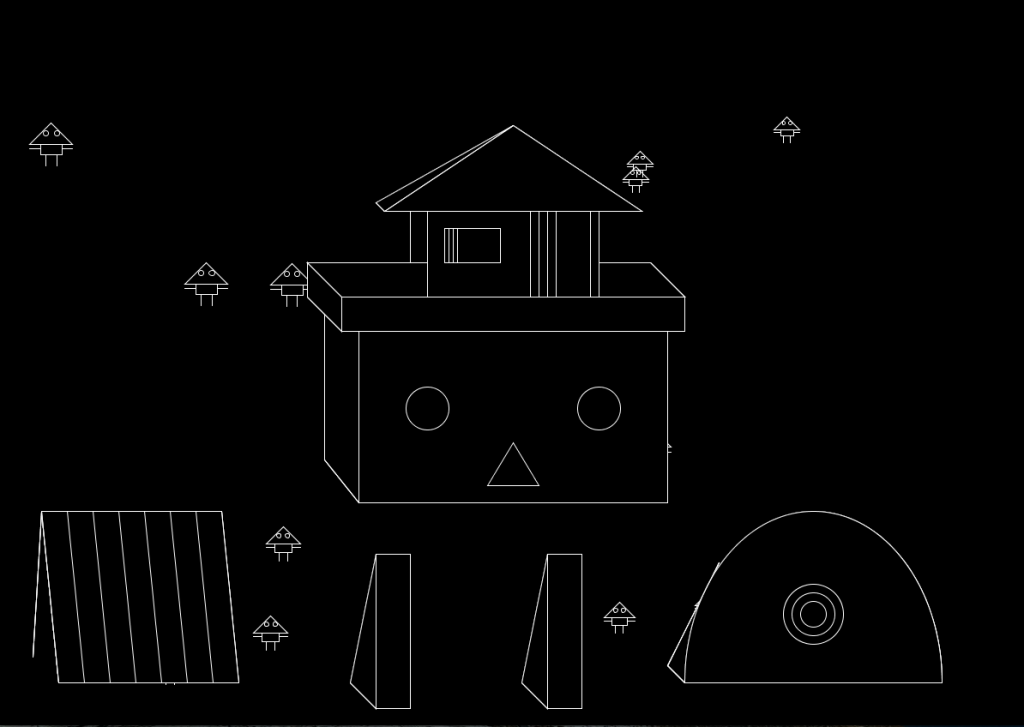
As time accumulates, the number of enemies will only increase, until they probably occupy the whole screen( which is horrifying, especially for those with Trypophobia. Sorry.) You can shoot your virtual paintball by clicking your mouse or drag it. The color of the paint is totally random, and there is actually a delay of less than a second before the paint is shown, partially to imitate the reality that bullet needs to travel a certain distance before it hits anything.
If you do hit the newest appearing enemy on the screen —- congrats! You will see a huge pool of blood/ red paint on that spot. Harsh right?
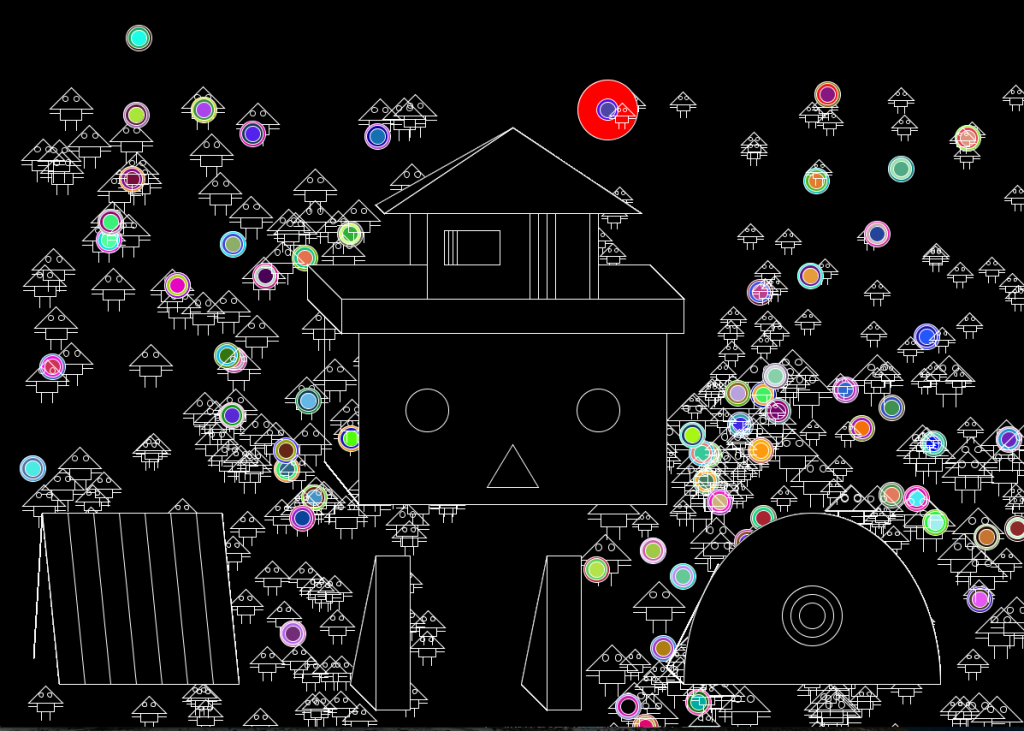
Finally you could switch the game to what I call the impossible mode. Instead of accumulating the enemy population overtime, the five targets will appear at random positions on the screen every second. It is literally impossible to hit them. But I think it would be interesting for a game to disappoint/ upset other than please people. That’s why I kept this one additional feature. It also serves as a way to reset the game.
Here’s the code:
Enemy myEnemy1; Enemy myEnemy2; Enemy myEnemy3; Enemy myEnemy4; Enemy myEnemy5; float quadX = 50; float quadY = 600; void setup() { size(1200,850); background(0); stroke(255); myEnemy1 = new Enemy(50, 500, 20); myEnemy2 = new Enemy(700, 400, 30); myEnemy3 = new Enemy(700, 100, 15); myEnemy4 = new Enemy(50, 100, 25); myEnemy5 = new Enemy(700, 600, 18); } void draw() { if((keyPressed == true) && (key == 'k')) { background(0); } noFill(); if( millis() % 1000 <= 8) { myEnemy1.update(); myEnemy2.update(); myEnemy3.update(); myEnemy4.update(); myEnemy5.update(); } myEnemy1.shoot(); myEnemy2.shoot(); myEnemy3.shoot(); myEnemy4.shoot(); myEnemy5.shoot(); fill(0); quad(quadX, quadY, quadX+210, quadY, quadX+230, quadY+200, quadX+20 , quadY+200); // draw a lower left corner wood fence line(quadX, quadY, quadX-10, quadY+170); for( int i=0; i<=6; i++) { line(quadX+30*i, quadY, quadX+(30*i+20), quadY+200); } arc(950, 800, 300, 400, radians(180), radians(360), CHORD); // draw an arc-shaped lower right corner barrier noFill(); line(800, 800, 780, 780); line(780, 780, 840, 660); ellipse(950, 720, 30, 30); ellipse(950, 720, 50, 50); ellipse(950, 720, 70, 70); fill(0); rect(440, 650, 40, 180); // draw a lower middle entrance rect(640, 650, 40, 180); triangle(440, 650, 440, 830, 410, 800); triangle(640, 650, 640, 830, 610, 800); quad(500, 250, 500, 350, 480, 330, 480, 250); // draw a main block in the middle rect(400, 350, 400, 40); quad(400, 350, 400, 390, 360, 350, 360, 310); quad(360, 310, 760, 310, 800, 350, 400, 350); rect(420, 390, 360, 200); triangle(600, 520, 630, 570, 570, 570); ellipse(500, 480, 50, 50); ellipse(700, 480, 50, 50); line(420, 590, 380, 540); line(380, 540, 380, 370); triangle(600, 150, 750, 250, 450, 250); triangle(600, 150, 450, 250, 440, 240); rect(500, 250, 200, 100); for( int j=0; j<=3; j++) { rect(520+5*j, 270, 50, 40); rect(620+10*j, 250, 40, 100); } } class Enemy { float xPos; float yPos; float side; Enemy( float x, float y, float s) { xPos = x; yPos = y; side = s; } void update() { pushMatrix(); translate( random(500), random(500)); // draw the enemy triangle( xPos, yPos, xPos+side, yPos+side, xPos-side, yPos+side); rect(xPos-side/2, yPos+side, side, side/2); ellipse(xPos-side/4, yPos+side/2, side/4, side/4); // left eye ellipse(xPos+side/4, yPos+side/2, side/4, side/4); // right eye line(xPos-side/2, yPos+side*1.2, xPos-side, yPos+side*1.2); // arms line(xPos+side/2, yPos+side*1.2, xPos+side, yPos+side*1.2); line(xPos-side/4, yPos+side*1.5, xPos-side/4, yPos+side*2); // legs line(xPos+side/4, yPos+side*1.5, xPos+side/4, yPos+side*2); popMatrix(); } void shoot() { cursor(CROSS); if( mousePressed == true) { fill(random(0,255), random(0,255), random(0,255)); ellipse(mouseX, mouseY, side, side); // Shoot paintballs } if ((mouseX>xPos-side) && (mouseX<xPos+side) && (mouseY>yPos) && (mouseY<yPos+side*2)) { fill(255, 0, 0); ellipse(mouseX, mouseY, 70, 70); // paint turns to red if hit the target } } }