This is art:
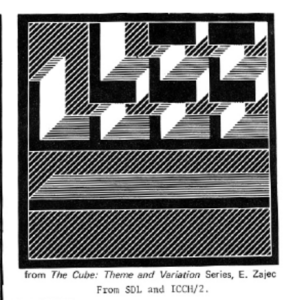
But I felt it needed more color, so I recreated it!
The time-consuming and tedious-ish part was drawing all those lines. Drawing them one by one would be suicide modern torture, so I made use of (many) many for() loops. That way, I told the program how to iterate the drawing of lines. at what interval, in which coordinates, etc. It was tricky, especially when trying to draw lines over irregular shapes: to do so, I had to use if() statements and/or constrain() to make the lines stop where needed.
Moreover, to line-fill similar areas I used nested for() loops. With two parameters, I could iterate the same line-shape in several places of the screen. Still, this didn’t solve all the issues that arise from the irregular shapes, so in the end I had to draw several lines manually (because adding more for() loops for such a small number of lines would be too much effort for little to no benefit).
So yeah. My code is a mess (I reaaally should have labeled which for() loop drew what), but hey, it works!
As a quick added bonus, I made the stroke color random (like in my flashy screens) to give the piece a trippy, retro feel my personal touch.
The code is below:
void setup() { size(600, 600); frameRate(60); } void draw() { background(0); stroke(random(0, 255), random(0, 255), random(0, 255)); noFill(); rect(20, 20, 560, 560); line(20, 450, 580, 450); line(160, 20, 160, 180); line(90, 80, 150, 20); line(100, 80, 160, 20); line(240, 80, 300, 20); line(250, 80, 300, 30); line(300, 70, 310, 60); line(440, 70, 450, 60); line(390, 80, 410, 60); line(380, 80, 400, 60); line(530, 80, 550, 60); line(520, 80, 540, 60); line(110, 200, 160, 150); //^Those are the I-was-too-lazy-to-iterate-them lines for (int a = 1; a <= 3; a ++) { line(20 + a*140, 180, 140 + a*140, 180); line(50 + a*140, 140, 140 + a*140, 140); if (a != 1) { line(20 + a*140, 60, 140 + a*140, 60); line(20 + a*140, 180, 20 + a*140, 160); line(20 + a*140, 20, 20 + a*140, 60); } } for (int a = 1; a <= 3; a ++) { for (int q = 160; q <= 220; q ++) { if (a == 1) { line(30 + (a - 1)*140 + q, 300 - q, 30 + (a - 1)*140 + q, 20); } else { int w = constrain(240 - q, 60, 300); line(30 + (a - 1)*140 + q, 300 - q, 30 + (a - 1)*140 + q, w); } } } for (int a = 1; a <= 3; a ++) { for (int q = 120; q <= 140; q ++) { line(20 + a*140 + q, 300 - q, 20 + a*140 + q, 260 - q); if (a != 1) { line(20 + a*140 + q, 180 - q, 20 + a*140 + q, 20); } } } for (int a = 1; a <= 3; a ++) { if (a != 1) { line(20 + a*140, 80, 40 + a*140, 60); for (int q = 80; q > 60; q -= 3) { line(100 + a*140 - q, q, 130 + a*140 - q, q); } } line(20 + a*140, 210, 50 + a*140, 180); } for (int a = 1; a <= 3; a ++) { for (int q = 140; q >= 80; q -= 3) { int w = constrain(280 + a*140 - q, 0, (a+1)*140 + 20); line(190 + a*140 - q, q, w, q); } } for (int a = 30; a <= 140; a += 10) { line(a, 450, 20, 430 + a); } for (int q = 20; q <= 580; q += 10) { int w = constrain(q+ 130, 0, 580); int e = 450; if (w == 580) { e = q; } line(q, 580, w, e); } line(20, 420, 580, 420); line(20, 300, 580, 300); for (int a = 360; a < 420; a += 3) { line(440 - a, a, 580, a); } for (int q = 30; q <= 580; q += 10) { int w = 280 + q; int e = constrain(q - 120, 20, 580); if (q > 140) { w = 360; e = q - 60; } line(q, 300, e, w); } for (int q = 310; q < 360; q += 10) { line(580, q, 220 + q, 360); } for (int q = 140; q >= 80; q -= 3) { line(160 - q, q, 190 - q, q); } for (int q = 50; q <= 110; q ++) { line(q, 190 - q, q, 320 - q); } for (int a = 0; a <= 3; a ++) { for (int q = 270; q >= 210; q -= 3) { line(320 - q + a*140, q, 20 + (a + 1)*140, q); } } for (int a = 0; a <= 2; a ++) { for (int q = 210; q >= 180; q -= 3) { line(370 - q + a*140, q, 400 - q + a*140, q); } } for (int a = 0; a <= 2; a++) { for (int q = 190; q <= 250; q ++) { int s = constrain(400 - q, 180, 400); line(a*140 + q, 460 - q, a*140 + q, s); } } for (int q = 30; q <= 140; q += 10) { line(20, q, q, 20); line(160, q, 100, q + 60); } for (int q = 30; q <= 70; q += 10) { line(250, q, 230 + q, 20); } for (int a = 0; a <= 3; a ++) { for (int w = 10; w <= 40; w += 10) { line(20 + (a+2)*140, 30 + w, (a+2)*140 - 30 + w, 80); } for (int w = 10; w <= 30; w += 10) { line(100 + a*140, 180 + w, 100 + a*140 + w, 180); if (a != 3) { line(160 + a*140, 180 + w, 160 + a*140 + w, 180); } } for (int q = 240; q < 300; q += 10) { line(10 + (a-1)*140 + q, 210, 300 + (a-1)*140, q - 80); } } } //EASTER EGG YAY void keyPressed() { if (key == ' ') { fill(255); textSize(random(24, 72)); text("YAY", random(0, 550), random(72, 600)); text("CUBE [ ]", random(0, 550), random(72, 600)); text("BREAD", random(0, 550), random(72, 600)); text("ART", random(0, 550), random(72, 600)); text("dis is gr8", random(0, 550), random(72, 600)); } }