For this assignment I decided to try out the solenoids to produce linear motion. Inspired by woodpeckers (thanks, Shunya), I set out to create a device that would produce ringing noises by hitting objects with the solenoids.
First I had to get acquainted with the solenoids. To use them, I had to hook them up to a transistor in order to not fry my RedBoard. Transistors are annoyingly not labled and their wiring changes between models, so I had to look that up. The TIP120 transistors we have in the lab have, seen from the front and from left to right, the base (signal in), the collector (voltage in) and then the emitter (to ground). That way, I could use the RedBoard’s output to control the transistors, which in turn control the solenoids.
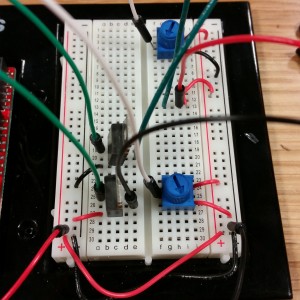
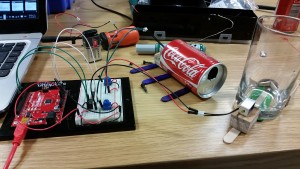
I wanted to control the period of the solenoids’ motion, and to control both independently I couldn’t really use delays. Thus, I had to write my own timers based on Scott’s code in the BlinkWithoutDelay example. Adding an analog input via a pot, I could control in real time how often the solenoid would hit the objects. The code looks like this:
unsigned long timer1 = millis(); //This will count time int pot1 = analogRead(5); //Read pot input period1 = map(pot1, 0, 1023, 1, 200); //Map pot input to period of "oscillation" //previousTimer1 is a global variable that stores the time at which the solenoid was last moved //Comparing it to timer1, I can determine if a period has elapsed and if it's time to move the solenoid if (timer1 - previousTimer1 >= period1) { previousTimer1 = timer1; if (state1 == LOW) state1 = HIGH; else state1 = LOW; digitalWrite(linearPin1, state1); //state1 is a global variable that stores if the solenoid is ON/OFF, btw }
Earlier code prototypes used while() loops to count the time. Those, however, pose the problem that I can’t really have two distinct while() “timers” running simultaneously, so it would pose a problem once I added a second solenoid.
Everything worked nicely… until I added solenoid 2. Code-wise, I just copied what I had done for the first solenoid and renamed the variables assigned to solenoid 2. However, my device started acting very strangely: instead of being controlled independently, the two pots would control both solenoids, making them ring in very weird (but quite regular) patterns. Scott recommended having a single, global timer to keep trak of the time the program had been running, but I don’t know if that was what later fixed the problem.
Another problem I had was dealing with the power supply. The arduino isn’t powerful enough to run both solenoids at once, unfortunately, and the 9V battery I had used for my Stupid Pet Trick is apparently now dead. So I had to hack a power supply, solder some male headers onto it, and plug that into my breadboard as an external 12V power supply. Two important things: 1. Decoupling capacitors are always nice, and 2. make sure the Arduino-powered components and externally-powered components share the same ground but not power. Or your Arduino might die.
After those fixes, my project finally worked as intended! Kind of. An unfortunateexpected side effect of the 12V supply was that after a while the solenoids would heat up. And melt the hot glue. So yeah. I guess it can work as a built-in limit so that the ringing doesn’t drive anyone insane! 😀