My inspiration for this assignment came from two sources:
- A quote that “success isn’t linear”,
- Casey Reas’ talk on Chance.
Now, let me explain what I mean.
Human brains are wired in a way to notice only up’s of certain experiences (Kahneman, “Thinking, Fast and Slow”). When looking at success stories of other people, it is oftentimes tempting to simplify their path into a mere collection of linear dots and draw a straight line connecting all. This is why I wanted to challenge this notion through my Processing code, but most importantly, to make a kind reminder to myself, too 🙂
So I took out my iPad and started drawing the image that I was bearing in my head. After looking at some of the examples from the syllabus, I started off with simple, straight lines that were forming a rectangular pattern all throughout the setup window. Then, I decided to add random red lines throughout the screen to perform in a noise-like pattern. All these straight lines serve as a metaphor to a “linear path”.
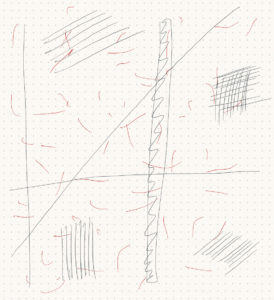
While I was implementing my sketch into code, I was listening to the Casey Reas’ talk on Chance which made me think a lot about random chances and possibilities in the meanwhile. I started playing around with my Processing code, challenging this “chance” to finally show in my work. Aaand…it did!
While I was taking this chance, one of my functions stopped working the way I expected it to work, and started to make a blinking effect on the screen instead. I took this as an opportunity to explore my options and made a slight shift to the initial idea.
It took me a while and a lot of web browsing to figure out how to place the red lines on the screen – however, all to turn the intended lines into rectangles and visit the class-example with the noise function and implement a similar one in my code.
So, in the very end, I got the following interpretation: in a black-and-white world where everything seems so linear, sometimes you have to be a red rectangle and take a step back to build your own, non-conventional path.
float x_width, y_height; float x_coordinate, y_coordinate; float x_center, y_center; float lineX, lineY; void setup(){ size(640, 480); x_width = 10; y_height = 10; x_coordinate = 10; y_coordinate = 10; x_center = width/2; y_center = height/2; } void redNoise(){ float freq; float angle; push(); fill(255, 0, 0); noStroke(); for (int x = 0; x<width; x+=50){ freq = frameCount*.01 + x*.001; angle = noise(freq); rotate(angle); //rect(x_center, y_center, x_width + 40, y_height + 60); rect(mouseX, mouseY, x_width + 40, y_height + 60); } pop(); } void drawLines() { // Section with rectangles for (int i = 0; i < width; i++){ rect(i, y_coordinate, x_width, y_height); rect(width-i, i, x_width, y_height+15); } for (int j = 0; j < height; j++) { rect(x_coordinate + 80, j, x_width, y_height); } // Section with horizontal lines/rectangles int numTotal = 10; for (int rowNum = 0; rowNum < numTotal; rowNum++) { for (int d_width = 0; d_width < numTotal*6; d_width+=5) { rect(3*width/4 + d_width, y_coordinate + 50, 0.5, y_height + 140); rect(width/4 + d_width, y_coordinate + 120, 1, y_height+30); rect(width/2 + d_width, y_coordinate + 320, 1.5, y_height+75); } } // Section with vertical lines/rectangles for (int colNum = 0; colNum < numTotal; colNum++) { for (int d_height = 0; d_height < numTotal*4; d_height+=3) { rect(3*width/4-50, y_coordinate + 30 + d_height, x_width + 100, 0.5); rect(width/8, y_coordinate + 300 + d_height, x_width + 70, 0.3); } } } // Blinking function that worked accidentally void blink(){ for(int i = 0; i < width; i++) { stroke(random(255), 100); line(random(0, width), height/8, i, height/8); } } void draw() { background(255); drawLines(); redNoise(); blink(); }