Finalized concept
For my final project (I’m doing it solo), I decided to make a radio that changes FM values according to what number you turn the potentiometer dial to (I will input song files that play according to their designated serial monitor value).
Each range of values will take you to a different channel.
This includes:
- 4-204 (Hip Hop)
- 205-408 (Historic Events)
- 409-612 (Classic Rock/ Oldies)
- 613-816 (Classical)
- 817-1023 (Khaleeji)
For 0-3 Radio is switched off.
Assembly
To assemble the circuit, I connected 5 different color LEDs to a digital pin on the anode ends, and to each cathode, I connected a resistor. I then connected a potentiometer dial to an analog pin (along with 5V and GND).
Arduino Code
So far, my Arduino code works separately from p5. I’m having trouble connecting both, as I’m trying to convert the 1023 ASCII serial monitor values to p5 without having to change it to 255.
My code generally consists of if statements designating each range of potentiometer values to each LED.
Example:
if ((potMeasure > 3) && (potMeasure <= 204)) { digitalWrite (redPin, HIGH); digitalWrite (yellowPin, LOW); digitalWrite (greenPin, LOW); digitalWrite (tealPin, LOW); digitalWrite (bluePin, LOW); } else if ((potMeasure > 204) && (potMeasure < 409)) { digitalWrite (yellowPin, HIGH); digitalWrite (redPin, LOW); digitalWrite (greenPin, LOW); digitalWrite (tealPin, LOW); digitalWrite (bluePin, LOW); }
The inputs are the LEDs, and the output is the single potentiometer.
p5 Code
I have the p5 code for the serial monitor that picks up values from 0-255, but I’m not sure how to change it so that it can pick up values from 0-1023.
I’m trying to make it so that when inData is between the designated range of values for each channel, it would display the channel name and also play a random song from the designated array of mp3 files.
p5 Screen Design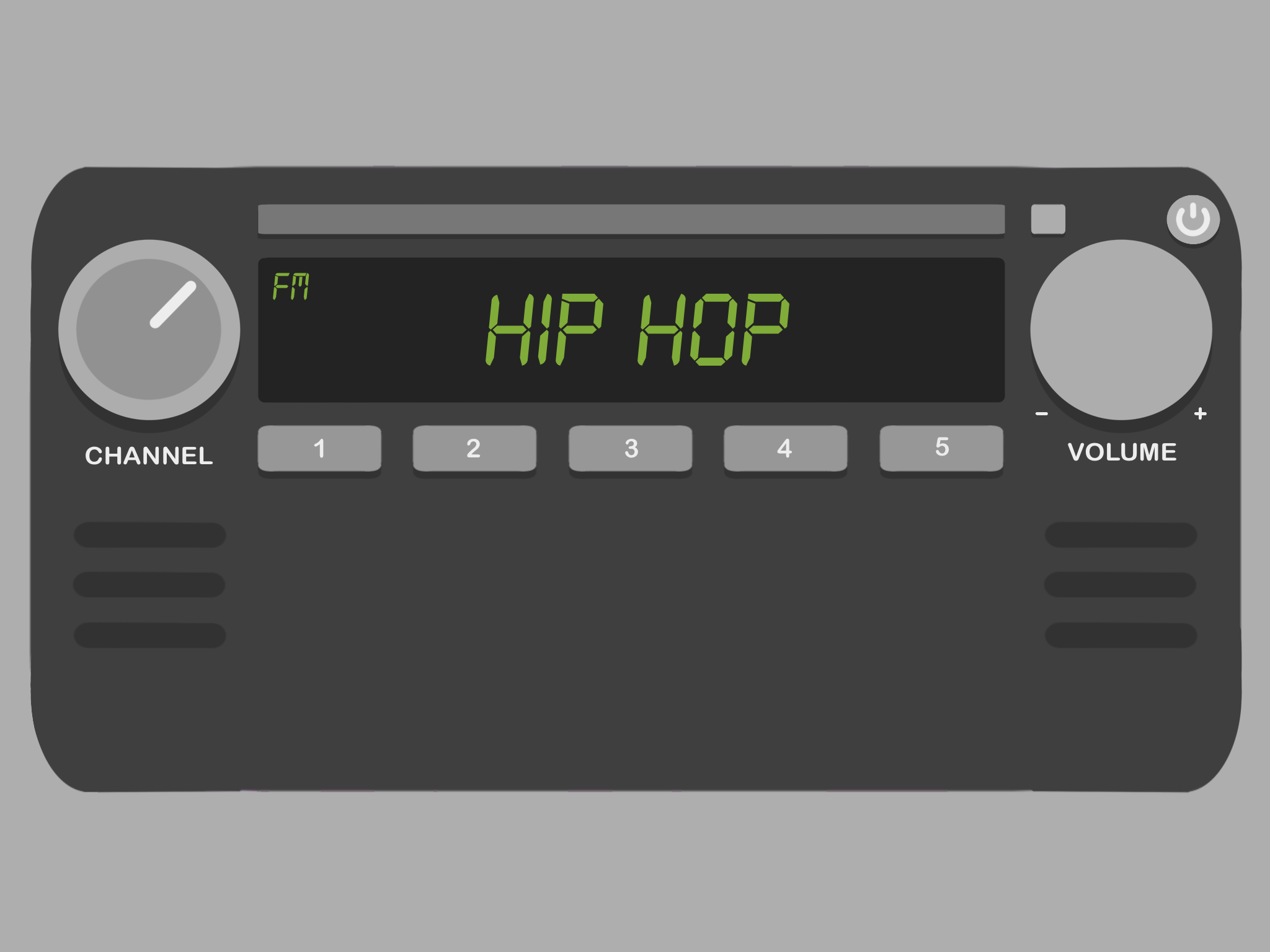
This is the design I made so far, but it of course could be subject to change later on!