Concept
For this idea, I decided to develop a prototype of ambient light. The project makes use of a photoresistor that collects data from the ambiance and lights up an appropriate LED. Initially, I was planning on using a few other tools, in addition to the one already used, but the use of a photoresistor prevented the use of additional tools. Since the resistance of the circuit increases or decreases based on the lighting, adding a few other tools further increased the resistance in the total circuit, as a result, LEDs were not lighting up. Thus, I resorted to a simple design using two LEDs, four resistors (all 330 Ω), two switches, one photoresistor and a few lines of Arduino code.
The idea of the project is straightforward in terms of functionality. The photoresistor detects the amount of light falling on the sensor, which then alters the resistance of the entire circuit. As a consequence of the altered resistance, the potential difference differs under varying conditions. Thus, if the potential difference falls under a particular threshold, one LED lights up and so on.
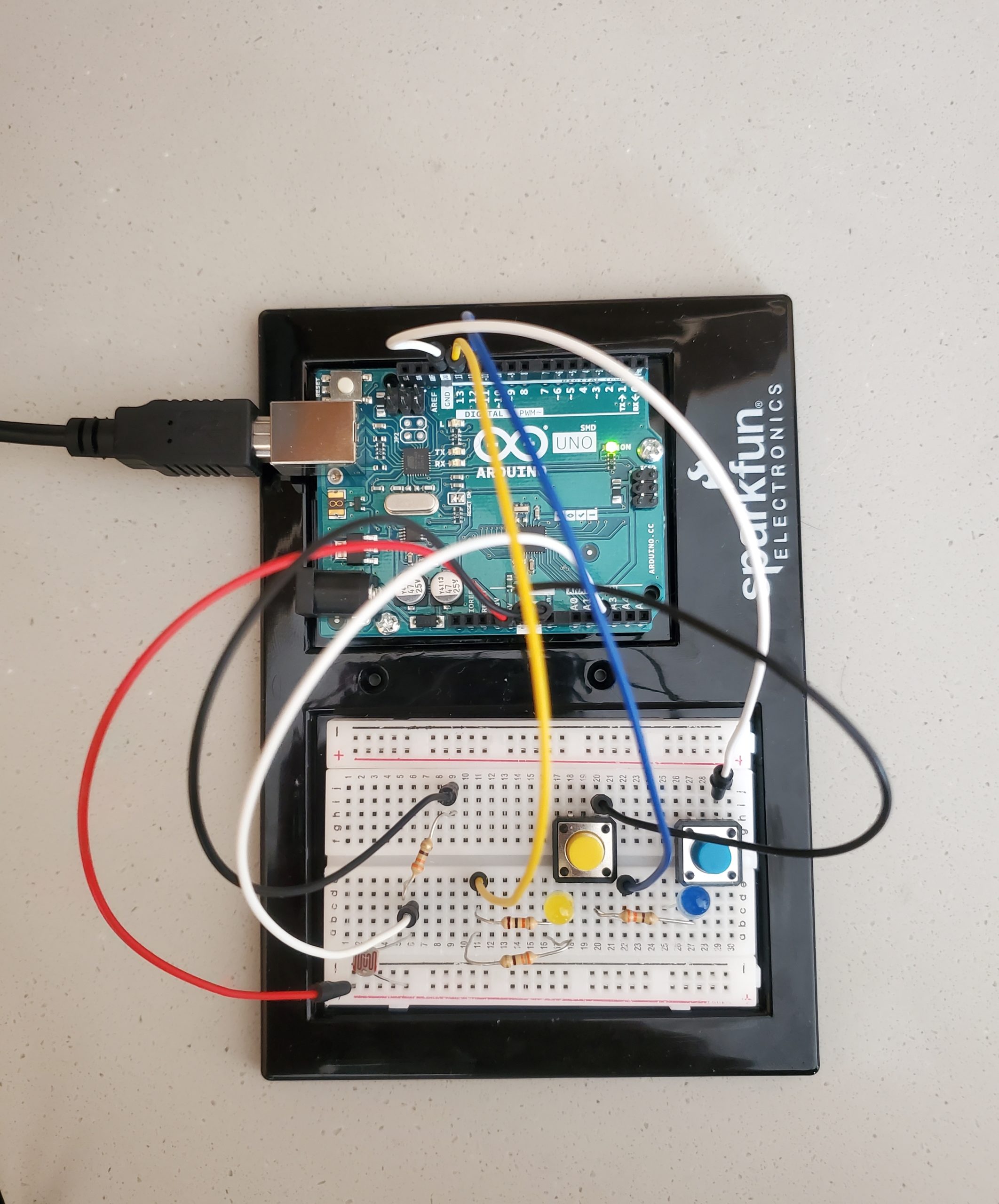
Code
At the beginning of the program, different parameters of the project are controlled by a bunch of global variables declared at the very beginning of the file. Inside the loop() function, the readings from the photo sensor are stored in a variable called ‘volt_reading’ – the value is a range of (1-1023). However, to replicate the actual configuration, I converted this value to an equivalent volt.
// Reading data from the photoresistor and storing it in volt_read
volt_read = analogRead(lightSensorPin);
// Mapping to a range of (0.0 V to 5.0 V)
float volt_converted = volt_read * (5.0/ 1023);
Here, the idea is similar to that of the map() function of p5.js. The value to be converted is mapped to a range of 1.0 V to 5.0 V since 5.0 V is the electromotive force (emf) of the circuit. This value is used in the latter section.
The LED controlling phase involves the use of conditional loops. The code that regulates which LED to light up is placed inside a function titled whichSensor() that takes the converted voltage reading as a parameter. The function is called recursively inside the loop() function.
// Function that determines which LED to light up - takes converted volt reading as input
int whichSensor(int volt)
{
int value_to_be_returned;
if (volt >= 0 && volt < 1.8)
{
value_to_be_returned = 13;
}
else if (volt >= 1.5 && volt < 4.0)
{
value_to_be_returned = 12;
}
return value_to_be_returned;
}
Inside this function, two if-conditions run continuously and check the value stored in the argument — if the value is a range of [0, 1.8) V, digital pin “13” is used to light up a yellow LED; similarly, if the value is a range of [1.8, 5.0] V, digital pin “12” is used to light up the blue LED.
View the entire project on GitHub.
Reflection
The project involves the use of a photoresistor (an analog sensor). In order to make it more realistic, I added two switches to the circuit. Thus, if the yellow switch is pressed and the sensor is giving a certain reading, the yellow LED lights up and vice versa.
Similarly, at first, the yellow LED was less fluorescent, so I added one 330 Ω resistor parallel to the already present resistor. This decreased the resistance of the circuit and the yellow LED was brighter.
Overall, I am happy with the results. I wanted to include a total of three LEDs, but I could not because there are just ground terminals in the circuit — at present, all of them have been used. Moreover, my initial thought was to induce a flickering effect on the second LED when the first LED is emitting light, but the use of switches prevented this idea. Thus, one improvement could be to devise a mechanism that facilitates the implementation of the aforementioned idea.
A partition has been placed between the LEDs and the photosensor for better visibility. Watch the completed demonstration on YouTube.