Challenge: Turn on the blue LED!
For this first Arduino exercise, I wired up three LEDs and three buttons in parallel. On my board, they are all mapped logically with the same color of button and LED next to each other. But when clicking, this mental mapping does not completely translate to the output. The red and yellow button work as we would expect them to and turn on the red and yellow LED respectively. The blue button, however, turns on both the red and yellow LEDs but not the blue one. How then can we turn on the blue LED?
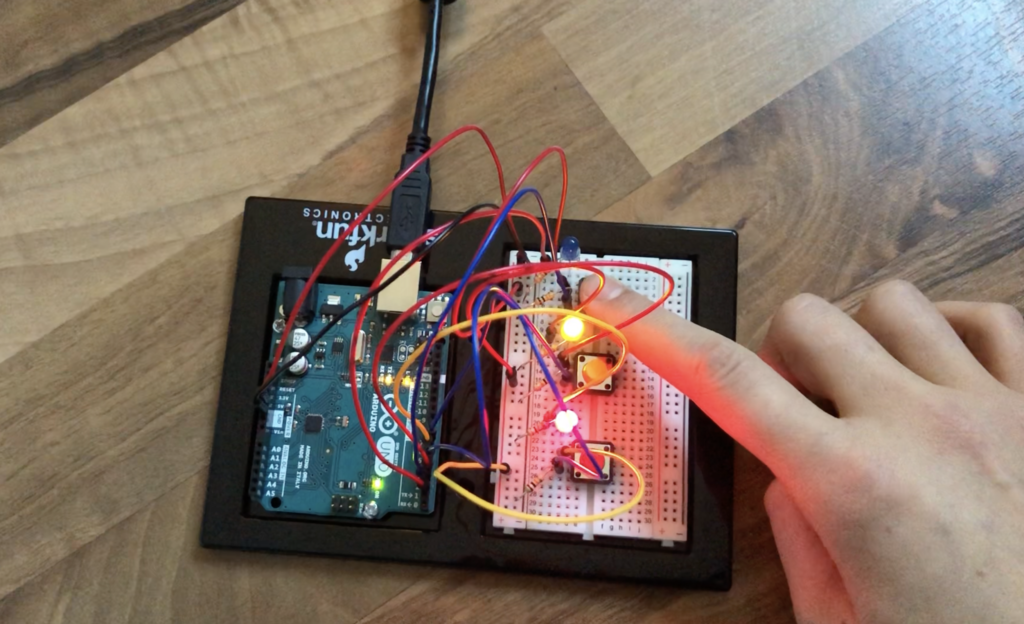
int blueledPin = 2; int bluebuttonPin = 3; int yellowledPin = 4; int yellowbuttonPin = 5; int redledPin = 6; int redbuttonPin = 7; void setup() { //BLUE button + LED pinMode(blueledPin, OUTPUT); pinMode(bluebuttonPin, INPUT); Serial.begin(9600); //YELLOW button + LED pinMode(yellowledPin, OUTPUT); pinMode(yellowbuttonPin, INPUT); Serial.begin(9600); //RED button + LED pinMode(redledPin, OUTPUT); pinMode(redbuttonPin, INPUT); Serial.begin(9600); } void loop() { //BLUE button triggers RED and YELLOW LED int bluebuttonState = digitalRead(bluebuttonPin); Serial.println(bluebuttonPin); digitalWrite(redledPin, bluebuttonState); digitalWrite(yellowledPin, bluebuttonState); //YELLOW button triggers YELLOW LED int yellowbuttonState = digitalRead(yellowbuttonPin); Serial.println(yellowbuttonPin); digitalWrite(yellowledPin, yellowbuttonState); //RED button triggers RED LED int redbuttonState = digitalRead(redbuttonPin); Serial.println(redbuttonPin); digitalWrite(redledPin, redbuttonState); //if YELLOW and RED button pressed turn on BLUE LED if (redbuttonState == yellowbuttonState) { digitalWrite(blueledPin, redbuttonState); } }
Solution: Pressing the red and yellow button together will turn on the blue LED.