As I was brainstorming for this exercise, I came across a recreation of Joy Division’s Unknown Pleasures album cover that incorporates looping and the noise function to animate the same mountain-like structure.
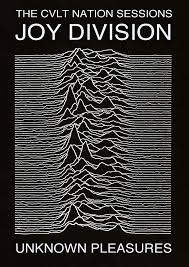
This led me to think of the album art of one of my favorite artists, Tame Impala. I looked some of the artwork up and it felt like something I could recreate using geometric shapes, loops, and the noise function.
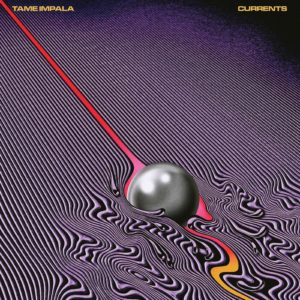
I recalled the lyric video for “Feels Like We Only Go Backwards”, and this particular frame inspired me. I wanted to attempt to recreate it and add an element of animation or flow.
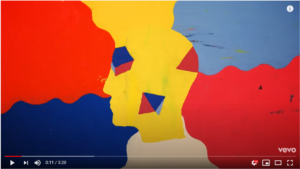
I thought: “this shouldn’t be too hard, right?” Weeeelll, I did struggle quite a bit with recreating the exact image I had in my head, so I will take you through my various attempts and takeaway from this assignment.
Attempt 1: Using Equidistant Lines in a Triangle Form:
My approach here was going through a loop and drawing multiple vertical lines close to each other in the form of a triangle and then apply noise() to each of these lines to create a stream that’s narrow and then widens near the edge of the screen.
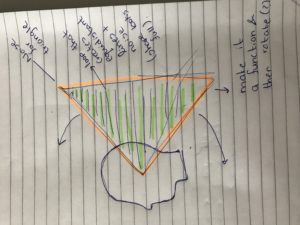
This didn’t work, as the noise function created a uniform movement where all the lines leaned together in one direction. Even when increasing and decreasing the input value to get more random movement, I didn’t get a smooth wave like I had imagined, the change in X was consistent for all the lines creating a beam-like movement rather than a wave.
Attempt 2: Using Points to Create a Diagonal Side
Then, inspired by this tutorial, I tried to take a different approach using Point(), where I draw the triangle sides as points and then apply the noise() function to the x value of each point. I used two variables for noise, the second one called xOffset which creates the animation.
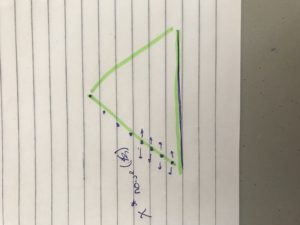
This way, I got closer to the movement I wanted, but it was still off, because I wanted the whole shape to flow as one, and this was too random to achieve that.
Finally, I decided that the most suitable approach would be similar to the one in the tutorial I linked earlier, to create a 4-sided shape (close to a rectangle or square) by drawing vertical equidistant lines through a loop and applying a noise() function to my X-value that takes a scaled Y for the first input, and an xOffset variable as the second input. This was the closest I got to my initial goal, but I think it could still be more accurate to the inspiration image.
This exercise really challenged my understanding of the thought process required for loops and the noise function, specifically how to choose values to input into the function. I went through our code in class and then followed any tutorials I could find on YouTube which helped make the concept more understandable. However, I still think that achieving my goal with this artwork could have been possible with the first two approaches I used, but it definitely requires more confidence with the concept of Noise(), so I might go back to these approaches after more practice.
Ironically, at some points, it really did feel like the more I tried the less progress I made (very in line with the song title), so it was a bit frustrating. Nonetheless, this exercise was a great way to work on my skills in using sketches and trial to translate an image I have in my head to code and to be patient and kind to myself when things don’t work out as well as I thought.
Have a look at my code, and make sure you go listen to Feels Like We Only Go Backwards!
color bgColor = color(240,245,230); color upperLeft = color(221, 28,26); color upperRight = color(111,156,235 ); color lowerLeft = color(7,42,200 ); color lowerRight = color( 249,240,0); float xOff = 0; float yOff =0; PImage img; void setup(){ size(400,400); //image from noun project, artist: Maxim Kulikov img = loadImage("noun_Head_2053552TWO.png"); } void draw(){ background(bgColor); // loop 1, upper left corner for(float y = 0; y<height/2; y++){ strokeWeight(4); stroke(upperLeft); //pushMatrix(); //translate(150,0); //rotate(radians(45)); // and attempt to make it look as if it is diagonally flowing out of the head. current shape looked better in my opinion. line(width/2*noise(y/100,xOff),y,width/2,y); //shifting the "wave" horizontally and decreasing opacity stroke(upperLeft,80); line(width/2*noise(y/100,xOff) - 50,y,width/2,y); stroke(upperLeft,60); line(width/2*noise(y/100,xOff) - 100,y,width/2,y); y +=1 ; } //loop2, upper right corner for(float y2 = 0; y2<height/2; y2++){ stroke(upperRight); line(width/2, y2, 200 + width/2*noise(y2/100,xOff),y2); stroke(upperRight,80); line(width/2, y2, 250 + width/2*noise(y2/100,xOff),y2); stroke(upperRight,60); line(width/2, y2, 300 + width/2*noise(y2/100,xOff),y2); y2 += 1; } //loop3, lower left corner for(float y3 = height/2; y3<height; y3++){ stroke(lowerLeft); line(width/2*noise(y3/100,xOff),y3,width/2,y3); stroke(lowerLeft,80); line(width/2*noise(y3/100,xOff) -50,y3,width/2,y3); stroke(lowerLeft,60); line(width/2*noise(y3/100,xOff)- 100,y3,width/2,y3); y3 +=1 ; } // loop 4, lower right corner for(float y4 = height/2; y4<height; y4++){ stroke(lowerRight); line(width/2, y4, 200 + width/2*noise(y4/100,xOff),y4); stroke(lowerRight,80); line(width/2, y4, 250 + width/2*noise(y4/100,xOff),y4); stroke(lowerRight,60); line(width/2, y4, 300 + width/2*noise(y4/100,xOff),y4); y4 += 1; } //incrementing the Y val of the noise function to create movement. xOff += 0.01; imageMode(CENTER); image(img, width/2, height/2); }