For my project, I decided to copy Ben F. Laposky’s sine wave Oscillons. Laposky created his original artwork starting in 1952, using oscillators, amplifiers, and modulators with a cathode ray tube to create what is generally regarded as the first computer art.
Since the image (presented here in both black and white and original) was created by taking pictures of analogue lines on a screen, I decided to simulate the motion of the original screen.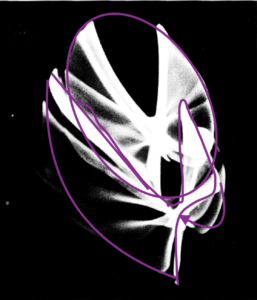
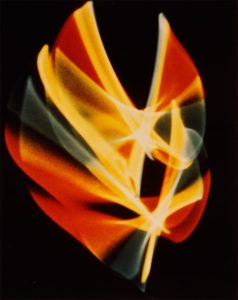
int t, x, y, x2, y2;
int a, b, b2, c, c2;
int d, e, e2, f, f2;
int w = 200;
float speed = .03;
float speed2 = .01;
float granulation = .0005;
float radius = 100;
void setup(){
size (480,640);
t = 0;
}
void draw(){
float frequency = (frameCount*speed) + (granulation);
float fillColor = map(noise(frequency), .01, .75, 0, 255);
float fillColor2 = map(sin(frequency), .01, .75, 0, 255);
float fillColor3 = map(cos(sin(frequency)), .01, .75, 0, 255);
fill(0,0,0,11);
rectMode(CORNER);
rect(-10,-10,width+20,height+20); // fade part
rectMode(CENTER);
//fill(100, 200, 3, fillColor);
stroke(fillColor, fillColor2, 3,fillColor);
strokeWeight(12);
pushMatrix();
float t= frameCount*speed;
float x = radius * sin(t);
float y = radius * cos(t/8);
float x2 = radius/2 * sin(t-3);
float y2 = radius * cos(t);
translate(width/2,height/2);
line(x,y,x2,y2);
//popMatrix();
stroke(fillColor2, fillColor, fillColor,fillColor);
strokeWeight(12);
//pushMatrix();
float a= frameCount*1.1*speed;
float c = radius * sin(a);
float b = radius * cos(a/2);
float c2 = radius * sin(a*1.3);
float b2 = radius * cos(a/3);
//translate(width/2,height/2);
line(c,b,c2,b2);
stroke(fillColor3, fillColor3, fillColor2,fillColor);
strokeWeight(12);
//pushMatrix();
float d= frameCount*1.1*speed2;
float e = radius * sin(d);
float f = 20+ radius * cos(d/1.8);
float e2 = radius * sin(d/3);
float f2 = radius * cos(d/3);
//translate(width/2,height/2);
line(e,f,e2,f2);
// float c = radius * sin(a(tan(a));
//float b = radius * cos(a/2);
//float c2 = radius * sin(a-3)*tan(a);
//float b2 = radius * cos(a/3);
////translate(width/2,height/2);
// line(c,b,c2,b2);
popMatrix();
}
To simulate the machine used to create the images, I decided to use bars or lines, making them mobile by having their endpoints following certain sin and cosine functions. I used a Trick Aaron taught me to blur it. The other main function of my code makes each bar change color within a certain specified range, either using noise or a sin/cos wave to change the values.
Once I figured this out, Most of what I had to do was play with where each bar was, what color it was, the speed, and blur.
link
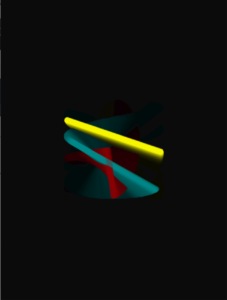
int t, x, y, x2, y2;
int a, b, b2, c, c2;
int d, e, e2, f, f2;
int w = 200;
float speed = .03;
float speed2 = .01;
float granulation = .0005;
float radius = 100;
void setup(){
size (480,560);
t = 0;
}
void draw(){
float frequency = (frameCount*speed) + (granulation);
float fillColor = map(noise(frequency*2), .01, .75, 190, 255);
float fillColor2 = map(sin(frequency), .01, .75, 190, 220);
float fillColor3 = map(cos(sin(frequency)), .01, .75, 0, 255);
fill(0,0,0,11);
rectMode(CORNER);
rect(-10,-10,width+20,height+20); // fade part
rectMode(CENTER);
//fill(100, 200, 3, fillColor);
stroke(fillColor, fillColor2, random(20),fillColor);
//stroke(200, 200, 3,fillColor);//test colors
strokeWeight(12);
pushMatrix();
float t= frameCount*speed;
float x = (radius * -cos(t))+100;
float y = (radius * sin(t))+100;
float x2 = radius * cos(t);
float y2 = radius * -sin(t);
translate(width/2+20,height/2+-100);
line(x,y,x2,y2);
//popMatrix();
stroke(fillColor, fillColor2, 3,fillColor);
strokeWeight(12);
//pushMatrix();
float a= frameCount*speed;
float c = (radius * -sin(a))+100;
float b = (radius * cos(a))+100;
float c2 = radius * sin(a);
float b2 = radius * -cos(a);
//translate(width/2+20,height/2-100);
line(c,b,c2,b2);
// stroke(fillColor3, fillColor3, fillColor2,fillColor);
// strokeWeight(12);
// //pushMatrix();
// float d= frameCount*1.1*speed2;
// float e = radius * sin(d);
// float f = 20+ radius * cos(d/1.8);
// float e2 = radius * sin(d/3);
// float f2 = radius * cos(d/3);
// //translate(width/2,height/2);
// line(e,f,e2,f2);
// float c = radius * sin(a(tan(a));
//float b = radius * cos(a/2);
//float c2 = radius * sin(a-3)*tan(a);
//float b2 = radius * cos(a/3);
////translate(width/2,height/2);
// line(c,b,c2,b2);
popMatrix();
}
Finally it was time for me to begin to copy the original image.
Here in this code, I have two systems working to create the first butterfly in the image. The color fluctuates within the spectrum from red/orange to yellow/green. What I need now is a second system, and possibly a way to translate it up and down as time goes in order to get the same quality as the original.
If
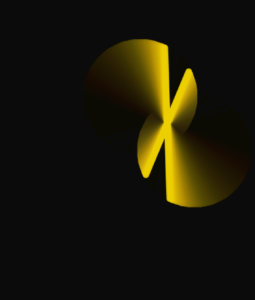