For my midterm project, I wanted to create something that had a defined storyline as the main component pulling the user in. After struggling to decide on whether to go for an interactive experience or a game, I went for the latter. My hope is to create a gamified storyline that takes the user through a beginning, middle, and end. Seeing as I have remained faithful to the butterfly motif for quite some time now, I decided it would be a shame to break the streak with this assignment.
Design and Concept
For the main design of the game, I was inspired by games like Super Mario, the offline Chrome Dinosaur Game, and also the feel of a retro game design in this work by Arshiya Khattak. The main storyline is to help a butterfly find her way home in the open fields after getting lost in a city full of high-rise skyscrapers, with no flowers in sight. The game starts with the scene of a butterfly fluttering through the main character’s window. After the scene ends, a prompt is displayed with directions on how to play the forthcoming game. For now, there will be two main levels, the first being easier than the second. The character, carrying the butterfly on her back, would have to jump over obstacles or enemy objects to maintain her health status. She would also be presented with the opportunity to regain some of her health back by collecting rewards on the way. If the character manages to reach the end without losing all of her health, the ending game scene is played in which the butterfly is reunited with her family in a field of flowers and a prompt is displayed congratulating the player with instructions on how to repeat the game. In the process of visualizing this, I sketched out the following storyboard:
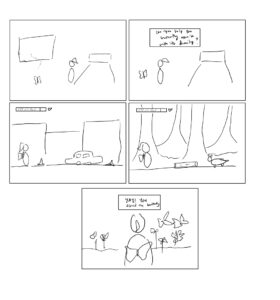
Code Design
To make my code easier to design and scale up, I plan to encapsulate everything, including the game experience itself in a class. Therefore, it was helpful to sketch out class diagrams in a UML-ish fashion as follows:
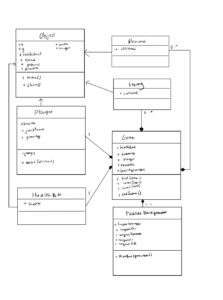
Anticipated Complexities/Challenges and Risk Mitigation
My strategy for mitigating risk was to implement the basic skeleton of the game levels to allow me to seamlessly scale up complexity later on. I also wanted to get the most time-consuming aspects of the game design, like collecting assets, out of the way so that I could focus on the game functionality this upcoming week.
Asset Collection
I knew one of the things I would struggle with was finding unified assets that were consistent with the feel of the game I was looking for. Thus, I dedicated some time to collecting spritesheets and level/scene backgrounds as I wanted to minimize having to design most components myself. After some digging, I collated the following:
Sprites:
(https://opengameart.org/content/oracles)
(https://opengameart.org/content/butterfly)
Backgrounds:
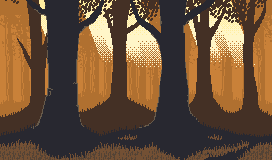
(https://opengameart.org/content/forest-background)
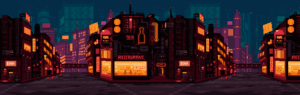
(https://opengameart.org/content/cyberpunk-street-environment)
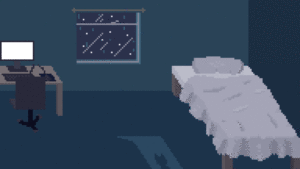
(https://www.deviantart.com/watashi-sensei/art/Bedroom-Pixel-628248413)
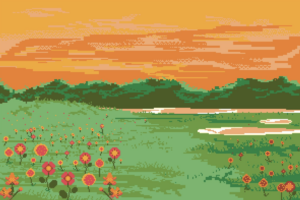
(https://www.freepik.com/free-vector/pixel-art-rural-landscape-background_49685498.htm#from_view=detail_serie)
Parallax Background Implementation
For the background of the levels, I wanted to incorporate a parallax effect to add dynamism to the backdrop of the experience. It has been some time since I have implemented this and I had forgotten the basic principles, so I wanted to get this part out of the way first. Each background is composed of multiple layers and the basic idea is to have each layer loop back into the screen at varying speeds to create a continuous animation with different depth components.
class ParallaxBackground {
constructor(layersImages, layersSpeeds, layersDir) {
this.layersImages = layersImages;
this.layersDir = layersDir;
this.layersSpeeds = layersSpeeds;
this.layersX1 = [];
this.layersX2 = [];
for (let i = 0; i < this.layersImages.length; i++) {
this.layersX1.push(0);
this.layersX2.push(width);
}
print(this.layersSpeeds);
}
showBackground() {
for (let i = 0; i < this.layersImages.length; i++) {
image(this.layersImages[i], this.layersX1[i], 0, width, height);
image(this.layersImages[i], this.layersX2[i], 0, width, height);
if (this.layersDir[i] == 1) {
this.layersX1[i] -= this.layersSpeeds[i];
this.layersX2[i] -= this.layersSpeeds[i];
if (this.layersX1[i] < -width) {
this.layersX1[i] = width;
}
if (this.layersX2[i] < -width) {
this.layersX2[i] = width;
}
}
}
}
}
Sprite Movements and Collisions
I also wanted to simulate the movement of objects on the screen to get a headstart. I used placeholder ellipses for this process, which I will later replace with actual images. I utilized principles of velocity and acceleration to simulate the player’s vertical jumps upon the space bar key press and the movement of enemies in the horizontal direction across the screen:
class Player {
constructor(x, y, ground) {
this.x = x;
this.y = y;
this.vy = 0;
this.ground = ground;
this.gravity = 1;
this.jumpPower = 20;
this.collisions = 0;
this.collidedEnemy = [];
}
move() {
this.y += this.vy;
// player is not on ground
if (this.y < this.ground) {
this.vy += this.gravity;
} else {
this.vy = 0;
this.y = this.ground;
}
}
jump() {
if (this.y >= this.ground) {
this.vy = -this.jumpPower;
}
}
show() {
ellipse(this.x, this.y, 50, 100);
this.move();
}
}
class Enemy {
constructor(x, y) {
this.x = x;
this.y = y;
this.vx = -5;
this.collided = false;
}
move() {
this.x += this.vx;
}
show() {
ellipse(this.x, this.y, 50, 50);
this.move();
}
}
I also wanted to implement the collision mechanism by essentially detecting when the boundary of the player touches the boundary of an object and classifying it as a collision:
detectCollision(enemy) {
let radius = 10;
if (!enemy.collided) {
// get distance betweem the center of the character and that of the enemy object
let d = dist(this.x, this.y, enemy.x, enemy.y);
// collision detected
// distance is less than the sum of objects' radii
// and the radius for collisioon
if (d < radius + 25 + 25) {
this.collisions += 1;
enemy.collided = true;
return true;
}
}
else{
return false;
}
}
Current Progress