In synthesizing the insights from TIGOE’s blog posts, “Physical Computing’s Greatest Hits (and misses)” and “Making Interactive Art: Set the Stage, Then Shut Up and Listen,” there emerges a compelling narrative about the nature of human interaction with technology and the role of the creator in guiding this interaction.
The first post examines the myriad ways in which creators harness technology to bridge the gap between art and the human experience. It underscores that the essence of physical computing is not in the machinery itself, but in the potential for human expression and interaction that it affords. TIGOE celebrates the diversity of approaches—from instruments that translate motion into sound to devices that sense and react to our emotions—each offering a unique dialogue between user and machine. This celebration is not just of the end product but of the process and potential for evolution, highlighting that even familiar ideas can be reinvigorated through creative reinterpretation.
The second post, meanwhile, delves into the philosophy behind interactive art. It champions the idea that art is a collaborative exchange and urges creators to step back after setting the scene, allowing the audience to bring their interpretations and experiences into play. This stance challenges the traditional static relationship between art and observer, proposing instead a dynamic interaction where the audience becomes an integral part of the art’s essence.
Both readings converge on the point that in the realm of interactive experiences, whether through physical computing or art, the creator’s role is not to dictate but to facilitate. It’s an invitation to viewers to engage, explore, and co-create, leading to a richer tapestry of experiences. The pieces do not stand as mere displays of technological or artistic prowess but as starting points for a journey that each participant completes in their own unique way.
By bringing together the physical and the interactive, TIGOE illuminates a future of creation that is ever-evolving, deeply personal, and universally accessible. In this future, the boundaries between the creator, the creation, and the consumer are not just blurred but are actively redefined with each interaction, fostering a space where art and technology serve as mediums for communication, learning, and exploration of the human condition. Both readings serve as a manifesto for the modern maker, encouraging a dialogue with technology and audience that is as open-ended as it is profound.
Blinding lights:
After being inspired by The Weeknd’s album ‘After Hours,’ I chose to name my assignment ‘Blinding Lights,’ which reflects the influence of his music had on me. 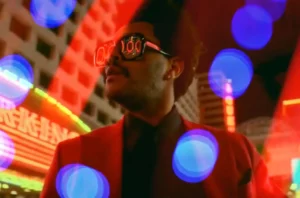
https://youtube.com/shorts/uRrlVS0TPpw?feature=shared
This Arduino code controls two LEDs (red and blue) based on the light detected by a photoresistor and the position of a potentiometer. The LEDs alternate based on the light level: if it’s dark (light level below 300), they alternate, with the speed of alternation controlled by the potentiometer. If it’s light, both LEDs remain off. The program uses serial output to display the light level detected by the photoresistor.
const int led0 = 3; // Red LED
const int led1 = 5; // Blue LED
const int photoResistor = A1;
const int potentiometer = A0; // Potentiometer connected to analog pin A0
void setup() {
Serial.begin(9600);
pinMode(led0, OUTPUT);
pinMode(led1, OUTPUT);
}
void loop() {
int lightValue = analogRead(photoResistor);
Serial.print("Light Value: ");
Serial.println(lightValue);
// Read the potentiometer value to control the speed of LED alternation.
int potValue = analogRead(potentiometer);
int delayTime = map(potValue, 0, 1023, 50, 1000); // Adjust this range as needed.
if (lightValue < 300) { // Threshold for photoresistor touch
// Alternate LEDs when the photoresistor is touched.
digitalWrite(led0, HIGH);
digitalWrite(led1, LOW);
delay(delayTime); // Delay controlled by potentiometer
digitalWrite(led0, LOW);
digitalWrite(led1, HIGH);
delay(delayTime); // Delay controlled by potentiometer
} else {
// If the photoresistor is not being touched, turn both LEDs off or keep them in a default state.
digitalWrite(led0, LOW);
digitalWrite(led1, LOW);
}
}