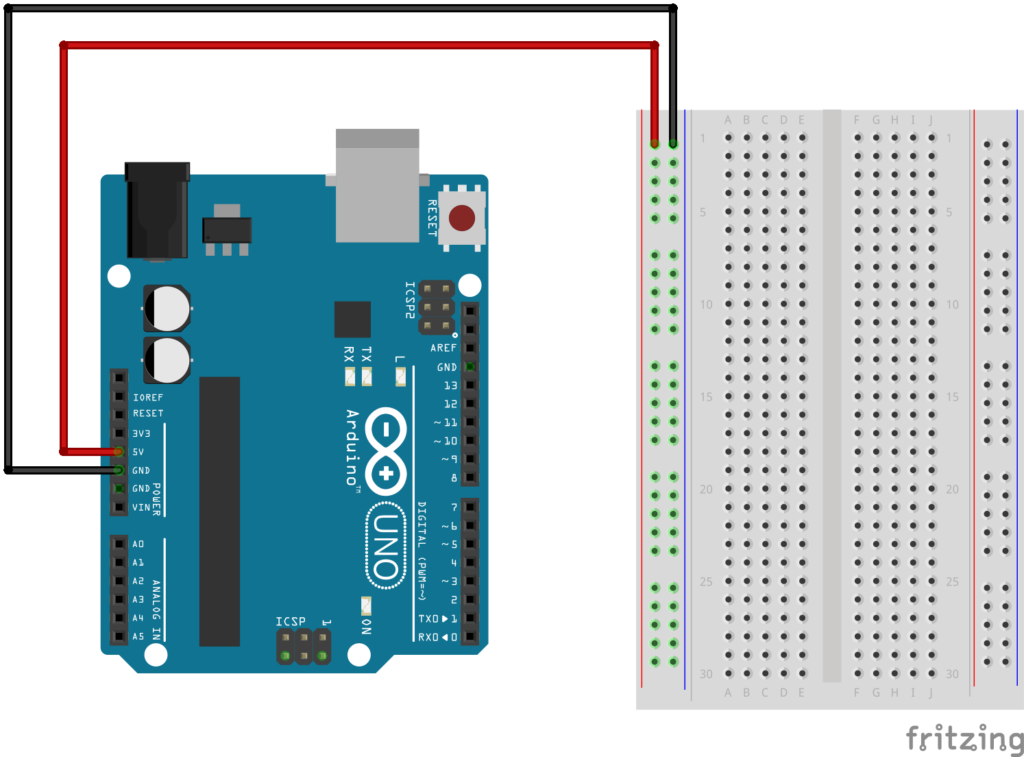
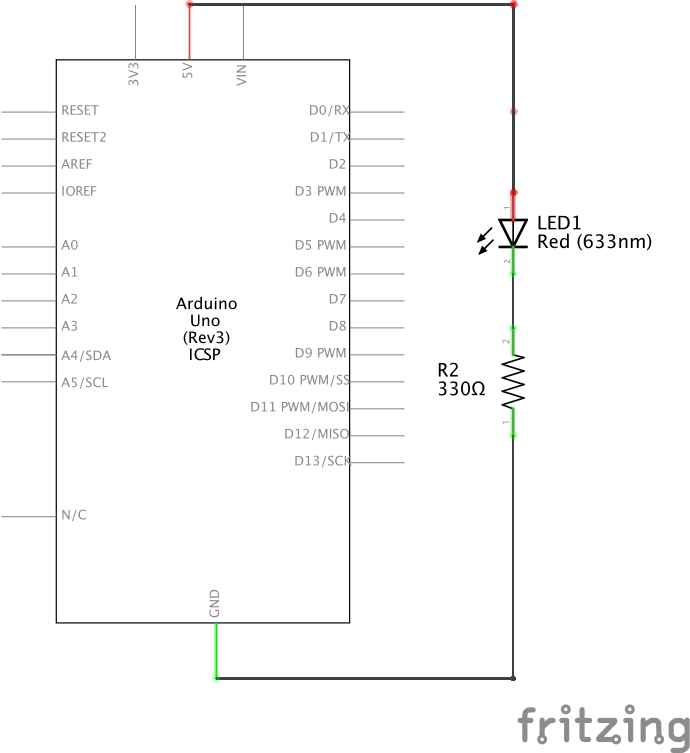
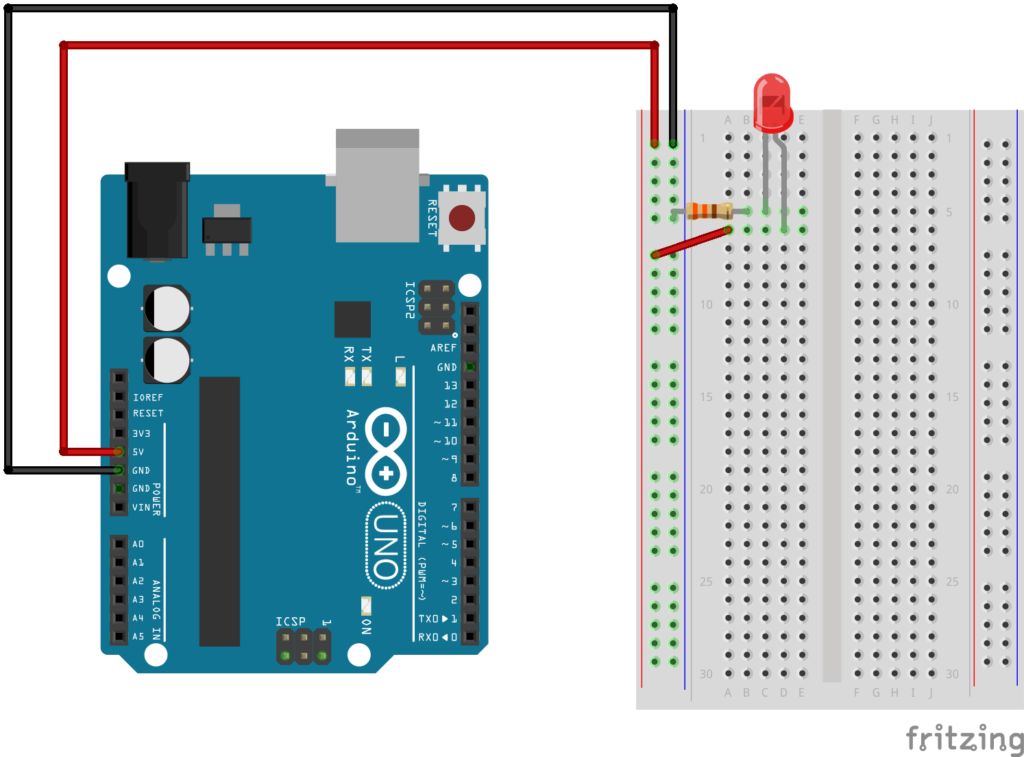
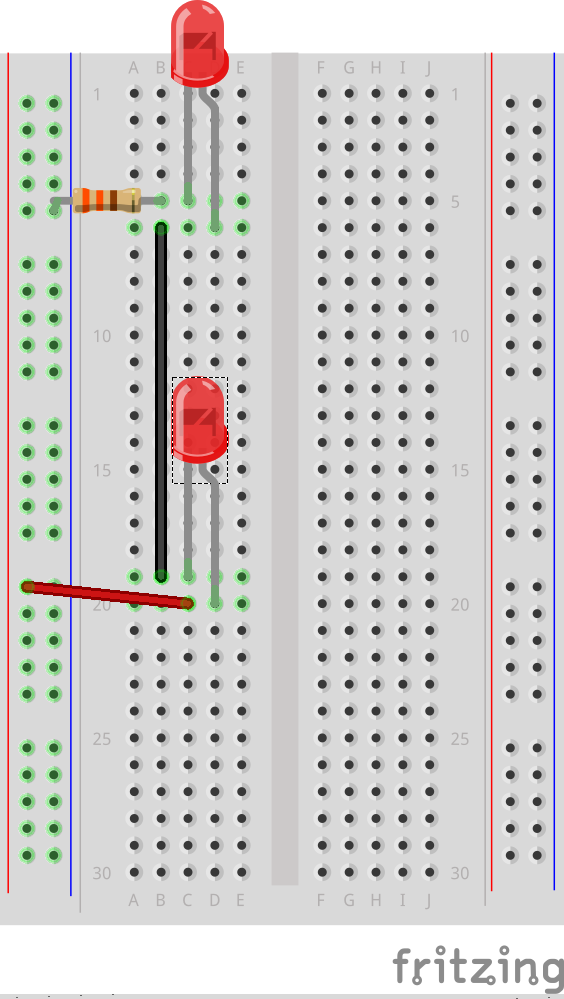
Series
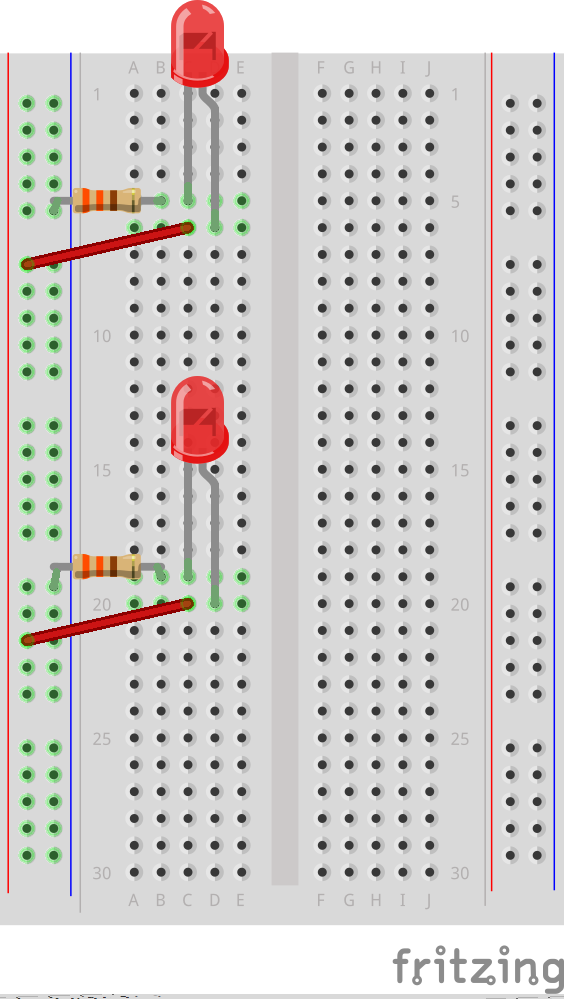
Parallel
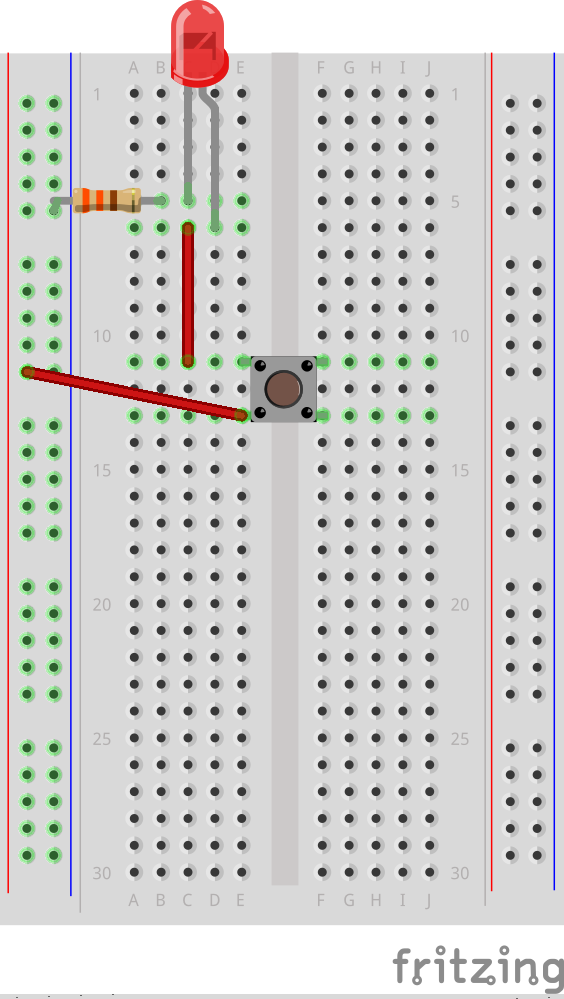
Button
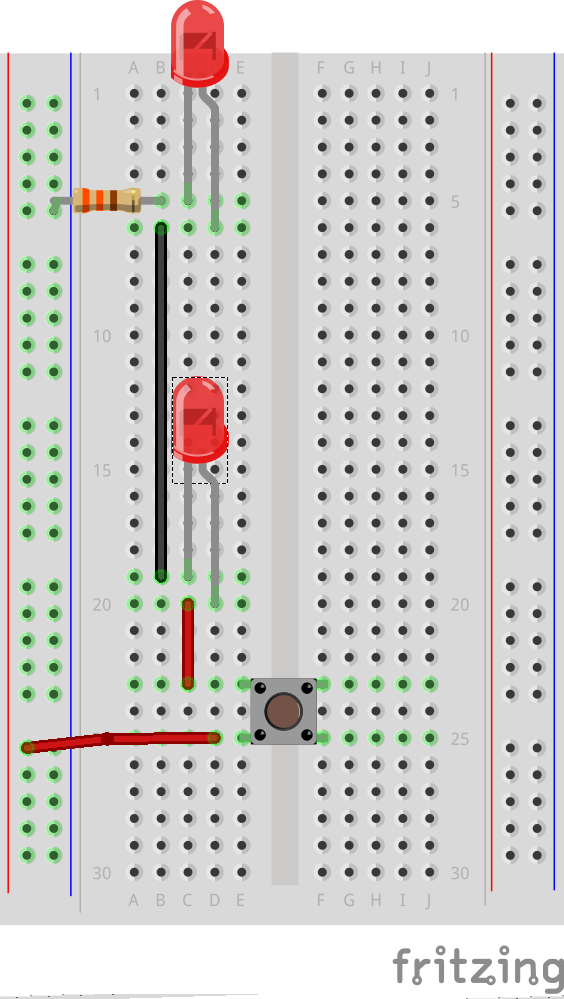
Series with Button
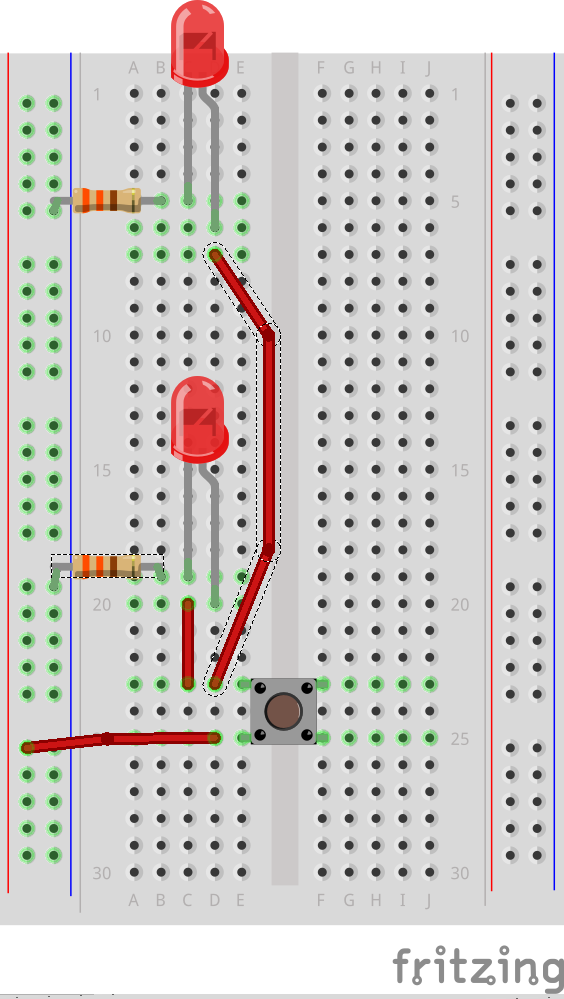
Parallel with Button
Series
Parallel
Button
Series with Button
Parallel with Button
And here’s the code from class to prove it
Here’s the code from class
Here’s what we covered in the first class with Processing:
Turning a transistor on and off is just a matter of applying voltage.
Use the Blink example code to turn the motor on and off every second, or try the Fade example to vary its speed.
Here’s the wiring for a p2n2222 transistor (the kind you have in your kit) :
Below is the schematic for a TIP120 transistor (the kind we have in the lab) :
A H-bridge (fund in the lab, not in your kit) allows you to turn the motor in either direction. Use the code below with this schematic :
const int motor1Pin = 9; // H-bridge leg 1 (pin 2, 1A) const int motor2Pin = 8; // H-bridge leg 2 (pin 7, 2A) void setup() { // set pins you're using as outputs: pinMode(motor1Pin, OUTPUT); pinMode(motor2Pin, OUTPUT); } void loop() { // turn the motor in one direction: digitalWrite(motor1Pin, LOW); // set leg 1 of the H-bridge low digitalWrite(motor2Pin, HIGH); // set leg 2 of the H-bridge high delay(1000); // wait for a second // stop the motor spinning : digitalWrite(motor1Pin, LOW); // set leg 1 of the H-bridge low digitalWrite(motor2Pin, LOW); // set leg 2 of the H-bridge high delay(1000); // wait for a second // turn the motor in the other direction : digitalWrite(motor1Pin, HIGH); // set leg 1 of the H-bridge low digitalWrite(motor2Pin, LOW); // set leg 2 of the H-bridge high delay(1000); // wait for a second // stop the motor spinning : digitalWrite(motor1Pin, LOW); // set leg 1 of the H-bridge low digitalWrite(motor2Pin, LOW); // set leg 2 of the H-bridge high delay(1000); // wait for a second }
For inspiration of different gears and things, look at http://robives.com/mechs and http://robives.com/techniques
We were talking last time about how awesome computers are at reconfiguring themselves to do different things. This was manifest in pins on the microcontroller that we could change the behavior of through software.
What we were lacking in this model though, something that we possess, is a sense of memory (regret, if you must). Our programs so far forget things as soon as we’re done with them. It’s not a very efficient method, like buying a new water bottle everytime you wanted a drink. Instead, let’s save the planet and use something over and over again. In programming, the tool we can use for this is a variable.
While you’ve successfully managed to leave the lights on, you’ve so far been limited in that you have only been able to do one thing at a time. With software, we can do multiple things (or at least have the appearance of multiple things) happening.
Software is the realization of Alan Turing’s universal machine. In short, this is a computational machine that can replicate any other computational machine after being fed a set of instructions that indicate the computation to go through and how the machine is set up to do this. Through software, we can reconfigure a machine to do anything we darn well please, including teaching the machine to behave like a different machine (see also, emulators).
Computers are great adding machines. They’re also great at comparing things that usually don’t work well together. Jim Campbell has a nice animation about this. We can get away from the mouse, monitor, keyboard (and now ubiquitous touchscreen) to think differently about these machines we use all the time.
At the end of the day, all we’re doing here is wrangling electrons. They want to move from a place of higher potential energy to a place of lower potential energy (we call this ground). The bus falling off the side of a cliff metaphor is generally a good idea of how we can think of these things moving through a circuit.