Concept:
GripSense is designed to empower recovery and build strength through simple, intuitive grip exercises. Inspired by the resilience required in rehabilitation, it is designed with individuals in mind who are recovering from hand injuries, surgeries, or conditions like arthritis that can limit hand mobility and grip strength. The idea is to create a device that not only helps measure improvement over time but also motivates users to engage in strength exercises by giving real-time feedback and adapting to different levels of force.
Work Procedure:
A squishy ball, embedded with Force Sensor, connected to 5V and Analog Pin A0 on the Arduino through a 1kΩ resistor to ground. This creates a voltage divider, enabling the Arduino to detect varying pressure levels. For feedback, two LEDs are connected: a Light Touch LED on Pin 8 with a 310Ω resistor for gentle squeezes, and a Strong Force LED on Pin 10 with a 310Ω resistorfor firmer grips. Each LED lights up based on the detected force level, providing immediate, intuitive feedback on grip strength.
The Arduino reads these values and provides real-time feedback through LEDs:
- Light Touch LED (Blue): Lights up for a gentle squeeze ensuring the sensor is working.
- Strong Force LED (Yellow): Lights up for a firmer, stronger grip.
Code:
const int forceSensorPin = A0; // Pin connected to the force sensor const int lightTouchLED = 8; // Pin for the first LED (light touch) const int strongForceLED = 10; // Pin for the second LED (strong force) int forceValue = 0; // Variable to store the sensor reading int lightTouchThreshold = 60; // Threshold for light touch int strongForceThreshold = 300; // Threshold for strong force void setup() { Serial.begin(9600); // Initialize serial communication at 9600 bps pinMode(lightTouchLED, OUTPUT); // Set LED pins as outputs pinMode(strongForceLED, OUTPUT); } void loop() { // Read the analog value from the force sensor forceValue = analogRead(forceSensorPin); // Print the value to the Serial Monitor Serial.print("Force Sensor Value: "); Serial.println(forceValue); // Check for strong force first to ensure it takes priority over light touch if (forceValue >= strongForceThreshold) { digitalWrite(lightTouchLED, LOW); // Turn off the first LED digitalWrite(strongForceLED, HIGH); // Turn on the second LED } // Check for light touch else if (forceValue >= lightTouchThreshold && forceValue < strongForceThreshold) { digitalWrite(lightTouchLED, HIGH); // Turn on the first LED digitalWrite(strongForceLED, LOW); // Ensure the second LED is off } // No touch detected else { digitalWrite(lightTouchLED, LOW); // Turn off both LEDs digitalWrite(strongForceLED, LOW); } delay(100); // Small delay for stability }
Schematic Diagram:
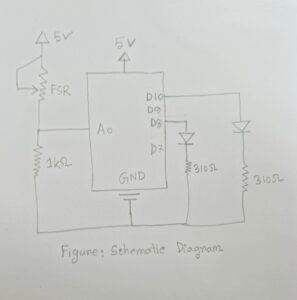
Media:
Reflection and Future Improvements:
In the future, I plan to enhance GripSense with several key upgrades:
- Secure Sensor Integration: I’ll embed the force sensor inside the squish ball or design a custom 3D-printed casing to hold both the ball and sensor. This will make the setup more professional, durable, and comfortable for users.
- Add OLED Display for Instant Feedback: I want to incorporate an OLED screen that will show live grip strength data, allowing users to see their strength level and progress instantly with each squeeze.
- Implement Multi-Level LED Indicators: To make feedback more visual, I’ll add a series of LEDs that light up at different levels of grip strength. For example, green will represent light grip, yellow for medium, and red for strong, giving users an intuitive, color-coded response.
- Data Logging for Progress Tracking: I aim to add an SD card module to log grip strength data over time, stored in a CSV format. This feature will enable users to track their improvement and analyze their progress with tangible data.
- Enable Bluetooth or Wi-Fi Connectivity: I plan to incorporate wireless connectivity so that users can view their grip data on a smartphone app. This will allow them to track progress visually with graphs, set goals, and receive real-time feedback, making the recovery journey more engaging and motivating.
These upgrades will help make GripSense a comprehensive, interactive tool that supports and inspires users through their rehabilitation process.