Task 1
Prompt: Make something that uses only one sensor on Arduino and makes the ellipse in p5 move on the horizontal axis, in the middle of the screen, and nothing on Arduino is controlled by p5.
We decided to approach all the tasks following Christmas style.
P5 Code:
// maping the values from light sensor to the width of the canvas
ellipse(map(positionX, 0, 1023, 0, width), height / 2, 100, 100);
// the initial screen of p5, when it is not connected to arduino, this helps to start serial communication
text("Press Space Bar to select Serial Port", 20, 30, width - 30, 200);
// starting serial communication when space bar is pressed
if (key == " ") setUpSerial();
function readSerial(data) {
// ensuring there is actual data, then storing it in a variable
let positionX = 0;
function setup() {
createCanvas(600, 600);
noFill();
}
function draw() {
background("#bb010b");
stroke("#ffcf00");
// maping the values from light sensor to the width of the canvas
ellipse(map(positionX, 0, 1023, 0, width), height / 2, 100, 100);
if (!serialActive) {
// the initial screen of p5, when it is not connected to arduino, this helps to start serial communication
background("#2d661b");
stroke("#white");
textSize(50);
text("Press Space Bar to select Serial Port", 20, 30, width - 30, 200);
}
}
function keyPressed() {
// starting serial communication when space bar is pressed
if (key == " ") setUpSerial();
}
function readSerial(data) {
if (data != null)
// ensuring there is actual data, then storing it in a variable
positionX = int(data);
}
let positionX = 0;
function setup() {
createCanvas(600, 600);
noFill();
}
function draw() {
background("#bb010b");
stroke("#ffcf00");
// maping the values from light sensor to the width of the canvas
ellipse(map(positionX, 0, 1023, 0, width), height / 2, 100, 100);
if (!serialActive) {
// the initial screen of p5, when it is not connected to arduino, this helps to start serial communication
background("#2d661b");
stroke("#white");
textSize(50);
text("Press Space Bar to select Serial Port", 20, 30, width - 30, 200);
}
}
function keyPressed() {
// starting serial communication when space bar is pressed
if (key == " ") setUpSerial();
}
function readSerial(data) {
if (data != null)
// ensuring there is actual data, then storing it in a variable
positionX = int(data);
}
Arduino Code
pinMode(lightPin, INPUT);
int sensorValue = analogRead(A0);
Serial.println(sensorValue);
int lightPin = A0;
void setup() {
Serial.begin(9600);
pinMode(lightPin, INPUT);
}
void loop() {
int sensorValue = analogRead(A0);
Serial.println(sensorValue);
delay(5);
}
int lightPin = A0;
void setup() {
Serial.begin(9600);
pinMode(lightPin, INPUT);
}
void loop() {
int sensorValue = analogRead(A0);
Serial.println(sensorValue);
delay(5);
}
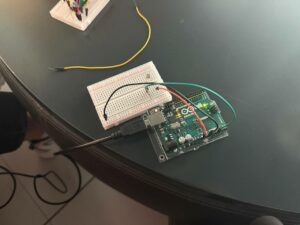
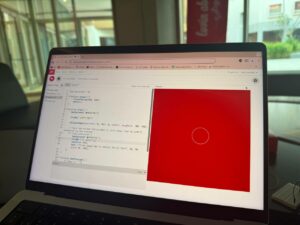
Task 2
Prompt: Make something that controls the LED brightness from p5
For this assignment, we decided to control LED brightness using 4 circles/buttons on p5 interface. By pressing different circles, you increased the brightness of LED.
P5 Code
circle (150, height/2, 50);
circle (250, height/2, 50);
circle (350, height/2, 50);
circle (450, height/2, 50);
// to understand whether user pressed specific circles, we used distance function. the LED brightness had 4 variations
if (dist (mouseX, mouseY, 150, height/2) <= 50){
if (dist(mouseX, mouseY, 250, height/2)<=50){
if (dist(mouseX, mouseY, 350, height/2)<=50){
if (dist(mouseX, mouseY, 450, height/2)<=50){
// to understand if serial communication is happening
text ("Press Space Bar to select Serial Port", 20, 30, width-30, 200);
// starting the serial connection using space bar press
function readSerial(data) {
//sending data to arduino
writeSerial(LEDbrightness);
let LEDbrightness = 0;
function setup() {
createCanvas(600, 600);
background('#2d661b');
stroke ('#white');
noFill();
}
function draw() {
if (serialActive){
//circles-buttons
circle (150, height/2, 50);
circle (250, height/2, 50);
circle (350, height/2, 50);
circle (450, height/2, 50);
// to understand whether user pressed specific circles, we used distance function. the LED brightness had 4 variations
if (dist (mouseX, mouseY, 150, height/2) <= 50){
LEDbrightness = 60;
}
if (dist(mouseX, mouseY, 250, height/2)<=50){
LEDbrightness = 120;
}
if (dist(mouseX, mouseY, 350, height/2)<=50){
LEDbrightness = 180;
}
if (dist(mouseX, mouseY, 450, height/2)<=50){
LEDbrightness = 255;
}
}
if (!serialActive) {
// to understand if serial communication is happening
textSize(50);
text ("Press Space Bar to select Serial Port", 20, 30, width-30, 200);
}
}
function keyPressed() {
if (key == " ")
// starting the serial connection using space bar press
setUpSerial();
}
function readSerial(data) {
//sending data to arduino
writeSerial(LEDbrightness);
}
let LEDbrightness = 0;
function setup() {
createCanvas(600, 600);
background('#2d661b');
stroke ('#white');
noFill();
}
function draw() {
if (serialActive){
//circles-buttons
circle (150, height/2, 50);
circle (250, height/2, 50);
circle (350, height/2, 50);
circle (450, height/2, 50);
// to understand whether user pressed specific circles, we used distance function. the LED brightness had 4 variations
if (dist (mouseX, mouseY, 150, height/2) <= 50){
LEDbrightness = 60;
}
if (dist(mouseX, mouseY, 250, height/2)<=50){
LEDbrightness = 120;
}
if (dist(mouseX, mouseY, 350, height/2)<=50){
LEDbrightness = 180;
}
if (dist(mouseX, mouseY, 450, height/2)<=50){
LEDbrightness = 255;
}
}
if (!serialActive) {
// to understand if serial communication is happening
textSize(50);
text ("Press Space Bar to select Serial Port", 20, 30, width-30, 200);
}
}
function keyPressed() {
if (key == " ")
// starting the serial connection using space bar press
setUpSerial();
}
function readSerial(data) {
//sending data to arduino
writeSerial(LEDbrightness);
}
Arduino Code
analogWrite(5, Serial.parseInt());
void setup() {
Serial.begin(9600);
pinMode(5, OUTPUT);
}
void loop() {
analogWrite(5, Serial.parseInt());
Serial.println();
}
void setup() {
Serial.begin(9600);
pinMode(5, OUTPUT);
}
void loop() {
analogWrite(5, Serial.parseInt());
Serial.println();
}
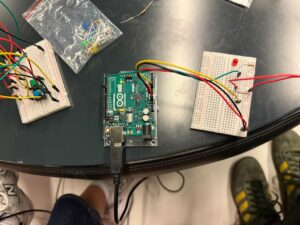
IMG_9258
Task 3
Prompt: Take the gravity wind example and make it so every time the ball bounces one led lights up and then turns off, and you can control the wind from one analog sensor.
We decided to use light sensor as analog sensor, which controlled the wind on p5 that affected movement of ball.
P5 Code
let position, velocity, acceleration, gravity, wind;
position = createVector(width / 2, 0);
velocity = createVector(0, 0);
acceleration = createVector(0, 0);
gravity = createVector(0, 0.5 * mass);
wind = createVector(0, 0);
text("Press F to select Serial Port", 20, 30, width - 30, 200);
velocity.add(acceleration);
ellipse(position.x, position.y, mass, mass);
if (position.y > height - mass / 2) {
velocity.y *= -0.9; // dampening
position.y = height - mass / 2;
if (!hasBounced && abs(velocity.y) > 1) {
setTimeout(() => (hasBounced = false), 100);
function applyForce(force) {
let f = p5.Vector.div(force, mass);
position.set(width / 2, -mass);
function readSerial(data) {
let value = int(trim(data));
wind.x = map(value, 0, 1023, -1, 1);
writeSerial(bounceState + "\n");
let position, velocity, acceleration, gravity, wind;
let drag = 0.99,
mass = 50,
hasBounced = false;
function setup() {
createCanvas(640, 360);
noFill();
position = createVector(width / 2, 0);
velocity = createVector(0, 0);
acceleration = createVector(0, 0);
gravity = createVector(0, 0.5 * mass);
wind = createVector(0, 0);
textSize(18);
}
function draw() {
background(255);
if (!serialActive) {
background("#2d661b");
fill("white");
text("Press F to select Serial Port", 20, 30, width - 30, 200);
} else {
applyForce(wind);
applyForce(gravity);
velocity.add(acceleration);
velocity.mult(drag);
position.add(velocity);
acceleration.mult(0);
if (hasBounced) {
fill("red");
} else {
fill("white");
}
ellipse(position.x, position.y, mass, mass);
if (position.y > height - mass / 2) {
velocity.y *= -0.9; // dampening
position.y = height - mass / 2;
if (!hasBounced && abs(velocity.y) > 1) {
hasBounced = true;
setTimeout(() => (hasBounced = false), 100);
}
}
}
}
function applyForce(force) {
let f = p5.Vector.div(force, mass);
acceleration.add(f);
}
function keyPressed() {
if (key == " ") {
mass = 20;
position.set(width / 2, -mass);
velocity.mult(0);
gravity.y = 0.5 * mass;
wind.mult(0);
} else if (key == "f") {
setUpSerial();
}
}
function readSerial(data) {
if (data != null) {
let value = int(trim(data));
wind.x = map(value, 0, 1023, -1, 1);
let bounceState = 0;
if (hasBounced) {
bounceState = 1;
}
writeSerial(bounceState + "\n");
}
}
let position, velocity, acceleration, gravity, wind;
let drag = 0.99,
mass = 50,
hasBounced = false;
function setup() {
createCanvas(640, 360);
noFill();
position = createVector(width / 2, 0);
velocity = createVector(0, 0);
acceleration = createVector(0, 0);
gravity = createVector(0, 0.5 * mass);
wind = createVector(0, 0);
textSize(18);
}
function draw() {
background(255);
if (!serialActive) {
background("#2d661b");
fill("white");
text("Press F to select Serial Port", 20, 30, width - 30, 200);
} else {
applyForce(wind);
applyForce(gravity);
velocity.add(acceleration);
velocity.mult(drag);
position.add(velocity);
acceleration.mult(0);
if (hasBounced) {
fill("red");
} else {
fill("white");
}
ellipse(position.x, position.y, mass, mass);
if (position.y > height - mass / 2) {
velocity.y *= -0.9; // dampening
position.y = height - mass / 2;
if (!hasBounced && abs(velocity.y) > 1) {
hasBounced = true;
setTimeout(() => (hasBounced = false), 100);
}
}
}
}
function applyForce(force) {
let f = p5.Vector.div(force, mass);
acceleration.add(f);
}
function keyPressed() {
if (key == " ") {
mass = 20;
position.set(width / 2, -mass);
velocity.mult(0);
gravity.y = 0.5 * mass;
wind.mult(0);
} else if (key == "f") {
setUpSerial();
}
}
function readSerial(data) {
if (data != null) {
let value = int(trim(data));
wind.x = map(value, 0, 1023, -1, 1);
let bounceState = 0;
if (hasBounced) {
bounceState = 1;
}
writeSerial(bounceState + "\n");
}
}
Arduino Code
pinMode(lightPin, INPUT);
while (Serial.available() <= 0) {
while (Serial.available()) {
int ledState = Serial.parseInt();
if (Serial.read() == '\n') {
digitalWrite(ledPin, ledState);
Serial.println(analogRead(lightPin));
const int ledPin = 5;
const int lightPin = A0;
void setup() {
Serial.begin(9600);
pinMode(ledPin, OUTPUT);
pinMode(lightPin, INPUT);
while (Serial.available() <= 0) {
Serial.println("0");
; }
}
void loop() {
while (Serial.available()) {
int ledState = Serial.parseInt();
if (Serial.read() == '\n') {
digitalWrite(ledPin, ledState);
Serial.println(analogRead(lightPin));
}
}
}
const int ledPin = 5;
const int lightPin = A0;
void setup() {
Serial.begin(9600);
pinMode(ledPin, OUTPUT);
pinMode(lightPin, INPUT);
while (Serial.available() <= 0) {
Serial.println("0");
; }
}
void loop() {
while (Serial.available()) {
int ledState = Serial.parseInt();
if (Serial.read() == '\n') {
digitalWrite(ledPin, ledState);
Serial.println(analogRead(lightPin));
}
}
}
IMG_9260