Tinh and I going into this were slightly stuck on the creative idea first. However, during a brainstorm session, we came across a video of a ballerina music box – inspiring us to create something similar to this whilst sticking to the ‘instrument’ concept of the assignments. As we did not have a mini ballerina, I had a mini figurine nonetheless – a small penguin rubber (Basil Jr). Now that we had a figurine, we had to figure out how to implement the ‘instrument’ concept into this, as we did not want to make it into a passive music box.
Therefore, we decided we wanted to control the movement of the penguin with the instrument. We ended up deciding that buttons could be used as the method to control movement — we decided that each button would correspond to a note, and each note has a specific rotation. Originally, we wanted to use 8 buttons to correspond to the 8 notes, however, due to the limited space on our breadboard, we chose to stick with three – C,D,E.
This is our code:
const int photoPin = A0; // Photoresistor analog pin
const int ledPin = 6; // LED pin
const int buttonPins[] = {2, 3, 4}; // Button pins for sound triggers
const int photoThreshold = 100; // Threshold for photoresistor reading
const int speakerPin = 8;
Servo penguinServo; // Create servo object
const int servoPin = 9; // Servo pin
// Initialize the photoresistor, LED, and buttons
for (int i = 0; i < 3; i++) {
pinMode(buttonPins[i], INPUT_PULLUP); // Using internal pull-up resistor
penguinServo.attach(servoPin);
Serial.begin(9600); // For debugging if needed
// Read the photoresistor value
int photoValue = analogRead(photoPin);
Serial.println(photoValue);
// Control the LED based on photoresistor value
if (photoValue < photoThreshold) {
digitalWrite(ledPin, HIGH); // Turn on LED if it's dark
digitalWrite(ledPin, LOW); // Turn off LED otherwise
for (int i = 0; i < 3; i++) {
if (digitalRead(buttonPins[i]) == LOW) { // Button pressed
movePenguin(i); // Move penguin based on button pressed
// Function to move the penguin based on the button pressed
void movePenguin(int buttonIndex) {
penguinServo.write(0); // Move penguin in one direction
penguinServo.write(90); // Move penguin to center
penguinServo.write(180); // Move penguin in the other direction
delay(1000); // Hold position for 1 second
penguinServo.write(90); // Return to center
void playPitch(int buttonIndex) {
tone(speakerPin, NOTE_C4, 300); // Play note C4
tone(speakerPin, NOTE_D4, 300); // Play note D4
tone(speakerPin, NOTE_E4, 300); // Play note E4
#include <Servo.h>
#include "pitches.h"
const int photoPin = A0; // Photoresistor analog pin
const int ledPin = 6; // LED pin
const int buttonPins[] = {2, 3, 4}; // Button pins for sound triggers
const int photoThreshold = 100; // Threshold for photoresistor reading
const int speakerPin = 8;
Servo penguinServo; // Create servo object
const int servoPin = 9; // Servo pin
void setup() {
// Initialize the photoresistor, LED, and buttons
pinMode(ledPin, OUTPUT);
for (int i = 0; i < 3; i++) {
pinMode(buttonPins[i], INPUT_PULLUP); // Using internal pull-up resistor
}
// Attach servo to pin
penguinServo.attach(servoPin);
Serial.begin(9600); // For debugging if needed
}
void loop() {
// Read the photoresistor value
int photoValue = analogRead(photoPin);
Serial.println(photoValue);
// Control the LED based on photoresistor value
if (photoValue < photoThreshold) {
digitalWrite(ledPin, HIGH); // Turn on LED if it's dark
} else {
digitalWrite(ledPin, LOW); // Turn off LED otherwise
}
// Check each button
for (int i = 0; i < 3; i++) {
if (digitalRead(buttonPins[i]) == LOW) { // Button pressed
playPitch(i);
movePenguin(i); // Move penguin based on button pressed
}
}
}
// Function to move the penguin based on the button pressed
void movePenguin(int buttonIndex) {
switch (buttonIndex) {
case 0:
penguinServo.write(0); // Move penguin in one direction
break;
case 1:
penguinServo.write(90); // Move penguin to center
break;
case 2:
penguinServo.write(180); // Move penguin in the other direction
break;
}
delay(1000); // Hold position for 1 second
penguinServo.write(90); // Return to center
}
void playPitch(int buttonIndex) {
switch (buttonIndex) {
case 0:
tone(speakerPin, NOTE_C4, 300); // Play note C4
break;
case 1:
tone(speakerPin, NOTE_D4, 300); // Play note D4
break;
case 2:
tone(speakerPin, NOTE_E4, 300); // Play note E4
break;
}
delay(300);
noTone(speakerPin);
}
#include <Servo.h>
#include "pitches.h"
const int photoPin = A0; // Photoresistor analog pin
const int ledPin = 6; // LED pin
const int buttonPins[] = {2, 3, 4}; // Button pins for sound triggers
const int photoThreshold = 100; // Threshold for photoresistor reading
const int speakerPin = 8;
Servo penguinServo; // Create servo object
const int servoPin = 9; // Servo pin
void setup() {
// Initialize the photoresistor, LED, and buttons
pinMode(ledPin, OUTPUT);
for (int i = 0; i < 3; i++) {
pinMode(buttonPins[i], INPUT_PULLUP); // Using internal pull-up resistor
}
// Attach servo to pin
penguinServo.attach(servoPin);
Serial.begin(9600); // For debugging if needed
}
void loop() {
// Read the photoresistor value
int photoValue = analogRead(photoPin);
Serial.println(photoValue);
// Control the LED based on photoresistor value
if (photoValue < photoThreshold) {
digitalWrite(ledPin, HIGH); // Turn on LED if it's dark
} else {
digitalWrite(ledPin, LOW); // Turn off LED otherwise
}
// Check each button
for (int i = 0; i < 3; i++) {
if (digitalRead(buttonPins[i]) == LOW) { // Button pressed
playPitch(i);
movePenguin(i); // Move penguin based on button pressed
}
}
}
// Function to move the penguin based on the button pressed
void movePenguin(int buttonIndex) {
switch (buttonIndex) {
case 0:
penguinServo.write(0); // Move penguin in one direction
break;
case 1:
penguinServo.write(90); // Move penguin to center
break;
case 2:
penguinServo.write(180); // Move penguin in the other direction
break;
}
delay(1000); // Hold position for 1 second
penguinServo.write(90); // Return to center
}
void playPitch(int buttonIndex) {
switch (buttonIndex) {
case 0:
tone(speakerPin, NOTE_C4, 300); // Play note C4
break;
case 1:
tone(speakerPin, NOTE_D4, 300); // Play note D4
break;
case 2:
tone(speakerPin, NOTE_E4, 300); // Play note E4
break;
}
delay(300);
noTone(speakerPin);
}
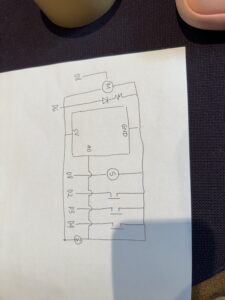