For this assignment I was inspired by Sol Lewitt’s work called Wall Drawings. I wanted to create an algorithm that connects multiple points together with lines similar to the artist’s work which asked people to draw points and join them together with straight lines.
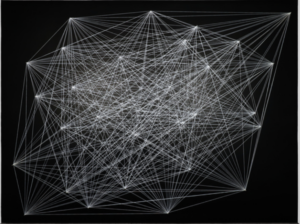
I started off with figuring out how to put random points on the canvas but using classes and arrays. Then, I proceeded on to figure out how to connect them and get rid of the original points. And then I used some code that we saw in class and modified it a little according to my needs to make the points move. I also used the background opacity feature to create a mesmerizing artwork that become more and more hypnotic the more you click on it.
Sketch:
Problems and Potential Future work:
I want to create a further element of interactivity where the points (or lines) move away from the user’s mouse as it is hovered over the canvas. I tried doing it, but ran into confusion due to multiple things going on with the classes and arrays already.
let fiftyballs = []; //array for storing however many balls the user wants to create
let xPosition = []; //arrays for x and y position of each point the line begins from
let x_Pos; //global variables to make it easy to store the x and y positions of the points
let totalcount = 5; // variable for number of points to be created
this.xPos = random(10, width - 10);
this.yPos = random(10, height - 10);
this.xSpeed = random(-3,3);
this.ySpeed = random(-3,3);
this.xPos += this.xSpeed;
this.yPos += this.ySpeed;
// check first for left and right wall
if (this.xPos <= 15 || this.xPos >= width - 15) {
this.xSpeed = -this.xSpeed;
// do the same for the ceiling and the floor
if (this.yPos <= 15 || this.yPos >= height - 15) {
this.ySpeed = -this.ySpeed;
circle(this.xPos, this.yPos, 0);
for (let i = 0; i < totalcount; i++) {
fiftyballs[i] = new ballproducer();
background(R, G , B ,15);
for (let i = 0; i < totalcount; i++) {
fiftyballs[i].checkForCollisions();
line(xPosition[i], yPosition [i], xPosition[i-1], yPosition[i-1]); //draws the line from the previous to the next point
function mouseClicked(){ //each mouse click adds +1 lines to the graphic
fiftyballs[totalcount] = new ballproducer(); // adds a new value to the array which has the objects for the balls
totalcount = totalcount+1; //increases the number of points or lines by 1 on each click
let fiftyballs = []; //array for storing however many balls the user wants to create
let xPosition = []; //arrays for x and y position of each point the line begins from
let yPosition = [];
let x_Pos; //global variables to make it easy to store the x and y positions of the points
let y_Pos;
let R = 236;
let G = 238;
let B = 129;
let totalcount = 5; // variable for number of points to be created
class ballproducer {
constructor() {
this.xPos = random(10, width - 10);
this.yPos = random(10, height - 10);
x_Pos = this.xPos;
y_Pos = this.yPos;
this.xSpeed = random(-3,3);
this.ySpeed = random(-3,3);
}
move() {
// move the ball
this.xPos += this.xSpeed;
this.yPos += this.ySpeed;
x_Pos = this.xPos;
y_Pos = this.yPos;
}
checkForCollisions() {
// check first for left and right wall
if (this.xPos <= 15 || this.xPos >= width - 15) {
this.xSpeed = -this.xSpeed;
}
// do the same for the ceiling and the floor
if (this.yPos <= 15 || this.yPos >= height - 15) {
this.ySpeed = -this.ySpeed;
}
}
draw() {
circle(this.xPos, this.yPos, 0);
}
}
function setup() {
createCanvas(600, 600);
frameRate (60);
for (let i = 0; i < totalcount; i++) {
fiftyballs[i] = new ballproducer();
}
}
function draw() {
background(R, G , B ,15);
for (let i = 0; i < totalcount; i++) {
xPosition[i] = x_Pos;
yPosition[i] = y_Pos;
fiftyballs[i].draw();
fiftyballs[i].move();
fiftyballs[i].checkForCollisions();
line(xPosition[i], yPosition [i], xPosition[i-1], yPosition[i-1]); //draws the line from the previous to the next point
}
}
function mouseClicked(){ //each mouse click adds +1 lines to the graphic
R = random(140,237);
G = random(160,238);
B = random(129,237);
fiftyballs[totalcount] = new ballproducer(); // adds a new value to the array which has the objects for the balls
totalcount = totalcount+1; //increases the number of points or lines by 1 on each click
}
let fiftyballs = []; //array for storing however many balls the user wants to create
let xPosition = []; //arrays for x and y position of each point the line begins from
let yPosition = [];
let x_Pos; //global variables to make it easy to store the x and y positions of the points
let y_Pos;
let R = 236;
let G = 238;
let B = 129;
let totalcount = 5; // variable for number of points to be created
class ballproducer {
constructor() {
this.xPos = random(10, width - 10);
this.yPos = random(10, height - 10);
x_Pos = this.xPos;
y_Pos = this.yPos;
this.xSpeed = random(-3,3);
this.ySpeed = random(-3,3);
}
move() {
// move the ball
this.xPos += this.xSpeed;
this.yPos += this.ySpeed;
x_Pos = this.xPos;
y_Pos = this.yPos;
}
checkForCollisions() {
// check first for left and right wall
if (this.xPos <= 15 || this.xPos >= width - 15) {
this.xSpeed = -this.xSpeed;
}
// do the same for the ceiling and the floor
if (this.yPos <= 15 || this.yPos >= height - 15) {
this.ySpeed = -this.ySpeed;
}
}
draw() {
circle(this.xPos, this.yPos, 0);
}
}
function setup() {
createCanvas(600, 600);
frameRate (60);
for (let i = 0; i < totalcount; i++) {
fiftyballs[i] = new ballproducer();
}
}
function draw() {
background(R, G , B ,15);
for (let i = 0; i < totalcount; i++) {
xPosition[i] = x_Pos;
yPosition[i] = y_Pos;
fiftyballs[i].draw();
fiftyballs[i].move();
fiftyballs[i].checkForCollisions();
line(xPosition[i], yPosition [i], xPosition[i-1], yPosition[i-1]); //draws the line from the previous to the next point
}
}
function mouseClicked(){ //each mouse click adds +1 lines to the graphic
R = random(140,237);
G = random(160,238);
B = random(129,237);
fiftyballs[totalcount] = new ballproducer(); // adds a new value to the array which has the objects for the balls
totalcount = totalcount+1; //increases the number of points or lines by 1 on each click
}