Concept
For this project, we decided on creating a creative musical instrument using a distance sensor. The device calculates the distance of an object (in this case, the object behaves as a percussion beater), and based on its location, tunes of varying frequencies are produced. The device allows the user to set the base note using a pushdown switch; once the switch is pressed, the distance calculated at that phase is used as an initial point. Thus, the user can move the beater to and fro in order to create a musical track. Similarly, using the potentiometer, the user can further control the duration and type of sound produced by the buzzer.
The devices used in the system are:
-
- One ultrasonic distance sensor
- One potentiometer
- One piezo buzzer
- One pushdown switch
- One 10 000 ohm resistor
The design of the instrument is based on the schematics as shown below:
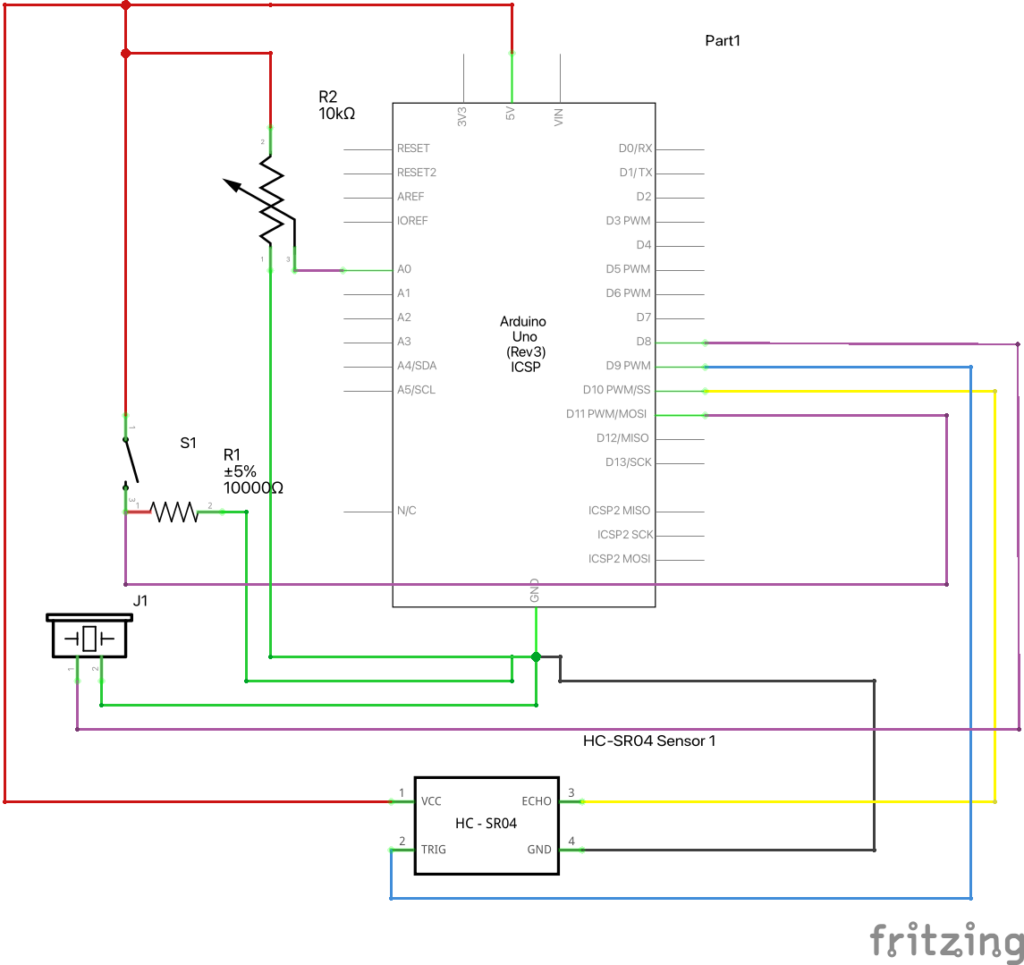
Codes
Our code mainly consists of 4 parts: ultrasonic sensor reading, button reading, potentiometer reading, and piezo buzzer output. We first started with ultrasonic sensors, referring to online code for simple distance measurement (Reference). We have slightly modified the original code to our needs.
int findDistance()
{
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
// Sets the trigPin on HIGH state for 10 micro seconds
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
// Reads the echoPin, returns the sound wave travel time in microseconds
long duration = pulseIn(echoPin, HIGH);
return (duration * 0.034 / 2);
}
This code above is a function that we used to measure distance using the ultrasonic sensor for each loop. Depending on the position of the object, the buzzer will produce different pitches of sound.
Then, the button comes in. Button is used to offset the starting position of the instrument. By placing an object in front of the sensor and pushing the button, the object’s position will automatically be set as a C4 note. Using this button, a player can adjust the instrument in a way that he or she is most comfortable with.
if (millis() - currentTime > 200 || currentTime == 0)
{
// If the switch is pressed, reset the offset
if (digitalRead(11) == 1)
{
distanceOffset = findDistance();
Serial.print("new offset: ");
Serial.println(distanceOffset);
currentTime = millis();
}
}
int distance = findDistance() - distanceOffset;
As shown above, when the button is pressed, the program will subtract the offset from the sensor reading.
int pitch_ = intoNotes(map(distance, 0, 20, 1, 7));
int pitch = pitch_ * myList[0];
int toneDuration = myList[1];
if(distance <= 20)
{
tone(pPin, pitch,toneDuration); // Controls 1. PinNumber, 2. Frequency, 3. Time Duration
}
else
{
noTone(pPin);
}
float intoNotes(int x)
{
switch(x)
{
case 1:
return 261.63;
case 2:
return 293.66;
case 3:
return 329.63;
case 4:
return 349.23;
case 5:
return 392.00;
case 6:
return 440.00;
case 7:
return 493.88;
default:
return 0;
}
}
The code above is how a program produces different pitches depending on the distance. The program maps 20 cm into 7 different integers; depending on the value of the mapped value, intoNotes() will return a specific pitch value accordingly. If the distance is above 20cm, the instrument will simply not produce a sound.
The code consists of two more functionalities which is facilitated by the function titled “noteModifier()”. It is a void function that takes the data read from the potentiometer as an argument.
void noteModifier(int volt)
{
float val1 = 0;
if (volt > 4 && volt <= 4.6)
val1 = 5;
else if (volt > 3 && volt <= 4)
val1 = 4;
else if (volt > 2 && volt <= 3)
val1 = 3;
else if (volt > 1 && volt <= 2)
val1 = 2;
else
val1 = 1;
myList[0] = val1;
myList[1] = val1 * 1000;
}
As a potentiometer is an analog sensor, it is connected to the A0 pin for a contiguous set of data that ranges from 0 to 1023. Before using this data as input, the values are mapped to a new scale of (1.0 to 5.0) V as the maximum emf in the circuit is 5V. The conversion is done by these lines of code at the beginning of the loop() function.
pVoltRead = analogRead(potentioPin);
trueVolt = (pVoltRead * 5.0/1023);
Serial.println(trueVolt);
Once the true volt in the potentiometer is calculated, the function noteModifier() uses a set of if-conditions to determine the value of a variable named ‘val1’. As seen in the code, the value of ‘val1’ varies based on the range of the true volt argument. The true purpose of using val1 is to alter the content of a global list “myList[]” declared at the beginning of the project. The list consists of two elements: (1) the first element is a coefficient that scales the frequency of the sound produced by the buzzer and (2) the second element is the duration of a single tone produced by the buzzer.
This way, the function determines two primary arguments — frequency and time duration — of the Arduino’s inbuilt function tone().
The idea here is to replicate the real-life application of the project. A user can rotate the knob in the potentiometer; consequently, different tones are produced for varying lengths of time. In short, including a potentiometer provides one additional layer of user interaction to the system. The second demo clip shows the functionality of the potentiometer and how it can be used to produce different tunes.
Reflection / Future Improvements
Working on this assignment was very entertaining and exciting. We were able to use different sensors in the kit and use them to create an object that we can physically interact with, unlike p5js. We believe the instrument came out as we initially expected, but there are a few points that we can improve upon:
-
-
- Ultrasonic Sensor
- Ultrasonic sensor uses sound waves to measure the distance. This means the measurement can be inaccurate and unreliable depending on the surface of the object the wave is hitting. Due to this, we found out our instrument malfunctions when trying to play it with our own hands. To improve this, we would like to use a laser distance sensor that is less affected by the object’s surface.
- Piezo Buzzer
- Piezo Buzzer is very simple to use, but its sound quality is great. If we use a better speaker, we may be able to add more interesting functionalities, such as producing different sounds of instruments when a button is pressed.
Watch demos of the instrument here:
Without potentiometer:
With potentiometer: